请根据所学语法,结合Python丰富的第三方库,设计一款小游戏。可以基于pygame,也可以基于其他第三方库。
时间: 2023-12-31 17:03:52 浏览: 27
好的,我可以帮你设计一款基于pygame的小游戏。这款游戏叫做“小球大作战”,玩家需要控制一个小球躲避其他球的碰撞,并在尽可能长的时间内存活下去。
首先,我们需要导入pygame库和其他必要的库。
```python
import pygame
import random
import math
```
接下来,我们需要定义一些常量和变量。
```python
# 定义窗口大小
WIDTH = 800
HEIGHT = 600
# 定义小球的半径和速度
BALL_RADIUS = 10
BALL_SPEED = 5
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义计分
score = 0
# 初始化pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("小球大作战")
```
接着,我们需要定义一个Ball类,表示小球。
```python
class Ball:
def __init__(self, x, y, speed):
self.x = x
self.y = y
self.speed = speed
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
def move(self):
direction = random.randint(0, 360)
self.x += self.speed * math.cos(math.radians(direction))
self.y += self.speed * math.sin(math.radians(direction))
def check_boundary(self):
if self.x < BALL_RADIUS or self.x > WIDTH - BALL_RADIUS:
return True
if self.y < BALL_RADIUS or self.y > HEIGHT - BALL_RADIUS:
return True
return False
def check_collision(self, other):
distance = math.sqrt((self.x - other.x) ** 2 + (self.y - other.y) ** 2)
if distance < BALL_RADIUS * 2:
return True
return False
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), BALL_RADIUS)
```
然后,我们需要创建一些小球,并在游戏循环中更新它们的位置和状态。
```python
balls = []
for i in range(10):
x = random.randint(BALL_RADIUS, WIDTH - BALL_RADIUS)
y = random.randint(BALL_RADIUS, HEIGHT - BALL_RADIUS)
ball = Ball(x, y, BALL_SPEED)
balls.append(ball)
# 游戏循环
running = True
clock = pygame.time.Clock()
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新小球的位置和状态
for ball in balls:
ball.move()
if ball.check_boundary():
ball.speed = -ball.speed
for other in balls:
if ball != other and ball.check_collision(other):
ball.speed = -ball.speed
other.speed = -other.speed
score += 1
# 绘制屏幕
screen.fill(WHITE)
for ball in balls:
ball.draw()
font = pygame.font.Font(None, 36)
text = font.render("Score: {}".format(score), True, BLACK)
screen.blit(text, (10, 10))
pygame.display.update()
# 控制帧率
clock.tick(60)
# 退出pygame
pygame.quit()
```
至此,游戏就完成了。你可以运行这个程序,体验一下这款“小球大作战”游戏。
相关推荐
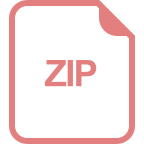
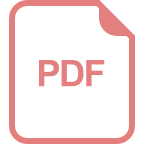
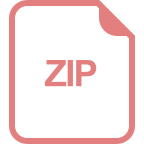














