ring road c++
时间: 2023-09-03 13:28:18 浏览: 152
### 回答1:
"Ring road" problem is a classic problem in computer science, which involves finding the shortest path that visits all the nodes in a graph. In the case of a ring road, the graph is a simple cycle, and the problem is to find the shortest Hamiltonian cycle.
Here is an example C++ code for solving this problem using the Brute-force approach:
```
#include<bits/stdc++.h>
using namespace std;
const int MAXN = 10;
int n, ans = INT_MAX;
int G[MAXN][MAXN];
void dfs(int cur, int cost, int vis) {
if(vis == (1 << n) - 1) { // all nodes have been visited
ans = min(ans, cost + G[cur][0]);
return;
}
for(int i = 0; i < n; i++) {
if(!(vis & (1 << i))) { // i-th node has not been visited
dfs(i, cost + G[cur][i], vis | (1 << i));
}
}
}
int main() {
cin >> n;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cin >> G[i][j];
}
}
dfs(0, 0, 1);
cout << ans << endl;
return 0;
}
```
The main idea of this code is to use depth-first search (DFS) to try all the possible paths, and find the shortest one. The `dfs` function takes three parameters: the current node `cur`, the cost so far `cost`, and a bit vector `vis` which keeps track of which nodes have been visited. The base case is when all nodes have been visited, we update the answer if the current path is shorter than the previous best. In the recursive case, we try all the unvisited nodes, and recursively call `dfs` with the updated parameters. Finally, we call `dfs` with the starting node `0`, cost `0`, and set the first bit of `vis` to `1` to represent that the first node has been visited.
### 回答2:
"Ring Road C"是一个指环路C的名称。指环路是一种环状的道路系统,通常围绕城市或地区。在很多大城市都可以找到不止一个环状道路,不同的环路可能有不同的名称,以便进行区分。
指环路C通常表示城市周围的第三个环路,有时也称为城市环线或外环。它通常位于离市中心较远的地区,连接主要的交通枢纽和郊区。指环路C的主要作用是分流穿越城市的交通流量,减少市区拥堵。
指环路C通常是一条较宽的高速公路,具有多个车道和出入口。它连接周边的主要城市、郊区以及通往市区的道路。该环路可以快速方便地将车辆从郊区引入市区,同时也可以将车辆从市区引出,以减轻市区的交通负担。
此外,指环路C也是城市规划的一部分。在城市建设时,规划者通常会考虑到城市未来的发展需求,并预留足够的空间来建设环路C。这样可以方便未来城市的扩张和道路网络的完善,并提供更好的交通条件。
总之,指环路C是城市周围的第三条环状道路,它在城市交通中扮演着重要的角色。通过分流交通流量和便捷地连接郊区和市区,指环路C能够减轻市区的交通压力,并提供更好的交通条件。
### 回答3:
ring road c 是指C环形路,也称为环城高速公路,它是一条环绕城市的高速公路。环城高速公路是一种重要的城市交通设施,通常用于连接主要城市区域,减少交通拥堵,提高交通效率。
C环形路的建设有许多好处。首先,C环形路可以分流城市内部的交通流量,减轻主要道路的压力,减少堵车现象。这样可以提高交通运输效率,节约人们的时间。其次,环形路可以缩短城市内部的距离,方便市民之间的交流和商业活动的发展,促进城市的经济繁荣。此外,环形路还可以为城市提供更好的交通服务,方便居民出行,提升城市形象和品质。
当然,建设环城高速公路也会面临一些挑战和问题。首先,环城高速公路的建设需要占用大量土地资源,可能会引起环境问题和土地利用冲突。其次,建设过程中可能会影响到周边居民的正常生活和工作。因此,在规划和建设环城高速公路时,需要考虑到相关方面的权益和环境保护问题,确保公共利益的最大化。
总的来说,C环形路的建设对城市发展和交通运输有着重要的意义。它是连接城市区域的重要交通枢纽,可以提高交通运输效率,促进经济发展,改善市民生活质量。然而,在建设过程中需要充分考虑到环保和社会效益,确保最大限度地实现公共利益。
阅读全文
相关推荐
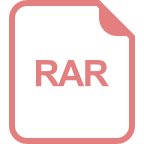
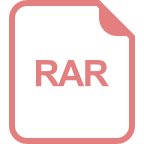
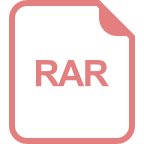
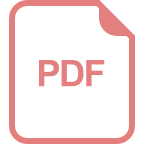
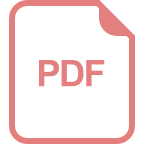
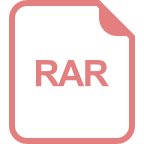
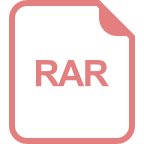
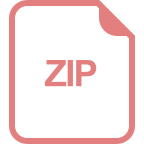
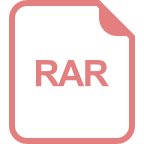
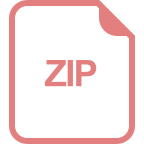
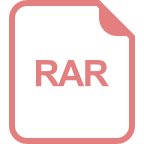
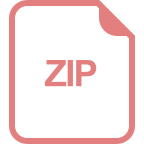
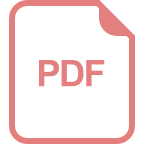
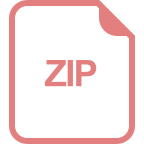
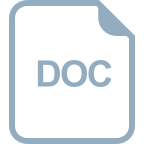
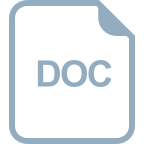