unity代码实现打字机效果,要求使用doweent实现按下ESC键后,文字立即全部显示
时间: 2024-03-05 18:51:59 浏览: 45
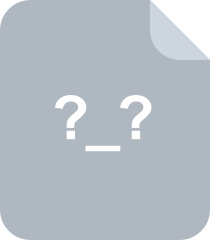
unity UGUI实现打字机效果
在Unity中实现打字机效果,可以使用协程(Coroutine)来控制每个字符的显示,并使用`Input.GetKeyDown(KeyCode.Escape)`来监听ESC键的按下。代码如下:
```c#
using UnityEngine;
using UnityEngine.UI;
using System.Collections;
public class TypewriterEffect : MonoBehaviour
{
public float delay = 0.1f; // 每个字的打印速度
public Text textComponent;
public string message;
private bool isTyping = true;
private bool cancelTyping = false;
private void Start()
{
StartCoroutine(TypeText());
}
private IEnumerator TypeText()
{
foreach (char letter in message.ToCharArray())
{
if (cancelTyping)
{
break;
}
textComponent.text += letter;
yield return new WaitForSeconds(delay);
}
isTyping = false;
}
private void Update()
{
if (isTyping && Input.GetKeyDown(KeyCode.Escape))
{
cancelTyping = true; // 立即显示全部文字
textComponent.text = message;
}
}
}
```
首先,在`Start`函数中启动协程`TypeText`,在其中使用`foreach`循环逐个打印每个字符,并根据`delay`变量设置打印速度。同时,设置`isTyping`标志位表示是否正在打印,`cancelTyping`标志位表示是否取消打印。
然后,在`Update`函数中监听ESC键的按下,如果按下了ESC键,则将`cancelTyping`标志位设置为`true`,并将`textComponent.text`设置为完整的字符串,即可立即显示全部文字。
需要注意的是,使用协程时需要使用`yield return`语句来控制等待时间。另外,在使用协程时需要注意协程的生命周期,确保在需要时及时停止协程。
阅读全文
相关推荐
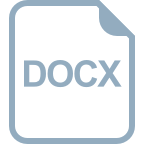
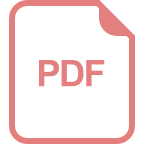
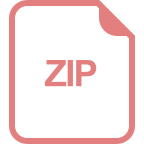
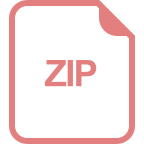
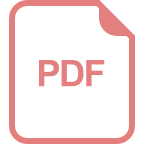
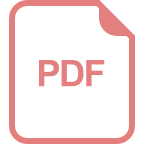
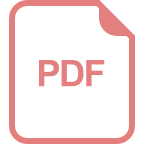
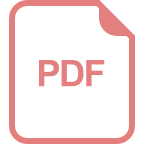
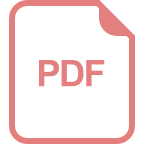
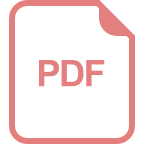
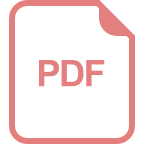
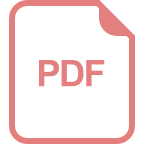
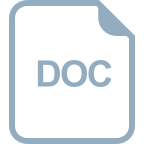