用vue3+ts+echarts实现一个双y轴折线图
时间: 2023-10-31 08:17:10 浏览: 50
首先,你需要安装 Echarts 和 Vue3,可以通过以下命令进行安装:
```
npm install echarts vue@next
```
然后,在你的 Vue3 项目中,创建一个组件,例如 `DoubleLineChart.vue`,并在模板中添加一个 div 元素,用于渲染 Echarts 图表:
```html
<template>
<div ref="chart" style="height: 500px"></div>
</template>
```
在组件中,你需要引入 Echarts 和定义图表的配置选项:
```typescript
import { defineComponent } from 'vue';
import * as echarts from 'echarts';
export default defineComponent({
name: 'DoubleLineChart',
props: {
data: {
type: Object,
required: true,
},
},
mounted() {
const chart = echarts.init(this.$refs.chart as HTMLDivElement);
const option = {
// 配置选项
};
chart.setOption(option);
},
});
```
在配置选项中,你需要定义两个 y 轴,分别对应两条折线:
```typescript
const option = {
tooltip: {
trigger: 'axis',
},
legend: {
data: ['销量', '访问量'],
},
grid: {
left: '3%',
right: '4%',
bottom: '3%',
containLabel: true,
},
xAxis: {
type: 'category',
boundaryGap: false,
data: this.data.xData,
},
yAxis: [
{
type: 'value',
name: '销量',
axisLabel: {
formatter: '{value} 件',
},
},
{
type: 'value',
name: '访问量',
axisLabel: {
formatter: '{value} 次',
},
},
],
series: [
{
name: '销量',
type: 'line',
yAxisIndex: 0,
data: this.data.y1Data,
},
{
name: '访问量',
type: 'line',
yAxisIndex: 1,
data: this.data.y2Data,
},
],
};
```
其中,`data` 属性是一个对象,包含了 x 轴和两个 y 轴的数据:
```typescript
export default defineComponent({
name: 'DoubleLineChart',
props: {
data: {
type: Object,
required: true,
},
},
mounted() {
const chart = echarts.init(this.$refs.chart as HTMLDivElement);
const option = {
tooltip: {
trigger: 'axis',
},
legend: {
data: ['销量', '访问量'],
},
grid: {
left: '3%',
right: '4%',
bottom: '3%',
containLabel: true,
},
xAxis: {
type: 'category',
boundaryGap: false,
data: this.data.xData,
},
yAxis: [
{
type: 'value',
name: '销量',
axisLabel: {
formatter: '{value} 件',
},
},
{
type: 'value',
name: '访问量',
axisLabel: {
formatter: '{value} 次',
},
},
],
series: [
{
name: '销量',
type: 'line',
yAxisIndex: 0,
data: this.data.y1Data,
},
{
name: '访问量',
type: 'line',
yAxisIndex: 1,
data: this.data.y2Data,
},
],
};
chart.setOption(option);
},
});
```
最后,在父组件中,你可以使用 `DoubleLineChart` 组件,并传递数据给它:
```html
<template>
<div>
<double-line-chart :data="chartData" />
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import DoubleLineChart from '@/components/DoubleLineChart.vue';
export default defineComponent({
name: 'App',
components: {
DoubleLineChart,
},
data() {
return {
chartData: {
xData: ['周一', '周二', '周三', '周四', '周五', '周六', '周日'],
y1Data: [120, 200, 150, 80, 70, 110, 130],
y2Data: [220, 180, 290, 130, 220, 200, 150],
},
};
},
});
</script>
```
相关推荐
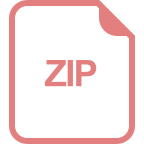














