c++ 基于opencv4.6 实现的人脸对齐算法
时间: 2023-10-23 14:09:38 浏览: 166
人脸对齐算法是一种常见的人脸识别前置步骤,可以提高人脸识别的准确率。在 OpenCV 4.6 中,可以使用 dlib 库提供的人脸检测器和关键点检测器来实现人脸对齐。以下是 C++ 代码实现人脸对齐的示例:
```c++
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/image_processing.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing/shape_predictor.h>
using namespace cv;
using namespace dlib;
int main()
{
// 加载人脸检测器和关键点检测器
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor predictor;
deserialize("shape_predictor_68_face_landmarks.dat") >> predictor;
// 加载需要对齐的图像
Mat img = imread("test.jpg");
// 将 OpenCV 的 Mat 转换为 dlib 的图像类型
cv_image<bgr_pixel> dlib_img(img);
// 人脸检测和关键点检测
std::vector<rectangle> faces = detector(dlib_img);
full_object_detection shape = predictor(dlib_img, faces[0]);
// 计算人脸对齐矩阵
Point2f src_points[3];
Point2f dst_points[3];
src_points[0] = Point2f(shape.part(36).x(), shape.part(36).y());
src_points[1] = Point2f(shape.part(45).x(), shape.part(45).y());
src_points[2] = Point2f(shape.part(30).x(), shape.part(30).y());
dst_points[0] = Point2f(0.2f * img.cols, 0.2f * img.rows);
dst_points[1] = Point2f(0.8f * img.cols, 0.2f * img.rows);
dst_points[2] = Point2f(0.5f * img.cols, 0.8f * img.rows);
Mat warp_mat = getAffineTransform(src_points, dst_points);
// 对齐图像
Mat aligned_img;
warpAffine(img, aligned_img, warp_mat, img.size());
// 显示对齐前和对齐后的图像
imshow("Original Image", img);
imshow("Aligned Image", aligned_img);
waitKey(0);
return 0;
}
```
在本例中,我们首先加载了 dlib 提供的人脸检测器和关键点检测器。然后,将需要对齐的图像转换为 dlib 的图像类型,进行人脸检测和关键点检测。接着,根据检测到的关键点计算人脸对齐矩阵,并使用 `warpAffine` 函数对图像进行对齐。最后,将对齐前和对齐后的图像显示出来。需要注意的是,本例中使用了
阅读全文
相关推荐
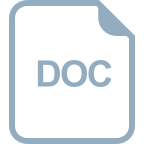
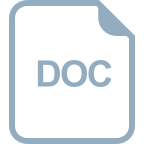
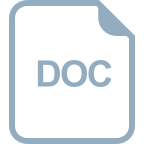
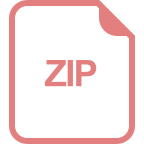
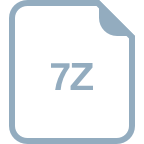
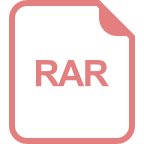
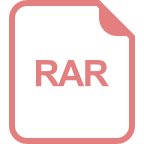
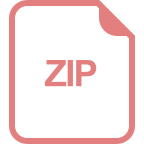
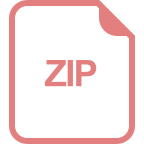
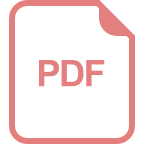
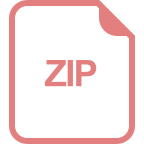
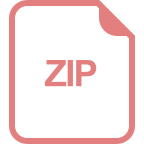
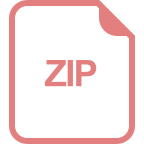
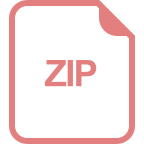
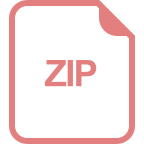
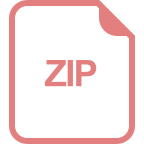
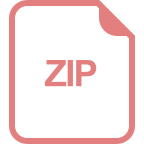
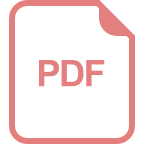
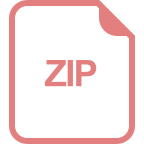