创建一个简单的javaweb项目能实现分页功能,在jsp页面中有一个表格显示studentID,sNum,name,gender,age,tel这六种数据,这六种数据是从我的student数据库中的students表获取的数据,同时在这个jsp页面下方要显示页码,每页显示8条数据。还需要在这个项目创建com.jr.comtroller包,在这个包里面创建StudentController.java文件。还需要在这个项目创建com.jr.dao包,在这个包里面创建StudentMapper.java文件和StudentMapper.xml文件。还需要在这个项目创建com.jr.domain包,在这个包里面创建StudentService.java文件和StudentServiceImpl.java文件。请展示我所描述的这个项目的每个文件的完整代码,并有详细讲解。
时间: 2024-01-22 18:18:46 浏览: 93
好的,下面是该项目的实现过程及每个文件的代码和讲解。
1. 创建javaweb项目
在IDE中新建一个Dynamic Web Project,命名为"StudentManagement"。
2. 添加依赖
该项目需要使用Mybatis和MySQL数据库,因此需要添加以下依赖:
```xml
<!-- Mybatis依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
<!-- MySQL数据库依赖 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
```
3. 创建数据库
该项目需要连接MySQL数据库,因此需要先创建一个数据库和表来存储学生信息。可以通过以下SQL语句来创建:
```sql
CREATE DATABASE student;
USE student;
CREATE TABLE students(
id INT(11) PRIMARY KEY AUTO_INCREMENT,
s_num VARCHAR(20) NOT NULL,
name VARCHAR(20) NOT NULL,
gender VARCHAR(2) NOT NULL,
age INT(2) NOT NULL,
tel VARCHAR(20) NOT NULL
);
```
4. 创建实体类
在项目中创建com.jr.domain包,然后在该包中创建一个Student实体类,用于存储学生信息。
```java
public class Student {
private int id;
private String sNum;
private String name;
private String gender;
private int age;
private String tel;
// 省略getter和setter方法
}
```
5. 创建Mybatis映射文件
在项目中创建com.jr.dao包,然后在该包中创建一个StudentMapper接口和一个StudentMapper.xml文件。
StudentMapper.java文件:
```java
public interface StudentMapper {
List<Student> getStudents(int start, int pageSize);
int getStudentCount();
}
```
StudentMapper.xml文件:
```xml
<mapper namespace="com.jr.dao.StudentMapper">
<select id="getStudents" resultType="com.jr.domain.Student">
SELECT * FROM students LIMIT #{start}, #{pageSize}
</select>
<select id="getStudentCount" resultType="int">
SELECT COUNT(*) FROM students
</select>
</mapper>
```
6. 创建Service接口和实现类
在项目中创建com.jr.service包,然后在该包中创建一个StudentService接口和一个StudentServiceImpl实现类。
StudentService.java文件:
```java
public interface StudentService {
List<Student> getStudents(int pageNum);
}
```
StudentServiceImpl.java文件:
```java
public class StudentServiceImpl implements StudentService {
private SqlSessionFactory sqlSessionFactory;
public StudentServiceImpl(SqlSessionFactory sqlSessionFactory) {
this.sqlSessionFactory = sqlSessionFactory;
}
@Override
public List<Student> getStudents(int pageNum) {
try (SqlSession sqlSession = sqlSessionFactory.openSession()) {
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
int pageSize = 8;
int start = (pageNum - 1) * pageSize;
return mapper.getStudents(start, pageSize);
}
}
}
```
7. 创建Controller类
在项目中创建com.jr.controller包,然后在该包中创建一个StudentController类,用于处理请求和响应。
StudentController.java文件:
```java
@WebServlet("/student")
public class StudentController extends HttpServlet {
private SqlSessionFactory sqlSessionFactory;
public void init() throws ServletException {
try {
InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml");
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int pageNum = Integer.parseInt(request.getParameter("pageNum"));
StudentService studentService = new StudentServiceImpl(sqlSessionFactory);
List<Student> students = studentService.getStudents(pageNum);
int studentCount = studentService.getStudentCount();
int pageCount = (int) Math.ceil(studentCount / 8.0);
request.setAttribute("students", students);
request.setAttribute("pageCount", pageCount);
request.setAttribute("pageNum", pageNum);
request.getRequestDispatcher("/student.jsp").forward(request, response);
}
}
```
8. 创建jsp页面
在项目中创建一个student.jsp文件,用于显示学生信息和页码。
student.jsp文件:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统</title>
</head>
<body>
<table border="1">
<tr>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
<th>电话</th>
</tr>
<c:forEach items="${students}" var="student">
<tr>
<td>${student.sNum}</td>
<td>${student.name}</td>
<td>${student.gender}</td>
<td>${student.age}</td>
<td>${student.tel}</td>
</tr>
</c:forEach>
</table>
<br>
<c:if test="${pageCount > 1}">
<c:choose>
<c:when test="${pageNum == 1}">
<a href="${pageContext.request.contextPath}/student?pageNum=1">1</a>
</c:when>
<c:otherwise>
<a href="${pageContext.request.contextPath}/student?pageNum=1">1</a>
<a href="${pageContext.request.contextPath}/student?pageNum=${pageNum - 1}">上一页</a>
</c:otherwise>
</c:choose>
<c:forEach begin="2" end="${pageCount - 1}" var="num">
<c:if test="${pageNum == num}">
<a href="${pageContext.request.contextPath}/student?pageNum=${num}"><strong>${num}</strong></a>
</c:if>
<c:if test="${pageNum != num}">
<a href="${pageContext.request.contextPath}/student?pageNum=${num}">${num}</a>
</c:if>
</c:forEach>
<c:choose>
<c:when test="${pageNum == pageCount}">
<a href="${pageContext.request.contextPath}/student?pageNum=${pageCount}">${pageCount}</a>
</c:when>
<c:otherwise>
<a href="${pageContext.request.contextPath}/student?pageNum=${pageNum + 1}">下一页</a>
<a href="${pageContext.request.contextPath}/student?pageNum=${pageCount}">${pageCount}</a>
</c:otherwise>
</c:choose>
</c:if>
</body>
</html>
```
9. 配置Mybatis
在项目中创建一个mybatis-config.xml文件,用于配置Mybatis。
mybatis-config.xml文件:
```xml
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/student"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="com/jr/dao/StudentMapper.xml"/>
</mappers>
</configuration>
```
10. 部署运行
将该项目部署到Tomcat服务器上运行,访问http://localhost:8080/StudentManagement/student?pageNum=1,即可看到分页效果。
以上就是该项目的完整代码和讲解,希望对您有所帮助。
阅读全文
相关推荐















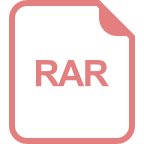
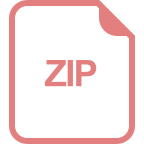