利用try…catch…finally语句处理 除数为零 和 数组下标越界 异常的完整Java程序代码
时间: 2024-12-04 19:22:19 浏览: 13
当你需要在Java程序中处理可能会抛出异常的情况,例如除数为零或数组下标越界,你可以使用`try-catch-finally`语句结构。这里是一个示例,演示如何分别处理这两个常见的运行时异常:
```java
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
// 除数为零的例子
int dividend = 5;
int divisor = 0;
System.out.println("Result: " + divide(dividend, divisor));
// 数组下标越界的例子
int[] array = new int[3];
System.out.println("Array element: " + array[array.length]); // 这里会抛出ArrayIndexOutOfBoundsException
} catch (ArithmeticException e) { // 处理除数为零异常
System.out.println("Caught ArithmeticException: " + e.getMessage());
e.printStackTrace();
} catch (ArrayIndexOutOfBoundsException e) { // 处理数组下标越界异常
System.out.println("Caught ArrayIndexOutOfBoundsException: " + e.getMessage());
e.printStackTrace();
} finally {
System.out.println("This block always executes, regardless of whether an exception was thrown or not.");
}
}
// 定义方法并处理除法操作可能导致的异常
private static int divide(int a, int b) throws ArithmeticException {
if (b == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return a / b;
}
}
```
在这个例子中,如果`divide`方法的除数为零,它会抛出`ArithmeticException`,然后会被`catch`块捕获并打印错误信息。同样,尝试访问不存在的数组元素会引发`ArrayIndexOutOfBoundsException`,也会被捕获并处理。
阅读全文
相关推荐
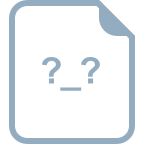
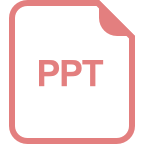
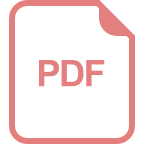

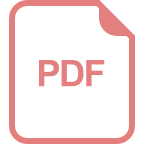
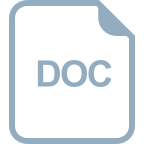
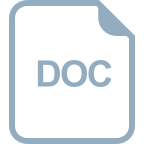
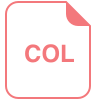










