在MATLAB中设计遗传算法时,如何编写代码以实现种群的适应度评估、选择、交叉和变异等关键步骤?请提供代码示例。
时间: 2024-11-11 10:42:38 浏览: 57
在MATLAB中实现遗传算法,编写适应度评估、选择、交叉和变异等关键步骤对于优化问题的解决至关重要。现在,我们将深入探讨如何在MATLAB环境中进行这些操作,并提供代码示例以供参考。
参考资源链接:[遗传算法MATLAB实现解析](https://wenku.csdn.net/doc/2nsr0jwipx?spm=1055.2569.3001.10343)
首先,适应度评估是遗传算法中至关重要的一个环节,它决定了每个个体在选择过程中的生存概率。在MATLAB中,你可以定义一个适应度函数,例如:
```matlab
function fit = fitnessFunction(chromosome)
% 适应度函数示例:最大化某个目标函数值
fit = -sum(chromosome.^2); % 示例目标函数为负的平方和
end
```
接下来是选择过程,选择操作用于从当前种群中选出优秀的个体,以便遗传到下一代。通常使用轮盘赌选择、锦标赛选择等方法。以下是一个简单的轮盘赌选择的示例:
```matlab
function selected = rouletteWheelSelection(population, fitness)
% 轮盘赌选择过程
totalFitness = sum(fitness);
probs = fitness / totalFitness;
cumProbs = cumsum(probs);
selected = zeros(size(population));
for i = 1:size(population, 1)
r = rand();
for j = 1:length(cumProbs)
if r <= cumProbs(j)
selected(i, :) = population(j, :);
break;
end
end
end
end
```
交叉(重组)是遗传算法中的另一个关键步骤,它通过组合父代个体的部分基因产生子代个体。以下是一个简单的单点交叉操作示例:
```matlab
function children = singlePointCrossover(parents)
% 单点交叉操作
children = parents;
crossPoints = randi([1, size(parents, 2)-1], size(parents, 1), 1);
for i = 1:size(parents, 1)
if rand() < 0.5
continue; % 保留50%的概率不进行交叉
end
point = crossPoints(i);
children(i, point+1:end) = parents(i, point+1:end);
children(i, 1:point) = parents(mod(i, size(parents, 1))+1, 1:point);
end
end
```
变异操作用于在种群中引入新的遗传多样性,防止算法早熟收敛。以下是一个简单的均匀变异操作示例:
```matlab
function mutated = uniformMutation(children, mutationRate, chromosomeLength)
% 均匀变异操作
mutated = children;
for i = 1:size(children, 1)
for j = 1:chromosomeLength
if rand() < mutationRate
mutated(i, j) = rand(); % 使用随机数替换基因值
end
end
end
end
```
以上代码片段展示了如何在MATLAB中实现遗传算法的关键步骤。实际应用中,你需要根据具体问题调整适应度函数,并合理设置遗传算法的参数,如种群大小、交叉率、变异率等。通过这些步骤的组合,你将能够在MATLAB中构建出强大的遗传算法框架,解决复杂的优化问题。
在开始编写代码之前,强烈建议阅读《遗传算法MATLAB实现解析》这份资源。它详细解释了遗传算法的基本原理和MATLAB实现的关键细节,可以帮助你更好地理解算法的每个组成部分及其相互作用,从而高效地构建出完整的遗传算法模型。
参考资源链接:[遗传算法MATLAB实现解析](https://wenku.csdn.net/doc/2nsr0jwipx?spm=1055.2569.3001.10343)
阅读全文
相关推荐




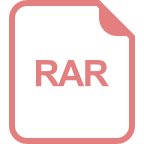









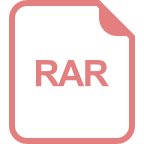


