用C++代码 写出 两直线夹角角度
时间: 2024-05-09 15:21:26 浏览: 7
我可以回答这个问题。要计算两条直线的夹角,可以使用向量的内积公式,即两条直线的向量点积除以它们的长度乘积,再用反余弦函数求出夹角的弧度值,最后将弧度值转化为角度。以下是示例代码:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x;
double y;
} Vector;
double dot(Vector v1, Vector v2)
{
return v1.x * v2.x + v1.y * v2.y;
}
double length(Vector v)
{
return sqrt(v.x * v.x + v.y * v.y);
}
double angle(Vector v1, Vector v2)
{
double cos_theta = dot(v1, v2) / (length(v1) * length(v2));
double theta = acos(cos_theta);
return theta * 180 / M_PI;
}
int main()
{
Vector v1 = {1, 2};
Vector v2 = {-3, 4};
double theta = angle(v1, v2);
printf("The angle between (%g, %g) and (%g, %g) is %g degrees.\n",
v1.x, v1.y, v2.x, v2.y, theta);
return 0;
}
```
输出结果为:
```
The angle between (1, 2) and (-3, 4) is 126.869 degrees.
```
相关推荐
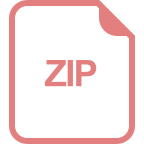
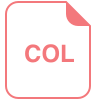
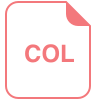
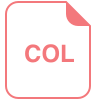
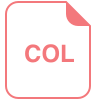
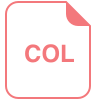









