统计从键盘输入的一行字符,统计其中的字母个数c语言代码
时间: 2024-12-01 15:30:27 浏览: 0
在C语言中,你可以通过遍历用户输入的一行字符串,并检查每个字符是否为字母来统计字母的数量。这里是一个简单的示例代码:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的功能
int main() {
char input[100]; // 定义一个数组用于存储用户输入
fgets(input, sizeof(input), stdin); // 从键盘读取一行输入
int letter_count = 0; // 初始化字母计数器
for (int i = 0; input[i] != '\0'; i++) { // 遍历输入字符串
if (isalpha(input[i])) { // 检查字符是否为字母(包括大写和小写字母)
letter_count++; // 如果是字母,则计数器加一
}
}
printf("字母数量: %d\n", letter_count); // 输出字母总数
return 0;
}
```
在这个代码里,`isalpha()` 函数会判断一个字符是否为字母。运行程序后,它会等待用户的输入,然后计算并打印出输入字符串中的字母个数。
相关问题
从键盘输入一行字符统计字母空格数字及其其他字符的个数C语言
以下是两种C语言统计输入字符中字母、空格、数字和其他字符个数的方法:
方法一:常规计算
```c
#include <stdio.h>
int main(){
char c;
int letters=0,space=0,digit=0,other=0;
printf("请输入一行字符:\n");
while((c=getchar())!='\n'){
if(c>='a'&&c<='z'||c>='A'&&c<='Z') letters++;
else if(c==' ') space++;
else if(c>='0'&&c<='9') digit++;
else other++;
}
printf("字母数:%d\n空格数:%d\n数字数:%d\n其他字符数:%d",letters,space,digit,other);
return 0;
}
```
方法二:普通函数调用
```c
#include <stdio.h>
#include <string.h>
void count(char c[]){
int letters=0,space=0,digit=0,other=0;
int i;
for (i = 0; c[i]; i++) {
if(c[i]>='a'&&c[i]<='z'||c[i]>='A'&&c[i]<='Z') letters++;
else if(c[i]==' ') space++;
else if(c[i]>='0'&&c[i]<='9') digit++;
else other++;
}
printf("字母数:%d\n空格数:%d\n数字数:%d\n其他字符数:%d",letters,space,digit,other);
}
int main(){
char c[32];
printf("请输入一行字符:\n");
gets(c);
count(c);
return 0;
}
```
c语言从键盘输入一行字符,统计其中大写字符的个数,小写字符的个数,数字的个数,以及非字母和数字的个数。请分别用for,while循环语句实现 从键盘输入一行字符,统计其中大写字符的个数,小写字符的个数,数字的个数,以及非字母和数字的个数。请分别用for,while循环语句实现
// for循环实现
#include <stdio.h>
int main() {
char c;
int upper = 0, lower = 0, digit = 0, other = 0;
printf("请输入一行字符:");
for (int i = 0; (c = getchar()) != '\n'; i++) {
if (c >= 'A' && c <= 'Z') {
upper++;
} else if (c >= 'a' && c <= 'z') {
lower++;
} else if (c >= '0' && c <= '9') {
digit++;
} else {
other++;
}
}
printf("大写字母:%d\n", upper);
printf("小写字母:%d\n", lower);
printf("数字:%d\n", digit);
printf("其他字符:%d\n", other);
return 0;
}
// while循环实现
#include <stdio.h>
int main() {
char c;
int upper = 0, lower = 0, digit = 0, other = 0;
printf("请输入一行字符:");
c = getchar();
while (c != '\n') {
if (c >= 'A' && c <= 'Z') {
upper++;
} else if (c >= 'a' && c <= 'z') {
lower++;
} else if (c >= '0' && c <= '9') {
digit++;
} else {
other++;
}
c = getchar();
}
printf("大写字母:%d\n", upper);
printf("小写字母:%d\n", lower);
printf("数字:%d\n", digit);
printf("其他字符:%d\n", other);
return 0;
}
阅读全文
相关推荐
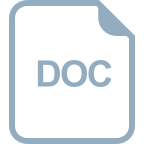
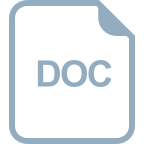
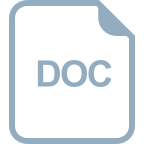













