server.c:110:43: warning: passing argument 2 of ‘strcmp’ from incompatible pointer type [-Wincompatible-pointer-types] if (strcmp(result[Index], data->header.sid) == 0)
时间: 2024-04-19 14:24:57 浏览: 10
这个警告是由于`strcmp`函数的第二个参数类型与`data->header.sid`的类型不兼容导致的。`strcmp`函数的第二个参数应该是一个`const char*`类型的指针,而`data->header.sid`可能是另一种类型的指针。
要解决这个警告,你可以尝试将`data->header.sid`强制转换为`const char*`类型,或者检查`data->header.sid`的定义并确保它是一个字符串类型。另外,确保你在使用`strcmp`之前已经正确地初始化了`result[Index]`和`data->header.sid`。
相关问题
signal.c:22:13: warning: passing argument 2 of ‘signal’ from incompatible pointer type [-Wincompatible-pointer-types] signal(12,show);
这个警告是因为在调用`signal`函数时,第二个参数的类型与函数原型不匹配。根据警告信息,`show`参数的类型与`signal`函数所需的指针类型不兼容。
在C语言中,`signal`函数用于注册信号处理函数。它的原型如下:
```c
void (*signal(int sig, void (*func)(int)))(int);
```
其中,第二个参数`func`是一个指向接受一个整型参数并返回`void`的函数的指针。根据警告信息,`show`的类型似乎与这个函数指针的类型不匹配。
要解决这个问题,你需要确保`show`的类型与信号处理函数所需的函数指针类型相匹配。请检查`show`的定义和函数原型,并确保它们匹配。如果需要转换类型,请使用合适的类型转换操作符。
passing argument 3 of 'ConvertBoolArrayToMBByteArray' from incompatible pointer type [-Wincompatible-pointer-types]
这个错误信息的意思是你在调用函数 `ConvertBoolArrayToMBByteArray` 时第三个参数的指针类型与函数声明中的指针类型不兼容。需要检查一下函数声明和调用中第三个参数的指针类型是否一致。
可能的原因是你在函数声明和函数调用中使用了不同的指针类型,或者是在调用函数时传递的参数类型与函数声明中定义的类型不同。
你可以检查一下函数声明和函数调用中第三个参数的指针类型,确保它们是一致的。如果类型不同,需要进行类型转换才能调用函数。
相关推荐
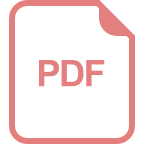
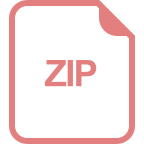
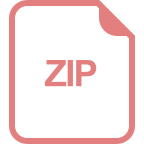













