java返回二进制文件
时间: 2023-08-31 11:05:59 浏览: 37
在Java中,可以通过以下步骤返回二进制文件:
1. 使用 FileInputStream 类创建一个输入流对象,用于读取文件的原始字节数据。
2. 创建一个字节数组,用于存储读取的文件数据。
3. 使用输入流对象的 read() 方法将文件数据读取到字节数组中。
4. 关闭输入流对象。
5. 返回字节数组。
以下是一个示例代码:
```
import java.io.FileInputStream;
import java.io.IOException;
public class BinaryFileReader {
public static byte[] readFile(String filePath) throws IOException {
FileInputStream fis = new FileInputStream(filePath);
byte[] buffer = new byte[fis.available()];
fis.read(buffer);
fis.close();
return buffer;
}
}
```
在上面的示例中,readFile() 方法接收文件路径作为参数,然后返回一个字节数组,其中包含了文件的原始二进制数据。你可以根据需要将字节数组转换为其他格式的数据,例如字符串或图像等。
相关问题
java接口返回二进制文件
在 Java 中,可以通过接口返回二进制文件的方式是将二进制数据转换为字节数组,然后在接口方法中返回这个字节数组。以下是一个示例代码:
```
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/binary")
public class BinaryFileResource {
@GET
@Path("/download")
@Produces(MediaType.APPLICATION_OCTET_STREAM)
public Response downloadFile() {
try {
String filePath = "/path/to/file"; // 文件路径
FileInputStream fis = new FileInputStream(filePath);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
byte[] fileContent = bos.toByteArray();
return Response.ok(fileContent, MediaType.APPLICATION_OCTET_STREAM)
.header("Content-Disposition", "attachment; filename=\"file.bin\"")
.build();
} catch (IOException e) {
e.printStackTrace();
return Response.serverError().build();
}
}
}
```
在上面的示例中,我们使用 FileInputStream 读取文件数据,并将二进制数据写入 ByteArrayOutputStream 中。最后,我们通过 toByteArray() 方法将 ByteArrayOutputStream 中的数据转换为字节数组。然后,在 downloadFile() 方法中,我们使用 Response.ok() 方法返回一个 Response 对象,其中包含字节数组和相应的 MediaType。此外,我们还设置了 Content-Disposition 头,用于指定浏览器下载文件时的文件名。
java接口返回二进制文件 hutool
在 Java 中,可以使用 Hutool 工具类库来简化返回二进制文件的操作。Hutool 中的 IoUtil 类提供了 readBytes() 方法,该方法可以将文件内容读取为字节数组。我们可以将字节数组作为接口返回值,从而实现接口返回二进制文件的功能。以下是一个使用 Hutool 返回二进制文件的示例代码:
```
import cn.hutool.core.io.IoUtil;
import org.springframework.core.io.ClassPathResource;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
@RestController
public class BinaryFileController {
@GetMapping(value = "/file", produces = MediaType.APPLICATION_OCTET_STREAM_VALUE)
public ResponseEntity<byte[]> downloadFile() throws IOException {
ClassPathResource fileResource = new ClassPathResource("file.bin"); // 文件路径
byte[] fileContent = IoUtil.readBytes(fileResource.getInputStream()); // 读取文件内容为字节数组
return ResponseEntity.ok()
.header("Content-Disposition", "attachment; filename=\"file.bin\"")
.body(fileContent);
}
}
```
在上面的示例中,我们使用 IoUtil.readBytes() 方法读取文件内容为字节数组。然后,我们使用 ResponseEntity.ok() 方法返回一个 ResponseEntity 对象,其中包含字节数组和相应的 MediaType。此外,我们还设置了 Content-Disposition 头,用于指定浏览器下载文件时的文件名。
相关推荐
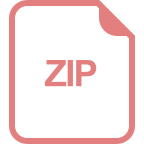
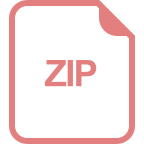
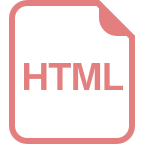












