setup /download config.xml 'setup' 不是内部或外部命令,也不是可运行的程序 或批处理文件。
时间: 2023-11-23 22:57:33 浏览: 154
根据提供的引用内容,执行命令'setup /download config.xml'会提示'setup'不是内部或外部命令,也不是可运行的程序或批处理文件。这是因为'setup'命令并不是系统默认的命令,需要先安装相应的软件或工具才能使用。同时,引用中提到了下载和安装的命令,但并没有明确说明需要安装哪个软件或工具。因此,需要更多的上下文信息才能回答问题。
相关问题
SpringBoot将本地文件传到远程服务器上
### Spring Boot 实现 FTP 和 SFTP 文件上传至远程服务器
#### 使用 FTP 协议上传文件
为了使用 FTP 方式上传文件到远程服务器,在 `application.properties` 中配置 FTP 参数:
```properties
ftp.host=192.168.1.xxx
ftp.port=21
ftp.username=admin
ftp.password=admin@123
ftp.filepath=/remote/path/
```
定义一个工具类用于处理 FTP 连接和操作,例如 `FtpUtil.java`:
```java
public class FtpUtil {
private final String host;
private final int port;
private final String username;
private final String password;
public FtpUtil(String host, int port, String username, String password) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
}
/**
* 检查 FTP 服务器状态.
*/
public boolean checkFtpServerStatus(String server, int port) {
try (FTPClient ftpClient = new FTPClient()) {
ftpClient.connect(server, port);
return FTPReply.isPositiveCompletion(ftpClient.getReplyCode());
} catch (IOException e) {
return false;
}
}
/**
* 执行文件上传功能.
*/
public void uploadFile(File file) throws IOException {
try (FTPClient ftpClient = new FTPClient()) {
ftpClient.connect(host, port);
ftpClient.login(username, password);
InputStream inputStream = new FileInputStream(file);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
ftpClient.storeFile(new File(ftp.filepath).getName(), inputStream);
inputStream.close();
ftpClient.logout();
ftpClient.disconnect();
}
}
}
```
在应用程序入口处测试连接并尝试上传文件[^1]:
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
FtpUtil ftpUtil = new FtpUtil(
"192.168.1.xxx",
21,
"admin",
"admin@123"
);
System.out.println("Checking FTP Server Status...");
if (!ftpUtil.checkFtpServerStatus("192.168.1.xxx", 21)) {
throw new RuntimeException("Failed to connect");
}
System.out.println("Uploading file...");
// 假设有一个名为 test.txt 的文件位于当前目录下
Path pathToFile = Paths.get("test.txt");
try {
ftpUtil.uploadFile(pathToFile.toFile());
System.out.println("Upload completed.");
} catch (IOException ex) {
System.err.println(ex.getMessage());
}
}
}
```
#### 使用 SFTP 协议上传文件
对于基于 SSH 的安全文件传输协议(SFTP),可以利用 JSch 库简化开发过程。
首先引入依赖项(Maven 配置):
```xml
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
```
接着创建控制器和服务层逻辑来支持 RESTful API 请求下的文件上传与下载操作[^3]:
```java
@RestController
@RequestMapping("/api/sftp")
public class SftpController {
@Autowired
private SftpService sftpService;
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile multipartFile,
@RequestParam(value="remotePath", required=false, defaultValue="/") String remotePath) {
try {
sftpService.upload(multipartFile.getInputStream(), remotePath + "/" + multipartFile.getOriginalFilename());
return ResponseEntity.ok().body("Successfully uploaded!");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.toString());
}
}
@GetMapping("/download/{filename}")
public ResponseEntity<Resource> serveFileAsResource(@PathVariable String filename) {
Resource resource = null;
try {
byte[] data = sftpService.download(filename);
resource = new ByteArrayResource(data);
} catch (Exception e) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok()
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() +"\"")
.body(resource);
}
}
@Service
class SftpService {
private Session session;
private ChannelSftp channelSftp;
public SftpService(){
setupConnection();
}
private void setupConnection(){
JSch jsch = new JSch();
try{
session = jsch.getSession("username","hostname",port);
session.setPassword("password");
java.util.Properties config = new java.util.Properties();
config.put("StrictHostKeyChecking", "no");
session.setConfig(config);
session.connect();
Channel channel=session.openChannel("sftp");
channel.connect();
channelSftp=(ChannelSftp)channel;
}catch(Exception e){
e.printStackTrace();
}
}
public void upload(InputStream inputstream,String remotepath)throws Exception{
channelSftp.cd("/");
channelSftp.mkdir(remotepath);
channelSftp.put(inputstream,remotepath);
}
public byte[] download(String filepath)throws Exception{
ByteArrayOutputStream baos=new ByteArrayOutputStream();
channelSftp.get(filepath,baos);
return baos.toByteArray();
}
}
```
阅读全文
相关推荐






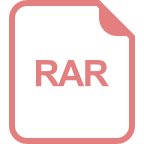









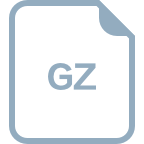