写出matlab读取Excel中多个sheet数据的代码
时间: 2024-01-06 10:03:29 浏览: 64
可以使用Matlab中的`xlsread`函数来读取Excel中的多个sheet数据。以下是示例代码:
```matlab
% 设置文件名和sheet名
filename = 'example.xlsx';
sheet1 = 'Sheet1';
sheet2 = 'Sheet2';
% 读取第一个sheet中的数据
[data1, headers1] = xlsread(filename, sheet1);
% 读取第二个sheet中的数据
[data2, headers2] = xlsread(filename, sheet2);
```
该代码将会读取名为`example.xlsx`的Excel文件中的两个sheet(`Sheet1`和`Sheet2`)中的数据。`xlsread`函数将返回每个sheet中的数据矩阵和表头信息。你可以根据需要使用这些变量进行后续处理。
相关问题
matlab读取excel多个sheet
### 回答1:
在 Matlab 中读取 Excel 文件的多个 sheet,可以使用函数 xlsread。语法如下:
[num,txt,raw] = xlsread(filename, sheetname)
其中,filename 是 Excel 文件的名称,sheetname 是 sheet 的名称。例如:
[num1,txt1,raw1] = xlsread('test.xlsx','Sheet1');
[num2,txt2,raw2] = xlsread('test.xlsx','Sheet2');
这样就可以读取 test.xlsx 中 Sheet1 和 Sheet2 两个 sheet 的数据。
### 回答2:
MATLAB 作为一个强大的计算工具,在数据分析和处理方面有着广泛的应用。 而在许多情况下,我们需要从 Excel 表格中读取数据进入 MATLAB 中进行分析和处理。Excel 表格的数据可能会被分散在不同的工作簿中,因此,本文将介绍如何使用 MATLAB 读取 Excel 的多个 Sheet。
在 MATLAB 中,可以使用 "xlsread" 函数来读取 Excel 的数据。此函数有许多输入和输出参数,其主要用法如下:
[num,txt,raw] = xlsread(filename,sheet,range)
其中,"filename" 参数是指导入的 Excel 文件名。"sheet" 参数则是要读取的工作表的名称或索引。若要读取多个工作簿,则需要循环读取每个工作簿,这里就不再赘述。"range" 参数是指要读取的单元格范围,在这里还可以使用 ":" 来指定读取整列或整行的数据。输出参数"num"、"txt" 和 "raw" 则表示导入的数据在 MATLAB 中的不同形式。其中,"num" 表示数值型数据,"txt" 表示文本型数据,"raw" 则表示未经过 MATLAB 解释的原始数据。
下面是一个示例代码,演示了如何读取 Excel 表格中的多个工作表数据。设定文件名为 "example.xlsx",其中包含两个工作簿,名为 "Sheet1" 和 "Sheet2":
filename = 'example.xlsx';
% 读取第一个工作簿
[num1,txt1,raw1] = xlsread(filename,'Sheet1','A1:C3');
% 读取第二个工作簿
[num2,txt2,raw2] = xlsread(filename,'Sheet2','A1:C3');
% 输出结果
disp(num1);
disp(txt1);
disp(num2);
disp(txt2);
代码中,先使用 "xlsread" 函数分别读取了两个工作簿的数据,然后对这些数据进行了输出。在这里,我们只读取了每个工作簿的前三行三列数据。根据您的需求,你可以设置 "range" 来读取表格中的不同数据。至此,我们已经成功读取了 Excel 表格中的多个工作表,将数据导入到了 MATLAB 中,进行进一步的处理或分析。
### 回答3:
在matlab中读取Excel多个sheet需要使用到"xlsread"函数。"xlsread"函数允许用户读取Excel文件的多个sheet,从而使得用户能够使用matlab处理多个表格数据。
以下是实现方法:
1. 确保已经安装了Microsoft Office Excel软件或者Excel阅读器。
2. 使用matlab打开需要读取的Excel文件。
3. 使用以下命令来读取特定的sheet中的单元格数据。其中,Sheet=’名称’命令用于读取名称为“名称”的sheet中的数据。
[x,y] = xlsread('filename.xlsx','SheetName','Range')
x:将返回Excel表格中的数值区域;
y:将返回Excel表格中的文本区域。
filename.xlsx:需要读取的Excel文件名称;
SheetName:需要读取的Excel文件sheet名称;
Range:需要读取的Excel文件单元格区域。
以下是一个示例:
[x,y] = xlsread('data.xls','Sheet1','A1:B6');
该命令将返回data.xls文件中的Sheet1中的A1到B6之间的单元格。(即文本区域x和数值区域y)。
可以使用循环语句(如for循环)来读取多个sheet中的数据。例如:
for sheet = 1:4
[data, text] = xlsread('data.xls', sheet, 'A1:G10');
% 在这里写代码用于处理data和text
end
这将读取数据的data和text从前4个sheet(sheet1到sheet4),并在每个循环中处理数据。
总之,对于需要读取Excel文件中的多个sheet的用户而言,matlab提供了一种快速有效的方式来读取并处理这些数据。以上是一些基本信息和示例,仅作为参考,具体还需根据实际情况进行调整。
matlab 读取excel有多少个sheet
在 MATLAB 中,可以使用 `xlsfinfo` 函数来读取 Excel 文件中的所有 sheet 数量。下面是一个示例代码:
```matlab
filename = 'example.xlsx'; % Excel 文件名
info = xlsfinfo(filename); % 获取 Excel 文件信息
numSheets = numel(info); % 获取 sheet 数量
disp(['Excel 文件中包含 ', num2str(numSheets), ' 个 sheet。']);
```
请将 `example.xlsx` 替换为你要读取的 Excel 文件名。执行上述代码后,会在命令窗口显示 Excel 文件中 sheet 的数量。
相关推荐
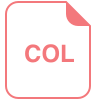
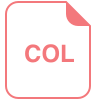
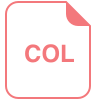
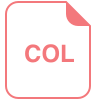
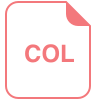







