python assert 用法
时间: 2024-02-11 19:36:48 浏览: 60
Python中的assert语句用于检查条件是否为True,如果不是,就会抛出AssertionError异常。
assert语句的用法如下:
```python
assert condition, message
```
其中,condition是要检查的条件,message是可选的错误信息。如果condition为False,就会抛出AssertionError异常,并将message作为异常信息。
例如,下面的代码检查一个列表是否为空:
```python
my_list = [1, 2, 3]
assert len(my_list) == 0, "The list is not empty"
```
由于my_list不为空,所以条件不成立,assert语句会抛出AssertionError异常,异常信息为"The list is not empty"。
相关问题
python assert用法
Python中的assert语句用于检查一个条件是否为真,如果条件为假,则会引发一个AssertionError。语法格式如下:
```
assert 条件 [, 错误消息]
```
举个例子:
```
x = 5
assert x > 0, "x should be positive"
```
这里,如果x大于0,就不会发生任何事情,否则会抛出一个AssertionError,错误消息为"x should be positive"
注意: assert语句只在条件为假时才会引发错误,且在python解释器运行时默认是开启状态,可以通过-O参数关闭。
python assert语句
`assert`语句在Python中用于断言某个条件必须为真。如果条件为假,它会引发一个`AssertionError`异常,这通常用来验证程序内部的状态。基本语法如下:
```python
assert condition, message
```
- `condition`: 需要测试的布尔表达式,如果结果为`False`,则触发异常。
- `message` (可选): 当条件失败时,异常消息,可以提供更详细的错误信息。
下面是一些使用示例[^1][^2]:
1. **基本用法**:
```python
x = 5
assert x > 0, "x should be positive"
```
这里,如果`x`小于等于0,将会抛出异常并显示自定义的消息"x should be positive"。
2. **仅检查条件**:
```python
assert len(some_list) == expected_length, "List length does not match"
```
检查列表长度是否如预期。
阅读全文
相关推荐
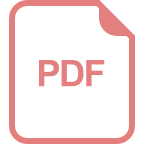
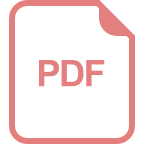






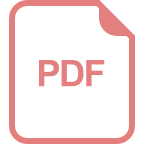
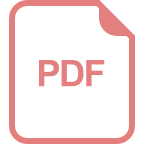
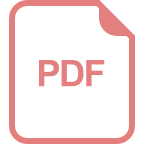
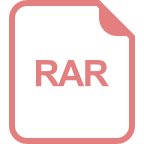