Python保存矢量文件
时间: 2023-11-05 16:40:04 浏览: 40
在Python中保存矢量文件,你可以使用第三方库如matplotlib和Pillow来实现。下面是保存矢量文件的示例代码:
使用保存矢量文件(如SVG或PDF)```python
import matplotlib.pyplot as plt
# 创建一个图形对象
fig, ax = plt.subplots()
# 绘制你的图形
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# 保存为SVG文件
plt.savefig('output.svg', format='svg')
# 保存为PDF文件
plt.savefig('output.pdf', format='pdf')
```
使用Pillow保存矢量文件(如EPS):
```python
from PIL import Image, ImageDraw
# 创建一个图像对象
image = Image.new('RGB', (200, 200), (255, 255, 255))
draw = ImageDraw.Draw(image)
# 绘制你的矢量图形
draw.rectangle((50, 50, 150, 150), fill=(255, 0, 0))
# 保存为EPS文件
image.save('output.eps', format='eps')
```
这些示例代码将创建一个简单的图形,并将其保存为矢量文件。你可以根据需要调整图形和文件格式。
相关问题
python合并多个矢量文件
要合并多个矢量文件,我们可以使用Python中的GDAL(地理数据抽象库)来实现。GDAL是一个功能强大的开源地理数据处理库,可以处理各种格式的矢量数据。
首先,我们需要安装GDAL库。可以通过在命令行中运行"pip install gdal"来安装。
接下来,我们可以使用以下代码来合并多个矢量文件:
```
from osgeo import ogr
# 创建输出文件
output_file = "merged.shp"
driver = ogr.GetDriverByName("ESRI Shapefile")
output_ds = driver.CreateDataSource(output_file)
output_layer = output_ds.CreateLayer("merged", geom_type=ogr.wkbPolygon)
# 读取要合并的矢量文件列表
input_files = ["file1.shp", "file2.shp", "file3.shp"]
# 遍历每个输入文件
for input_file in input_files:
input_ds = ogr.Open(input_file)
input_layer = input_ds.GetLayer()
# 获取输入图层中的要素
for feature in input_layer:
# 创建新要素
output_feature = ogr.Feature(output_layer.GetLayerDefn())
output_feature.SetGeometry(feature.GetGeometryRef())
# 将新要素添加到输出图层
output_layer.CreateFeature(output_feature)
# 关闭输入数据源
input_ds = None
# 保存并关闭输出数据源
output_ds = None
print("矢量文件已成功合并为", output_file)
```
在上面的代码中,我们首先创建了一个输出数据源(Shapefile格式),然后遍历要合并的每个输入文件。对于每个输入文件,我们打开它,获取图层中的要素,并将每个要素添加到输出图层中。最后,我们保存并关闭输出数据源。
这样,我们就成功地将多个矢量文件合并为一个文件"merged.shp"。
python+矢量数据融合代码
当涉及到Python和矢量数据融合时,通常会使用一些库和工具来处理和分析地理空间数据。以下是一个简单的示例代码,展示了如何使用Python中的geopandas库进行矢量数据融合:
```python
import geopandas as gpd
# 读取待融合的矢量数据
data1 = gpd.read_file('data1.shp')
data2 = gpd.read_file('data2.shp')
# 进行空间融合
merged_data = gpd.overlay(data1, data2, how='union')
# 保存融合后的数据
merged_data.to_file('merged_data.shp')
```
上述代码中,首先使用`geopandas`库导入待融合的矢量数据,可以通过`read_file`函数读取`.shp`文件。然后,使用`overlay`函数对两个矢量数据进行空间融合,其中`how`参数指定了融合的方式,这里使用了`union`表示取并集。最后,使用`to_file`函数将融合后的数据保存为新的`.shp`文件。
请注意,上述代码仅为示例,实际应用中可能需要根据具体需求进行适当的修改和调整。
相关推荐
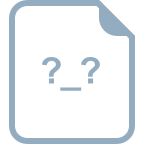
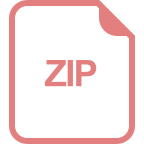
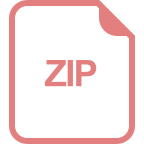












