用C语言写从键盘输入一个以秒为单位的时间值整数,将其转换成时、分、秒的形式输出,输入格式,输入代表总秒数的整数,输出格式,输出时、分、秒的整数值,以英文冒号分隔,最后换行
时间: 2024-11-10 22:26:53 浏览: 20
In C language, you can accomplish this by following these steps:
```c
#include <stdio.h>
// Function to convert seconds to hours, minutes, and seconds
void convertSeconds(int total_seconds) {
int hours = total_seconds / 3600;
int minutes = (total_seconds % 3600) / 60;
int seconds = total_seconds % 60;
printf("%d:%02d:%02d\n", hours, minutes, seconds); // '02d' ensures single-digit minutes and seconds are padded with a leading zero
}
int main() {
int input_seconds;
printf("Enter the time in seconds: ");
scanf("%d", &input_seconds);
convertSeconds(input_seconds);
return 0;
}
```
Here's how it works:
1. Include the `stdio.h` header for standard input/output functions.
2. Define a function `convertSeconds` that takes an integer parameter `total_seconds`.
3. Inside `convertSeconds`, calculate hours by dividing `total_seconds` by 3600, minutes by taking the remainder of the division by 3600, and seconds by the modulus operator `%` on 60.
4. Use `printf` with the appropriate format specifier `%d` for each value, followed by a colon and the desired padding (`%02d` ensures two digits).
5. In `main()`, prompt the user for input using `scanf`, read into `input_seconds`, and call the `convertSeconds` function.
Example run:
```
Enter the time in seconds: 9780
2:46:20
```
阅读全文
相关推荐
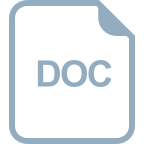
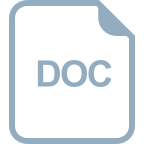
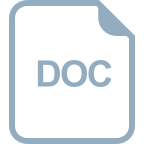















