蚁群算法解决旅行商问题c++
时间: 2023-09-22 20:13:06 浏览: 88
非常感谢您的提问。关于蚁群算法解决旅行商问题,是一个非常经典的应用。蚁群算法是一种仿生算法,模拟蚂蚁在寻觅食物过程中释放信息素、遵循信息素浓度高的路径,从而逐渐找到最优解的过程。在旅行商问题中,蚂蚁模拟器会模拟多个蚂蚁同时搜索路径,然后根据路径长度和信息素浓度等因素,通过迭代优化不断得到最优解。这是一种非常有效的算法,被广泛应用于各种问题的最优化求解过程。
相关问题
用蚁群算法解决旅行商问题c++
蚁群算法是一种模拟蚂蚁觅食行为的启发式算法,可以用于解决旅行商问题(TSP)。下面是一个用C++实现蚁群算法解决旅行商问题的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_CITIES = 10; // 城市数量
const int MAX_ANTS = 10; // 蚂蚁数量
const int MAX_ITERATIONS = 100; // 最大迭代次数
const double ALPHA = 1.0; // 信息素重要程度因子
const double BETA = 2.0; // 启发函数重要程度因子
const double RHO = 0.5; // 信息素蒸发系数
const double Q = 100.0; // 信息素增加强度系数
struct City {
double x, y;
};
double distance(const City& city1, const City& city2) {
double dx = city1.x - city2.x;
double dy = city1.y - city2.y;
return sqrt(dx * dx + dy * dy);
}
class Ant {
public:
Ant() {
tabu.resize(MAX_CITIES, false);
path.resize(MAX_CITIES);
}
void clear() {
for (int i = 0; i < MAX_CITIES; ++i) {
tabu[i] = false;
path[i] = 0;
}
}
void visitCity(int city) {
tabu[city] = true;
path[currentCity] = city;
currentCity = city;
tourLength += distance(cities[path[currentCity]], cities[path[currentCity - 1]]);
}
int getCurrentCity() const {
return currentCity;
}
double getTourLength() const {
return tourLength;
}
void setCurrentCity(int city) {
currentCity = city;
}
private:
vector<bool> tabu;
vector<int> path;
int currentCity = 0;
double tourLength = 0.0;
};
class ACO {
public:
ACO() {
cities.resize(MAX_CITIES);
ants.resize(MAX_ANTS);
pheromone.resize(MAX_CITIES, vector<double>(MAX_CITIES, 1.0));
// 初始化城市坐标
for (int i = 0; i < MAX_CITIES; ++i) {
cities[i].x = rand() % 100;
cities[i].y = rand() % 100;
}
// 初始化蚂蚁
for (int i = 0; i < MAX_ANTS; ++i) {
ants[i].clear();
ants[i].setCurrentCity(rand() % MAX_CITIES);
}
}
void updatePheromone() {
for (int i = 0; i < MAX_CITIES; ++i) {
for (int j = 0; j < MAX_CITIES; ++j) {
pheromone[i][j] *= (1.0 - RHO);
}
}
for (int i = 0; i < MAX_ANTS; ++i) {
for (int j = 0; j < MAX_CITIES; ++j) {
int city1 = ants[i].path[j];
int city2 = ants[i].path[(j + 1) % MAX_CITIES];
pheromone[city1][city2] += Q / ants[i].getTourLength();
pheromone[city2][city1] += Q / ants[i].getTourLength();
}
}
}
void antColonyOptimization() {
for (int iteration = 0; iteration < MAX_ITERATIONS; ++iteration) {
for (int i = 0; i < MAX_ANTS; ++i) {
while (ants[i].getCurrentCity() != -1) {
int nextCity = selectNextCity(ants[i]);
ants[i].visitCity(nextCity);
}
if (ants[i].getTourLength() < bestTourLength) {
bestTourLength = ants[i].getTourLength();
bestTour = ants[i].path;
}
ants[i].clear();
ants[i].setCurrentCity(rand() % MAX_CITIES);
}
updatePheromone();
}
}
int selectNextCity(const Ant& ant) {
int currentCity = ant.getCurrentCity();
double sum = 0.0;
for (int i = 0; i < MAX_CITIES; ++i) {
if (!ant.tabu[i]) {
sum += pow(pheromone[currentCity][i], ALPHA) * pow(1.0 / distance(cities[currentCity], cities[i]), BETA);
}
}
double r = (double)rand() / RAND_MAX;
double probability = 0.0;
for (int i = 0; i < MAX_CITIES; ++i) {
if (!ant.tabu[i]) {
probability += pow(pheromone[currentCity][i], ALPHA) * pow(1.0 / distance(cities[currentCity], cities[i]), BETA) / sum;
if (r <= probability) {
return i;
}
}
}
return -1;
}
void printBestTour() {
cout << "Best tour: ";
for (int i = 0; i < MAX_CITIES; ++i) {
cout << bestTour[i] << " ";
}
cout << endl;
cout << "Best tour length: " << bestTourLength << endl;
}
private:
vector<City> cities;
vector<Ant> ants;
vector<vector<double>> pheromone;
vector<int> bestTour;
double bestTourLength = numeric_limits<double>::max();
};
int main() {
srand(time(nullptr));
ACO aco;
aco.antColonyOptimization();
aco.printBestTour();
return 0;
}
```
这段代码实现了蚁群算法解决旅行商问题。它使用了随机生成的城市坐标作为输入,通过迭代更新信息素矩阵和蚂蚁的路径来寻找最优的旅行路径。最终输出最优路径和路径长度。
用蚁群算法求解旅行商问题c++模板
以下是使用蚁群算法求解旅行商问题的C++模板代码:
```
#include <iostream>
#include <cmath>
#include <vector>
#include <limits>
#include <algorithm>
#include <random>
using namespace std;
const int MAX_N = 100; // 最大城市数量
const int MAX_GEN = 1000; // 最大迭代次数
const int ANT_NUM = 50; // 蚂蚁数量
const double ALPHA = 1.0; // 信息素重要程度因子
const double BETA = 5.0; // 距离的重要程度因子
const double RHO = 0.5; // 信息素挥发因子
const double Q = 100.0; // 信息素增加强度系数
const double INIT_PHEROMONE = 1.0; // 初始信息素浓度
const double BEST_PHEROMONE = 2.0; // 最优路径信息素浓度
int n; // 城市数量
double dist[MAX_N][MAX_N]; // 城市之间的距离
double pheromone[MAX_N][MAX_N]; // 信息素浓度
int path[ANT_NUM][MAX_N]; // 蚂蚁路径
double path_length[ANT_NUM]; // 蚂蚁路径长度
int best_path[MAX_N]; // 最优路径
double best_path_length = numeric_limits<double>::max(); // 最优路径长度
// 计算两个城市之间的距离
double calc_distance(int i, int j) {
double x1 = 0, y1 = 0, x2 = 0, y2 = 0;
// 计算第i个城市的坐标
// ...
// 计算第j个城市的坐标
// ...
return sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
}
// 初始化距离和信息素
void init() {
// 初始化距离
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
double d = calc_distance(i, j);
dist[i][j] = d;
dist[j][i] = d;
}
}
// 初始化信息素
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
pheromone[i][j] = INIT_PHEROMONE;
}
}
}
// 蚂蚁选择下一个城市
int select_next_city(int ant, int cur_city, bool visited[]) {
// 计算当前城市到其他城市的信息素和距离的乘积
vector<double> p(n, 0.0);
double p_total = 0.0;
for (int i = 0; i < n; i++) {
if (!visited[i]) {
p[i] = pow(pheromone[cur_city][i], ALPHA) * pow(1.0 / dist[cur_city][i], BETA);
p_total += p[i];
}
}
// 轮盘赌选择下一个城市
uniform_real_distribution<double> u(0.0, p_total);
double rand_val = u(default_random_engine());
int next_city = -1;
for (int i = 0; i < n; i++) {
if (!visited[i]) {
rand_val -= p[i];
if (rand_val <= 0.0) {
next_city = i;
break;
}
}
}
return next_city;
}
// 蚂蚁行走一步
void ant_move(int ant) {
bool visited[MAX_N] = {false};
// 蚂蚁从起点出发
path[ant][0] = ant;
visited[ant] = true;
// 蚂蚁依次访问其他城市
for (int i = 1; i < n; i++) {
int cur_city = path[ant][i - 1];
int next_city = select_next_city(ant, cur_city, visited);
path[ant][i] = next_city;
visited[next_city] = true;
path_length[ant] += dist[cur_city][next_city];
}
// 蚂蚁返回起点
int last_city = path[ant][n - 1];
path_length[ant] += dist[last_city][ant];
}
// 更新信息素
void update_pheromone() {
// 更新信息素浓度
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
pheromone[i][j] *= (1.0 - RHO);
}
}
// 更新蚂蚁路径上的信息素
for (int ant = 0; ant < ANT_NUM; ant++) {
for (int i = 0; i < n - 1; i++) {
int cur_city = path[ant][i];
int next_city = path[ant][i + 1];
pheromone[cur_city][next_city] += Q / path_length[ant];
pheromone[next_city][cur_city] += Q / path_length[ant];
}
// 更新最优路径
if (path_length[ant] < best_path_length) {
best_path_length = path_length[ant];
copy(path[ant], path[ant] + n, best_path);
}
}
// 最优路径上的信息素浓度增加
for (int i = 0; i < n - 1; i++) {
int cur_city = best_path[i];
int next_city = best_path[i + 1];
pheromone[cur_city][next_city] += BEST_PHEROMONE;
pheromone[next_city][cur_city] += BEST_PHEROMONE;
}
}
// 蚁群算法求解旅行商问题
void solve() {
// 初始化距离和信息素
init();
// 迭代搜索
for (int gen = 0; gen < MAX_GEN; gen++) {
// 蚂蚁行走
for (int ant = 0; ant < ANT_NUM; ant++) {
ant_move(ant);
}
// 更新信息素
update_pheromone();
// 输出当前结果
cout << "Generation " << gen << ": " << best_path_length << endl;
}
// 输出最终结果
cout << "Best path: ";
for (int i = 0; i < n; i++) {
cout << best_path[i] << " ";
}
cout << endl;
cout << "Best path length: " << best_path_length << endl;
}
int main() {
// 读入城市数量
cin >> n;
// 求解旅行商问题
solve();
return 0;
}
```
需要注意的是,这只是一个简单的模板,实际使用时需要根据具体问题进行调整。
相关推荐
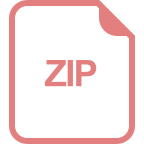
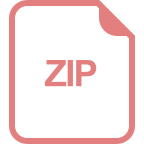








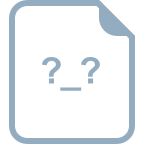
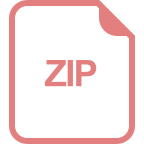
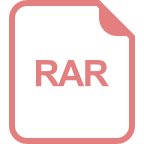
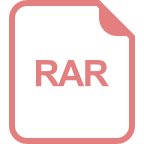