python正则匹配查找字符串的代码
时间: 2024-01-02 19:01:46 浏览: 66
可以使用 re 模块来进行正则匹配,比如通过 re.search() 函数来查找字符串中是否包含特定的模式。例如:
import re
pattern = r'hello'
string = 'hello world'
match = re.search(pattern, string)
if match:
print("Found a match!")
else:
print("No match found.")
相关问题
python 正则表达式查找字符串中两个字符串之间的数据的程序
同样可以使用Python中的re模块实现正则表达式查找字符串中两个字符串之间的数据。以下是一个示例程序:
```python
import re
string = "The text is between 'start' and 'end'"
match = re.search(r"'(.*?)'", string)
if match:
print(match.group(1))
else:
print("No match")
```
在这个示例中,我们使用正则表达式查找字符串中"'start'"和"'end'"之间的文本。正则表达式是"'(.*?)'",其中"'"表示匹配单引号,"(.*?)"表示匹配任意字符,但是尽可能少地匹配。如果找到匹配项,则打印结果"start"和"end"。注意,在正则表达式中使用了"?"字符,这是一个非贪婪匹配,确保我们只捕获两个单引号之间的最小文本段。
python 正则表达式查找字符串中两个字符之间的数据的程序
可以使用Python中的re模块实现正则表达式查找字符串中两个字符之间的数据。以下是一个示例程序:
```python
import re
string = "The price is $10.50 per unit"
match = re.search(r'(?<=\$)\d+\.\d+', string)
if match:
print(match.group(0))
else:
print("No match")
```
在这个示例中,我们使用正则表达式查找字符串中"$"和"per unit"之间的数字。正则表达式是"(?<=\$)\d+\.\d+",其中"(?<=\$)"表示查找前面是"$"的位置,"\d+"表示匹配一个或多个数字,"\."表示匹配小数点,"\d+"再次表示匹配一个或多个数字。如果找到匹配项,则打印结果"$10.50"。
阅读全文
相关推荐
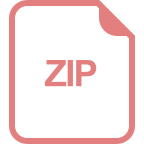
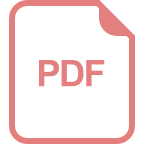


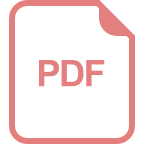
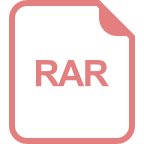
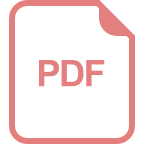
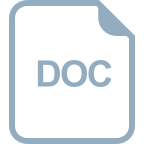
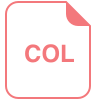
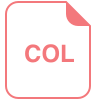




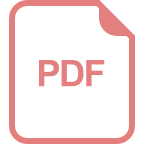