使用javascript在页面中生成100个图片,并用js对图片添加事件
时间: 2024-04-16 08:26:40 浏览: 87
可以使用以下代码来生成100个图片并添加事件:
```javascript// 创建图片数组
var images = [];
// 生成100个图片
for (var i = 0; i < 100; i++) {
var img = document.createElement('img');
img.src = 'image.jpg'; // 设置图片路径
img.addEventListener('click', function() {
// 点击事件处理逻辑
console.log('图片被点击了');
});
images.push(img);
}
// 将图片添加到页面中
var container = document.getElementById('container'); // 假设有一个id为container的容器元素
images.forEach(function(img) {
container.appendChild(img);
});
```
在上面的代码中,我们使用了一个循环来生成100个`<img>`元素,并给每个元素添加了一个点击事件监听器。在点击事件处理逻辑中,我们简单地打印了一条消息到控制台。
最后,我们将生成的图片元素添加到页面中的一个容器元素(假设其id为`container`)中。你可以根据实际情况修改代码来适应你的页面布局和需求。
相关问题
用html帮我写一个登录页面,并用JavaScript生成随机验证码
好的,以下是一个简单的登录页面示例,包含了一个随机生成验证码的JavaScript函数:
```html
<!DOCTYPE html>
<html>
<head>
<title>登录页面</title>
<script>
// 生成随机验证码
function generateCode() {
var codeLength = 6; // 验证码长度
var codeChars = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"; // 验证码字符集合
var code = "";
for (var i = 0; i < codeLength; i++) {
code += codeChars.charAt(Math.floor(Math.random() * codeChars.length));
}
document.getElementById("code").innerHTML = code; // 将验证码更新到页面
}
</script>
</head>
<body>
<h2>登录</h2>
<form>
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br><br>
<label for="code">验证码:</label>
<input type="text" id="codeInput" name="codeInput" style="width: 100px;"> <span id="code" onclick="generateCode()">点击刷新</span><br><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
这个页面包含了一个用户名输入框、一个密码输入框、一个验证码输入框和一个登录按钮。当用户点击“点击刷新”时,`generateCode()`函数将生成一个6位长度的随机验证码,并将其更新到页面上的`<span>`元素中。用户输入正确的验证码后,点击“登录”按钮即可提交表单。
需要注意的是,这个验证码只是一个简单的示例,安全性并不高。在实际应用中,需要使用更加复杂的验证码来防止恶意攻击。
使用javaScript实现遗传算法,并用CSS封装
遗传算法是一种优化算法,可以用于求解各种问题,例如函数极值、组合优化、路径规划等。以下是使用JavaScript实现遗传算法的简单示例代码,并用CSS进行封装:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>遗传算法示例</title>
<style>
.result {
margin-top: 20px;
padding: 10px;
background-color: #eee;
border: 1px solid #aaa;
}
.chart {
margin-top: 20px;
padding: 10px;
background-color: #eee;
border: 1px solid #aaa;
}
</style>
</head>
<body>
<h1>遗传算法示例</h1>
<p>求解函数 y = x^2 在区间 [-5, 5] 中的最大值。</p>
<button onclick="runGA()">运行算法</button>
<div class="result">
<h2>结果</h2>
<p>最优解 x: <span id="x"></span></p>
<p>最优解 y: <span id="y"></span></p>
</div>
<div class="chart">
<h2>收敛曲线</h2>
<canvas id="chart"></canvas>
</div>
<script>
// 遗传算法参数
const popSize = 50; // 种群大小
const eliteSize = 10; // 精英个数
const mutationRate = 0.01; // 变异概率
const numGenerations = 100; // 迭代次数
// 适应度函数(目标函数)
function fitness(x) {
return x * x;
}
// 生成随机数
function random(min, max) {
return Math.random() * (max - min) + min;
}
// 初始化种群
function initPopulation() {
let population = [];
for (let i = 0; i < popSize; i++) {
population.push(random(-5, 5));
}
return population;
}
// 计算适应度
function calcFitness(population) {
let fitnessScores = [];
for (let i = 0; i < population.length; i++) {
let x = population[i];
let y = fitness(x);
fitnessScores.push(y);
}
return fitnessScores;
}
// 选择
function selection(population, fitnessScores) {
let elite = [];
for (let i = 0; i < eliteSize; i++) {
let maxIndex = fitnessScores.indexOf(Math.max(...fitnessScores));
elite.push(population[maxIndex]);
population.splice(maxIndex, 1);
fitnessScores.splice(maxIndex, 1);
}
return elite;
}
// 交叉
function crossover(elite) {
let offspring = [];
while (offspring.length < popSize - eliteSize) {
let parent1 = elite[Math.floor(Math.random() * elite.length)];
let parent2 = elite[Math.floor(Math.random() * elite.length)];
if (parent1 !== parent2) {
let child = (parent1 + parent2) / 2;
offspring.push(child);
}
}
return offspring;
}
// 变异
function mutation(offspring) {
for (let i = 0; i < offspring.length; i++) {
if (Math.random() < mutationRate) {
offspring[i] += random(-1, 1);
}
}
return offspring;
}
// 运行遗传算法
function runGA() {
let population = initPopulation();
let fitnessScores = calcFitness(population);
let elite = selection(population, fitnessScores);
let chartData = [{x: 0, y: Math.max(...fitnessScores)}];
for (let i = 0; i < numGenerations; i++) {
let offspring = crossover(elite);
offspring = mutation(offspring);
population = elite.concat(offspring);
fitnessScores = calcFitness(population);
elite = selection(population, fitnessScores);
chartData.push({x: i + 1, y: Math.max(...fitnessScores)});
}
let maxIndex = fitnessScores.indexOf(Math.max(...fitnessScores));
let x = population[maxIndex];
let y = fitness(x);
document.getElementById("x").innerHTML = x.toFixed(2);
document.getElementById("y").innerHTML = y.toFixed(2);
let ctx = document.getElementById("chart").getContext("2d");
new Chart(ctx, {
type: "line",
data: {
datasets: [{
label: "最优解",
data: chartData,
borderColor: "blue",
fill: false
}]
},
options: {
title: {
display: true,
text: "收敛曲线"
},
scales: {
xAxes: [{
type: "linear",
position: "bottom",
scaleLabel: {
display: true,
labelString: "迭代次数"
}
}],
yAxes: [{
type: "linear",
position: "left",
scaleLabel: {
display: true,
labelString: "适应度"
}
}]
}
}
});
}
</script>
</body>
</html>
```
在上面的示例代码中,我们定义了一个遗传算法函数`runGA()`,其中包含了遗传算法的各个步骤,例如初始化种群、计算适应度、选择、交叉、变异等。最后,我们将最优解和收敛曲线展示在网页中,并用CSS进行了一定的样式美化。
需要注意的是,上面的示例代码只是一种简单的实现方式,实际上遗传算法的性能和效果还取决于许多因素,例如选择、交叉和变异的策略、种群大小、迭代次数等。如果需要使用遗传算法求解实际问题,建议使用专业的遗传算法库或者进行更加深入的研究。
阅读全文
相关推荐










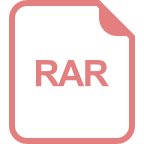





