帮我用c语言定义一个链表
时间: 2023-09-30 14:02:08 浏览: 87
以下是一个简单的链表定义:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct node {
int data; // 节点数据
struct node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
int main() {
// 创建链表头节点
Node* head = createNode(0);
// 在链表尾部添加新节点
Node* tail = head;
for (int i = 1; i <= 5; i++) {
Node* newNode = createNode(i);
tail->next = newNode;
tail = newNode;
}
// 遍历链表并输出节点数据
Node* current = head->next;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
// 释放链表内存
current = head;
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
return 0;
}
```
这个链表包含一个头节点和五个数据节点,每个数据节点包含一个整数数据和一个指向下一个数据节点的指针。链表的创建和遍历使用了指针操作。链表的释放需要遍历整个链表并释放每个节点的内存。
阅读全文
相关推荐
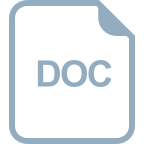
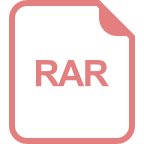
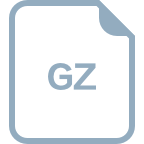

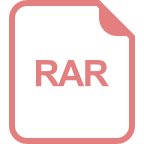










