使用bootspring写出vue考试随机组卷代码
时间: 2024-12-13 18:08:27 浏览: 37
好的,我来为您介绍一下如何使用Spring Boot和Vue.js来编写一个考试随机组卷的代码。
前端(Vue.js):
1. 首先,我们需要在Vue项目中安装axios用于HTTP请求:
```
npm install axios
```
2. 然后,我们可以创建一个组件来显示随机生成的试卷:
```vue
<template>
<div>
<h1>随机生成的试卷</h1>
<ul>
<li v-for="(question, index) in questions" :key="index">
{{ index + 1 }}. {{ question.content }}
</li>
</ul>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
questions: []
}
},
created() {
this.getRandomQuestions()
},
methods: {
async getRandomQuestions() {
try {
const response = await axios.get('/api/exams/random')
this.questions = response.data
} catch (error) {
console.error('获取随机试题失败:', error)
}
}
}
}
</script>
```
后端(Spring Boot):
1. 首先,我们需要创建一个控制器来处理随机组卷的请求:
```java
@RestController
@RequestMapping("/api/exams")
public class ExamController {
@Autowired
private QuestionService questionService;
@GetMapping("/random")
public List<Question> getRandomQuestions() {
return questionService.getRandomQuestions(10); // 例如,生成10道随机题
}
}
```
2. 接下来,我们需要实现QuestionService:
```java
@Service
public class QuestionService {
@Autowired
private QuestionRepository questionRepository;
public List<Question> getRandomQuestions(int count) {
List<Question> allQuestions = questionRepository.findAll();
Collections.shuffle(allQuestions);
return allQuestions.subList(0, Math.min(count, allQuestions.size()));
}
}
```
3. 最后,我们需要创建一个Question实体和对应的Repository:
```java
@Entity
public class Question {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String content;
// 其他字段和方法...
}
public interface QuestionRepository extends JpaRepository<Question, Long> {
}
```
这个实现可以随机从数据库中选取指定数量的试题。为了提高性能,对于大型题库,可以考虑使用缓存或更高级的随机算法。
阅读全文
相关推荐
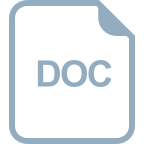
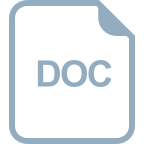
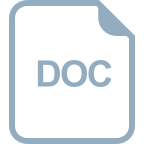
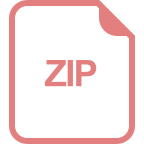
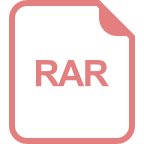
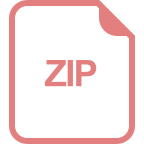
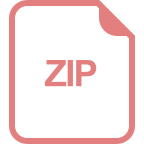
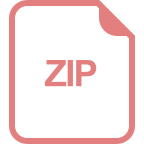
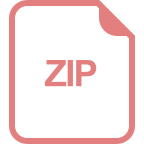
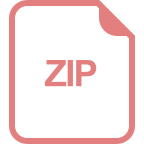
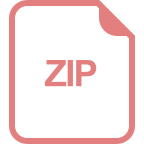
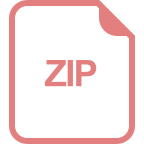
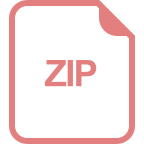
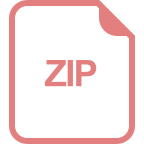
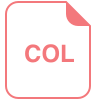
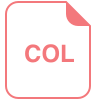
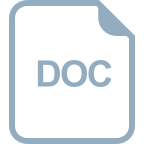
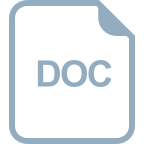