C++ Builder中在不使用TComPort组件的情况下如何搭建Uart串口通信?
时间: 2024-02-12 14:06:51 浏览: 270
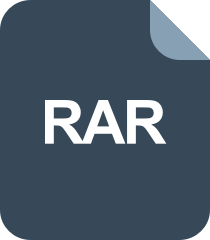
uart.rar_C Builder_串口 api

在C++ Builder中实现Uart串口通信的方法有很多种,下面介绍两种常用的方法。
方法一:使用Windows API函数
可以使用Windows API函数实现串口通信,如CreateFile、ReadFile、WriteFile、SetCommState等。以下是一个简单的例子:
```c++
#include <Windows.h>
HANDLE hCom;
void OpenCom()
{
hCom = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
if (hCom == INVALID_HANDLE_VALUE)
{
MessageBox(NULL, "打开串口失败!", "错误提示", MB_OK);
return;
}
DCB dcb;
GetCommState(hCom, &dcb);
dcb.BaudRate = 9600;
dcb.ByteSize = 8;
dcb.Parity = NOPARITY;
dcb.StopBits = ONESTOPBIT;
SetCommState(hCom, &dcb);
}
void CloseCom()
{
CloseHandle(hCom);
}
void SendData(char* pData, int nLen)
{
DWORD dwBytesWritten;
WriteFile(hCom, pData, nLen, &dwBytesWritten, NULL);
}
void ReceiveData(char* pData, int nLen)
{
DWORD dwBytesRead;
ReadFile(hCom, pData, nLen, &dwBytesRead, NULL);
}
```
方法二:使用第三方库
也可以使用第三方库,如Boost.Asio、Serial Communication Library (SCL)等。以下是一个使用Boost.Asio的例子:
```c++
#include <boost/asio.hpp>
#include <boost/bind.hpp>
using namespace boost::asio;
io_service io;
serial_port port(io, "COM1");
char data[1024];
void SendData(char* pData, int nLen)
{
write(port, buffer(pData, nLen));
}
void ReceiveData()
{
port.async_read_some(buffer(data), boost::bind(&ReceiveHandler, _1, _2));
}
void ReceiveHandler(const boost::system::error_code& error, std::size_t bytes_transferred)
{
if (!error)
{
// 处理接收到的数据
}
ReceiveData();
}
```
需要注意的是,以上代码仅为演示用途,实际应用中需要对异常情况进行处理。
阅读全文
相关推荐
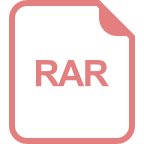
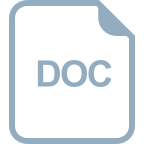



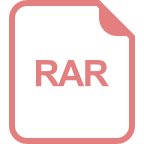
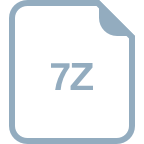
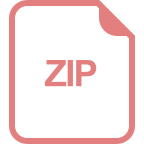
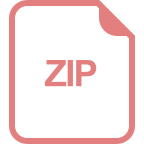
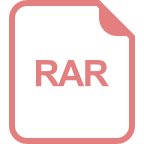
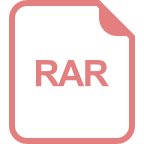
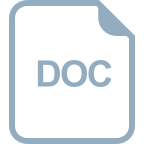
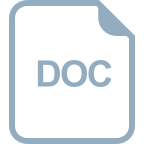
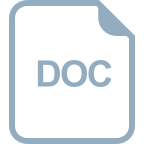
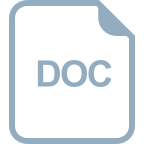
