mybatis 一对多查询时,子查询只有一条数据什么情况
时间: 2023-06-13 19:04:17 浏览: 78
在 MyBatis 中进行一对多查询时,如果子查询只返回一条数据,有以下几种情况:
1. 外键值重复:可能是由于外键值重复导致查询结果只返回了一条数据。此时需要检查外键值是否正确,是否存在重复。
2. 查询条件不正确:子查询可能存在查询条件,如果查询条件不正确,可能导致查询结果只返回了一条数据。此时需要检查查询条件是否正确,是否漏掉了某些条件。
3. 数据库设计问题:如果数据库表的设计存在问题,可能会导致一对多查询时只返回一条数据。此时需要检查数据库表的设计是否合理。
4. MyBatis 配置问题:可能是 MyBatis 的配置问题导致查询结果只返回了一条数据。此时需要检查 MyBatis 的配置文件是否正确,是否漏掉了某些配置信息。
相关问题
Mybatis中的进行一对多映射时使用什么完成
在 MyBatis 中进行一对多映射时,可以使用嵌套查询或嵌套结果映射来完成。下面是具体的实现方法:
1. 嵌套查询
嵌套查询是指在主查询中使用子查询来获取从表的数据,然后将这些数据与主表数据合并返回。这种方式需要在 SQL 语句中使用嵌套查询语句,例如:
```
<select id="selectUser" resultType="User">
select * from user where id=#{id};
select * from order where user_id=#{id};
</select>
```
这样就能同时查询出用户信息和该用户的所有订单信息。在使用时,可以将多条 SQL 语句封装到一个 Mapper 接口中,并在 XML 配置文件中进行配置。
2. 嵌套结果映射
嵌套结果映射是指在主查询的结果集中包含从表的数据,然后使用 MyBatis 的 resultMap 映射方式进行映射。这种方式需要在 XML 配置文件中定义 resultMap,并使用 collection 元素来定义从表的映射关系,例如:
```
<resultMap id="userMap" type="User">
<id property="id" column="id"/>
<result property="name" column="name"/>
<collection property="orders" ofType="Order">
<id property="id" column="order_id"/>
<result property="price" column="price"/>
</collection>
</resultMap>
```
这样就能同时查询出用户信息和该用户的所有订单信息,并将其映射到 User 对象中。在使用时,只需要在 SQL 语句中使用 JOIN 或 LEFT JOIN 连接主表和从表即可。
总之,MyBatis 中进行一对多映射可以使用嵌套查询或嵌套结果映射来完成,具体的实现方式需要根据具体的场景和需求来选择。
mybatis collection标签 一对多查询 怎么才可以只对主表进行分组 子表不允许收到影响 一条查询语句写出来
当使用 MyBatis 的 `collection` 标签进行一对多查询时,可以使用嵌套的 `select` 元素来指定子查询的 SQL 语句,从而实现对子表的查询操作。如果想要只对主表进行分组,而不影响子表的查询结果,可以在子查询中添加 `where` 子句来筛选出需要的数据。
下面是一个示例,其中查询语句包括了 `collection` 标签和嵌套的 `select` 元素,以及 `where` 子句来限制子表的查询条件:
```
<select id="selectOrders" resultMap="orderResult">
SELECT o.*, c.customer_name
FROM orders o
JOIN customers c ON o.customer_id = c.customer_id
GROUP BY o.order_id
</select>
<resultMap id="orderResult" type="Order">
<id column="order_id" property="orderId"/>
<result column="order_date" property="orderDate"/>
<result column="customer_name" property="customerName"/>
<collection property="orderDetails" ofType="OrderDetail">
<id column="detail_id" property="detailId"/>
<result column="product_id" property="productId"/>
<result column="quantity" property="quantity"/>
<result column="price" property="price"/>
<association property="product" javaType="Product">
<id column="product_id" property="productId"/>
<result column="product_name" property="productName"/>
<result column="unit_price" property="unitPrice"/>
</association>
<select id="selectOrderDetailsByOrderId" resultMap="orderDetailResult">
SELECT *
FROM order_details
WHERE order_id = #{orderId}
AND product_id IN (SELECT DISTINCT product_id FROM orders WHERE order_id = #{orderId})
</select>
</collection>
</resultMap>
```
在这个示例中,`selectOrders` 标签用来查询主表 orders 的数据,并使用 `group by` 子句对主表进行分组。`orderResult` 标签用来映射主表 orders 和子表 order_details 的数据,其中子表 order_details 的查询操作使用了 `select` 元素。具体来说:
- `orderResult` 标签的 `collection` 属性指定了主表 orders 和子表 order_details 之间的关系,其中 `property` 属性指定了 orders 对象中的属性名(即 orderDetails),`ofType` 属性指定了子表的 Java 类型。
- `orderResult` 标签中的 `id` 和 `result` 标签用来映射主表 orders 的数据。
- `orderResult` 标签中的 `collection` 标签用来映射子表 order_details 的数据,其中 `id` 和 `result` 标签用来映射子表 order_details 的字段,`association` 标签用来映射子表 order_details 和另一张表 products 的关系。
- `selectOrderDetailsByOrderId` 标签用来查询子表 order_details 的数据,其中 `where` 子句中的条件限制了查询结果只包括主表 orders 中出现过的产品,从而确保了子表不受主表分组的影响。
以上是一条完整的 MyBatis 查询语句,可以实现只对主表进行分组,而不影响子表的查询结果。
相关推荐
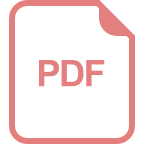
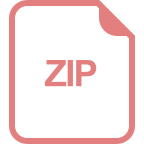
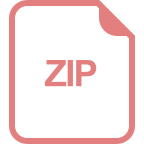







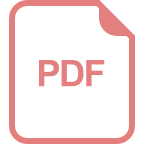
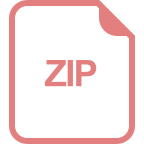