定义computecart函数计算每一种商品的总价和所用商品总价 为加减按钮定义事件及方法,实现数量加减功能,每次加1或者减1,当数量为1时,不再减。在js中
时间: 2024-10-27 14:04:36 浏览: 18
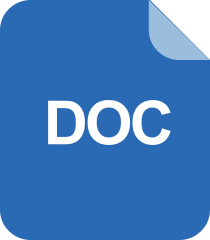
线性表设计100位以内的长整数加减运算的程序
在JavaScript中,我们可以定义一个`computeCart`函数来计算单个商品的总价和整个购物车的总价。同时,为了实现加减按钮的功能,我们需要设置点击事件处理程序,动态地更新商品数量并控制最小数量限制(即1)。以下是一个简单的示例:
```javascript
// 假设我们有商品对象,包含name、price和quantity属性
var products = [
{ name: "商品A", price: 10, quantity: 0 },
{ name: "商品B", price: 5, quantity: 0 },
// 添加更多商品...
];
function computeCart(product) {
var totalPrice = product.price * product.quantity;
return totalPrice;
}
// 计算购物车总价
function cartTotal(cartItems) {
var total = 0;
for (var i = 0; i < cartItems.length; i++) {
total += computeCart(cartItems[i]);
}
return total;
}
// 商品元素结构
var itemTemplate = '<div class="product-item">'
+ '名称: <span id="productName">{name}</span>'
+ '价格: <span id="productPrice">{price}</span>'
+ '数量: <input type="number" id="productQuantity" min="1" value={quantity}>'
+ ' <button onclick="increment({id})">+</button> '
+ ' <button onclick="decrement({id})">-</button>'
+ '</div>';
// 初始化商品展示
document.body.innerHTML += products.map(product => itemTemplate.replace("{name}", product.name)
.replace("{price}", product.price)
.replace("{quantity}", product.quantity)
.replace("{id}", product.id)).join('');
// 动态增减数量
function increment(productId) {
var quantityElement = document.getElementById("productQuantity-" + productId);
if (quantityElement.value > 1) {
quantityElement.value++;
}
}
function decrement(productId) {
var quantityElement = document.getElementById("productQuantity-" + productId);
if (quantityElement.value > 1) {
quantityElement.value--;
} else {
// 防止数量小于1
alert("该商品数量已达到最低值");
}
}
// 给购物车添加总计
setInterval(function() {
var cartItems = Array.from(document.getElementsByClassName('product-item'));
var cartTotalDisplay = document.getElementById('cartTotal');
cartTotalDisplay.textContent = '购物车总计: ' + cartTotal(cartItems);
}, 1000); // 每秒更新一次
阅读全文
相关推荐
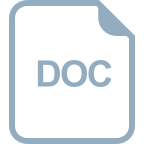
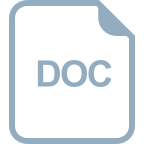
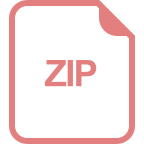
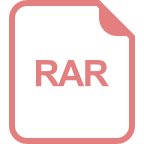
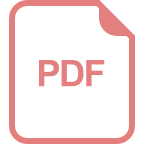
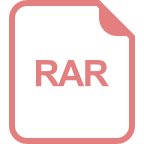
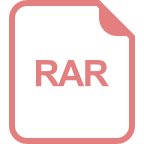
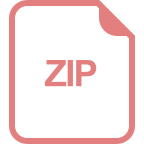
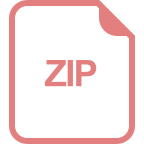
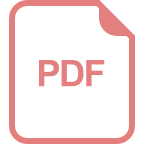
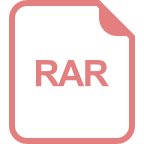
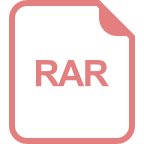
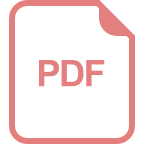
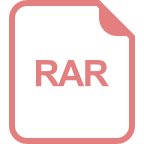
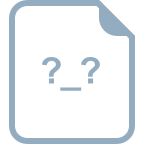
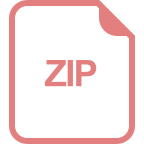
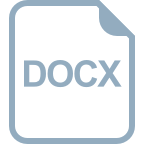