java 实现C#的.any()方法
时间: 2024-04-13 10:31:18 浏览: 11
在Java中,可以使用Lambda表达式和Stream API来实现类似C#中的`.Any()`方法的功能。下面是一个示例代码:
```java
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
boolean anyMatch = numbers.stream().anyMatch(n -> n > 3);
System.out.println(anyMatch);
}
}
```
在上面的代码中,我们首先创建了一个包含整数的列表`numbers`。然后,我们使用`stream()`方法将列表转换为流,然后使用`anyMatch()`方法来检查流中的元素是否满足条件(这里的条件是元素大于3)。如果至少有一个元素满足条件,`anyMatch()`方法将返回`true`,否则返回`false`。
这个示例代码的输出将是`true`,因为列表中存在大于3的元素。你可以根据自己的需求修改Lambda表达式中的条件来实现不同的功能。
希望能帮到你!如果还有其他问题,请随时问我。
相关问题
java sockechannel 传输文件到c#
使用Java SocketChannel传输文件到C语言,可以通过以下步骤实现:
1. 在Java端,创建一个SocketChannel对象,并连接到C语言的服务器。可以使用SocketChannel.open()和SocketChannel.connect()方法来完成。
2. 打开文件并读取文件内容。可以使用FileInputStream来打开文件,并使用BufferedInputStream将文件内容读取到缓冲区中。
3. 创建一个ByteBuffer对象,将缓冲区中的文件内容写入到该ByteBuffer对象中。
4. 使用SocketChannel的write()方法将ByteBuffer中的内容发送到C语言的服务器。
5. 在C语言的服务器上,使用相应的socket库函数来接收数据。
6. 在C语言端,创建一个文件,并将接收到的数据写入到这个文件中。使用标准的文件操作函数,如fopen()和fwrite()来实现。
7. 关闭SocketChannel和文件,释放资源。
以下是一个简单的示例代码:
Java端代码:
```java
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
public class FileTransfer {
public static void main(String[] args) {
String filename = "path/to/file"; // 要传输的文件路径
try (SocketChannel socketChannel = SocketChannel.open()) {
socketChannel.connect(serverAddress); // 连接到C语言服务器
// 打开文件并读取文件内容
FileInputStream fileInputStream = new FileInputStream(filename);
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = bufferedInputStream.read(buffer)) != -1) {
// 创建ByteBuffer,并将文件内容写入到其中
ByteBuffer byteBuffer = ByteBuffer.wrap(buffer, 0, bytesRead);
// 将ByteBuffer中的内容发送到C语言服务器
socketChannel.write(byteBuffer);
}
System.out.println("文件传输完成");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
C语言服务器端代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#define PORT 8080
int main() {
int serverSocket, newSocket, bytesRead;
struct sockaddr_in address;
int addrlen = sizeof(address);
char buffer[1024] = {0};
// 创建服务器Socket
if ((serverSocket = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
// 绑定服务器Socket
if (bind(serverSocket, (struct sockaddr *)&address, sizeof(address))<0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
// 监听连接请求
if (listen(serverSocket, 1) < 0) {
perror("listen failed");
exit(EXIT_FAILURE);
}
printf("等待接收数据...\n");
// 接收连接请求
if ((newSocket = accept(serverSocket, (struct sockaddr *)&address, (socklen_t*)&addrlen))<0) {
perror("accept failed");
exit(EXIT_FAILURE);
}
FILE* file = fopen("received_file.txt", "wb");
if (file == NULL) {
perror("file open failed");
exit(EXIT_FAILURE);
}
// 接收数据并写入文件
while ((bytesRead = read(newSocket, buffer, sizeof(buffer))) > 0) {
fwrite(buffer, 1, bytesRead, file);
}
printf("文件传输完成\n");
fclose(file);
close(newSocket);
close(serverSocket);
return 0;
}
```
以上是一个基本示例,真实的文件传输可能还需要添加错误处理、文件校验等逻辑,具体根据实际需求进行修改。
1.Explain how bytecode (either CIL or Java bytecode) differs from machine code with examples of instructions. 2.Why autoboxing and autounboxing is not needed in C#? 3.How to decide when and when not to use auto-implemented properties in C#? 4.What is the difference between structs and class in C#? 5.How to decide between using a regular parameter declaration and a ref parameter declaration in a C# method? 6.What is the difference between modifiers new and override in C#? 7.How to decide between using a two-dimensional array and an array of arrays in a programming situation in C#? 8.Discuss the common errors that can arise with switch statements in Java and how C# eliminates those errors automatically. 9.How to use foreach loop in C#? Give one example. 10.What does this program print on the screen? Explain your answer by describing what a delegate is, including the meaning of +=. class C { delegate bool Check (int number); static bool IsEven (int number) { return number % 2 == 0;} static bool IsSmallerThan3 (int number) { return number < 3;} static void Main () { Check f = IsEven; f += IsSmallerThan3; Console.WriteLine ( f(3)); Console.WriteLine ( f(2)); } }
1. Bytecode is an intermediate code that is generated by a compiler to be executed by a virtual machine. It is platform-independent and can be executed on any platform that has a virtual machine installed. Machine code, on the other hand, is the binary code that is directly executed by a computer's CPU. Here are some examples of instructions in bytecode and machine code:
- Bytecode (CIL): ldloc.0 (load local variable 0), ldc.i4.5 (load constant value 5), add (addition)
- Machine code: mov eax, [ebp-4] (move value from memory to register), mov ebx, 5 (move constant value to register), add eax, ebx (addition)
2. Autoboxing and autounboxing is not needed in C# because C# has a feature called "value types" that allows the value types to be treated like objects. This means that value types can be used in collections and passed as parameters to methods without the need for autoboxing and autounboxing.
3. Auto-implemented properties should be used when there is no additional logic required for the getter or setter methods. If additional logic is required, then a regular property with custom getter or setter methods should be used.
4. Structs and classes are similar in that they both can contain fields, methods, and properties. The main difference is that structs are value types, while classes are reference types. This means that structs are passed by value, while classes are passed by reference.
5. A regular parameter declaration should be used when the method only needs to read the value of the parameter. A ref parameter declaration should be used when the method needs to modify the value of the parameter.
6. The "new" modifier is used to hide a method or property in a derived class, while the "override" modifier is used to replace a method or property in a base class with a new implementation in a derived class.
7. A two-dimensional array should be used when the data is logically a matrix or a table with rows and columns. An array of arrays should be used when the data is logically a collection of arrays.
8. In Java, the switch statement can cause errors if there are duplicate case values or if the break statement is not used after each case. In C#, the switch statement automatically eliminates these errors by requiring a default case and not allowing fall-through cases.
9. The foreach loop is used to iterate over a collection of items, such as an array or a list. Here is an example:
```
int[] numbers = { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
```
10. The program will print "True" and "False" because the delegate "f" contains two methods, "IsEven" and "IsSmallerThan3", which are both called when "f" is invoked with a parameter. The "+=" operator is used to add the "IsSmallerThan3" method to the delegate "f". A delegate is a type that encapsulates a method or a group of methods, and the "+=" operator is used to add a method to a delegate's invocation list.
相关推荐
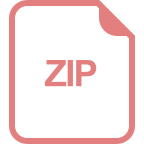
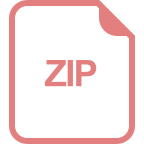








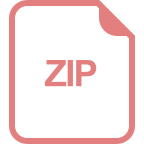
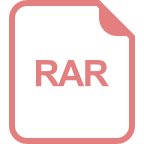
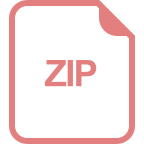
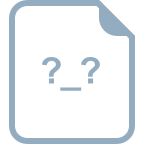
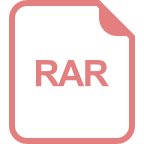