C语言 创建4个子进程,分别求2500个浮点数之和
时间: 2023-04-08 11:03:42 浏览: 116
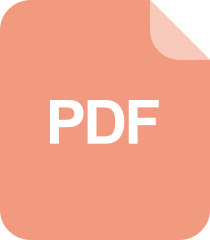
C语言浮点数运算

可以回答这个问题。C语言可以使用fork()函数创建子进程,然后在子进程中使用循环计算每个子进程需要计算的2500个浮点数之和,最后将结果返回给父进程。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#define NUM_CHILDREN 4
#define NUM_FLOATS 2500
float floats[NUM_CHILDREN][NUM_FLOATS];
float sum(float *arr, int size) {
float s = 0.0;
for (int i = 0; i < size; i++) {
s += arr[i];
}
return s;
}
int main() {
pid_t pid;
int status;
float total = 0.0;
// Generate random floats
for (int i = 0; i < NUM_CHILDREN; i++) {
for (int j = 0; j < NUM_FLOATS; j++) {
floats[i][j] = (float) rand() / RAND_MAX;
}
}
// Fork child processes
for (int i = 0; i < NUM_CHILDREN; i++) {
pid = fork();
if (pid == 0) {
// Child process
float s = sum(floats[i], NUM_FLOATS);
exit(s);
} else if (pid < 0) {
// Fork error
perror("fork");
exit(1);
}
}
// Wait for child processes to finish
for (int i = 0; i < NUM_CHILDREN; i++) {
pid = wait(&status);
if (WIFEXITED(status)) {
// Child process exited normally
total += WEXITSTATUS(status);
} else {
// Child process exited abnormally
fprintf(stderr, "Child process %d exited abnormally\n", pid);
}
}
printf("Total sum: %f\n", total);
return 0;
}
```
阅读全文
相关推荐



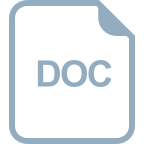
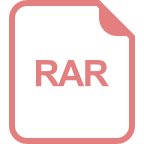
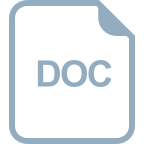
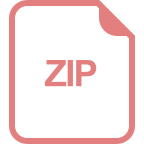
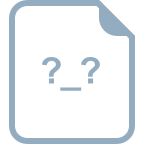
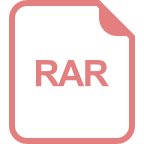
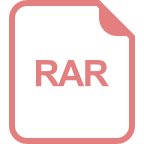
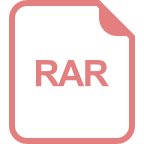
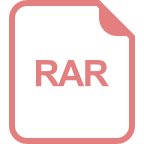
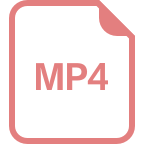