将OBJECT 列转为日期格式
时间: 2023-09-01 09:10:50 浏览: 130
要将一个 Pandas DataFrame 的某个列从 `object` 类型转换为日期格式,可以使用 `pandas.to_datetime()` 方法。
具体实现步骤如下:
1. 读入数据,生成一个 DataFrame;
2. 使用 `pandas.to_datetime()` 方法将需要转换格式的列转换为日期格式。
示例代码如下:
```python
import pandas as pd
# 生成示例数据
df = pd.DataFrame({'date': ['2022-01-01', '2022-01-02', '2022-01-03'],
'value': [1, 2, 3]})
# 查看数据类型
print(df.dtypes)
# 将 'date' 列转换为日期格式
df['date'] = pd.to_datetime(df['date'])
# 再次查看数据类型
print(df.dtypes)
```
输出结果如下:
```
date object
value int64
dtype: object
date datetime64[ns]
value int64
dtype: object
```
可以看到,`date` 列的数据类型已经从 `object` 转换为了 `datetime64[ns]`。
相关问题
C# 在gridcontrol中将特定列转为标准时间格式yyyy-MM-dd HH:mm:ss
在C#的Windows Forms中,如果你想要在DataGridView GridControl中的特定列转换为日期时间格式"yyyy-MM-dd HH:mm:ss",你可以通过以下步骤操作:
1. 首先,确保你的 DataGridView 已经包含了 DateTime 类型的数据。如果数据不是 DateTime,你需要将其转换成 DateTime。
2. 创建一个事件处理器,如 `CellFormatting` 或者自定义一个事件,当单元格值需要显示时触发。
```csharp
private void dataGridView_CellFormatting(object sender, DataGridViewCellFormattingEventArgs e)
{
// 确保只有指定列的数据需要格式化
if (e.ColumnIndex == yourDateTimeColumnIndex) // 替换为实际的列索引
{
// 将单元格的值转换为 DateTime
DateTime value = Convert.ToDateTime(e.Value);
// 设置格式字符串
string format = "yyyy-MM-dd HH:mm:ss";
// 格式化日期并设置新的值
e.Value = value.ToString(format);
}
}
```
3. 如果你想让这个格式化在整个应用程序中都生效,可以在窗体加载时订阅 DataGridView 的 CellFormatting 事件,如下所示:
```csharp
this.dataGridView.CellFormatting += new DataGridViewCellFormattingEventHandler(dataGridView_CellFormatting);
```
别忘了替换 `yourDateTimeColumnIndex` 为你要格式化的列的实际索引。
C# 在gridview1中将特定列转为标准时间格式yyyy-MM-dd HH:mm:ss
在C#中,如果你想要在GridView1中将特定列的数据转换为标准的时间格式"yyyy-MM-dd HH:mm:ss",你可以这样做:
首先,确保你有一个DateTime类型的字段或者数据源支持这种类型。然后,在GridView的RowDataBound事件中处理这个转换。以下是一个简单的示例:
```csharp
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow) // 只对数据行操作
{
DateTime dateValue = Convert.ToDateTime(e.Row.Cells[YourColumnIndex].Text); // 你的列索引位置替换为实际的列号
string formattedDate = dateValue.ToString("yyyy-MM-dd HH:mm:ss"); // 格式化日期
e.Row.Cells[YourColumnIndex].Text = formattedDate; // 更新单元格显示格式化的日期
}
}
```
这里,`YourColumnIndex`应该替换为你想要转换的列的实际索引。注意,如果`Cells`集合为空,可能是因为数据还没有加载完成,你需要确保在绑定数据后再触发该事件。
阅读全文
相关推荐
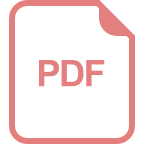
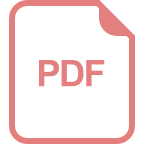
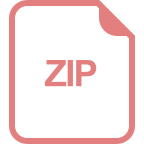
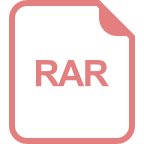

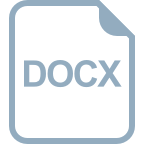
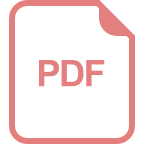
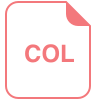







