编写一个name.c程序,每1秒钟将自己的学号姓名和当前时间写入name.txt文件。
时间: 2024-09-14 14:13:01 浏览: 44
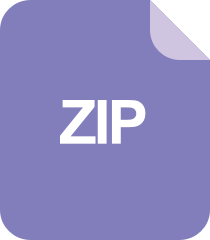
一个用C语言编写的学生管理系统.zip
编写一个名为`name.c`的程序,可以使用C语言的基本文件操作和定时器函数来实现这个需求。这里是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
#include <unistd.h> // 包含sleep函数
#define STUDENT_ID "Your_Student_ID" // 你需要将学号替换为实际值
#define FILE_NAME "name.txt"
void write_to_file() {
char timestamp[20];
struct tm *current_time;
time_t raw_time;
time(&raw_time);
current_time = localtime(&raw_time);
strftime(timestamp, sizeof(timestamp), "%Y-%m-%d %H:%M:%S", current_time);
FILE *file = fopen(FILE_NAME, "a");
if (file) {
fprintf(file, "学号: %s, 姓名: [Your_Name], 时间: %s\n", STUDENT_ID, timestamp);
fclose(file);
printf("信息已写入文件.\n");
} else {
printf("无法打开文件 %s.\n", FILE_NAME);
}
}
int main() {
while(1) {
write_to_file();
sleep(1); // 每隔1秒执行一次write_to_file
}
return 0;
}
```
在这个程序中,我们首先包含必要的头文件,然后定义了学生学号、文件名常量。`write_to_file` 函数用于获取当前时间和学生的详细信息,并将其写入文件。主函数使用无限循环,每隔1秒就调用一次`write_to_file`。
请注意,你需要替换`STUDENT_ID`为你真实的学号,以及`[Your_Name]`为你的真实姓名。运行此程序之前,确保`name.txt`文件存在或者创建好,如果不存在,程序会尝试创建它。
阅读全文
相关推荐
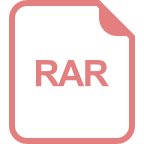
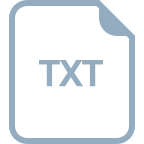















