用python写一个网页爬虫
时间: 2024-05-19 15:10:22 浏览: 141
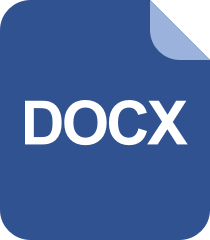
基于Python和Scrapy框架的网页爬虫设计与实现.docx
好的,下面是使用Python编写一个网页爬虫的基本步骤:
1. 安装必要的库
在Python中,有很多用于网页爬取的第三方库,例如requests、beautifulsoup4、lxml等等。您需要先安装这些库,以便使用它们提供的功能。您可以使用pip命令来安装这些库,例如:
```
pip install requests
pip install beautifulsoup4
pip install lxml
```
2. 发送HTTP请求
使用requests库向目标网站发送HTTP请求,获取网页源代码。例如,您可以使用如下代码获取百度首页的源代码:
```
import requests
url = 'http://www.baidu.com'
response = requests.get(url)
print(response.text)
```
3. 解析HTML代码
使用beautifulsoup4和lxml库解析网页源代码,以便提取所需的信息。您可以使用这些库提供的函数来查找特定的HTML标签、属性和文本内容。例如,以下代码可以实现从百度首页中提取所有的超链接:
```
from bs4 import BeautifulSoup
import requests
url = 'http://www.baidu.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
```
4. 存储数据
将爬取得到的数据存储到本地或者数据库中。例如,您可以使用如下代码将提取到的超链接保存到一个文本文件中:
```
from bs4 import BeautifulSoup
import requests
url = 'http://www.baidu.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
with open('links.txt', 'w', encoding='utf-8') as f:
for link in links:
f.write(link.get('href') + '\n')
```
以上就是使用Python编写一个简单的网页爬虫的基本步骤。当然,实际的爬虫程序可能会更加复杂,需要根据具体的需求进行调整和优化。
阅读全文
相关推荐
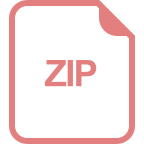
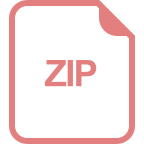
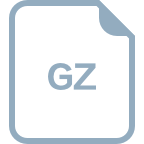
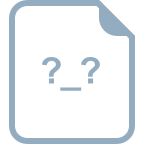
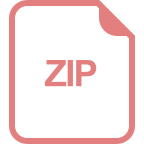
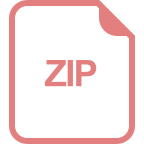
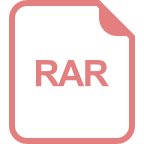
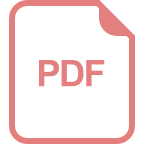
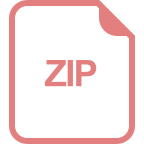
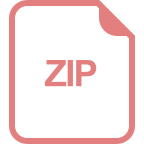

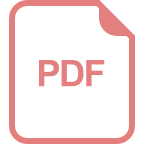
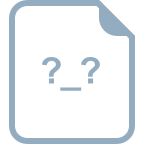
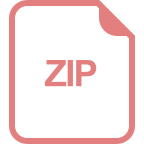
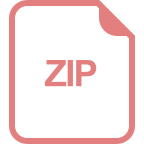