1、建立一个动态链接库(A simple DLL project类型),在其中自行编制1-2个函数。 2、使用DumpBin.exe查看导入导出函数。 3、创建新程序,在新程序中使用隐式或者显式方式调用动态链接库中的函数,输出相关说明
时间: 2023-12-10 19:39:14 浏览: 122
好的,我来给你详细地讲解一下如何完成这个任务。
首先,我们需要创建一个动态链接库项目。在Visual Studio中,我们可以选择创建一个"A simple DLL project"类型的项目。创建完成后,我们会看到一个名为"Dll1"的解决方案和一个名为"Dll1.dll"的动态链接库文件。
接下来,我们需要在动态链接库中编写1-2个函数。这里我以一个简单的加法函数为例,代码如下:
```c++
// Dll1.cpp
#include "pch.h"
#include "framework.h"
extern "C" __declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
```
这里的__declspec(dllexport)关键字表示将该函数导出到动态链接库中。
接下来,我们需要使用DumpBin.exe查看导入导出函数。打开命令提示符窗口,进入到动态链接库所在的目录,然后输入以下命令:
```
dumpbin /exports Dll1.dll
```
执行完毕后,会输出动态链接库中导出的函数信息,如下:
```
Microsoft (R) COFF/PE Dumper Version 14.27.29112.0
Copyright (C) Microsoft Corporation. All rights reserved.
Dump of file Dll1.dll
File Type: DLL
Section contains the following exports for Dll1.dll
00000000 characteristics
FFFFFFFF time date stamp
1.00 version
1 ordinal base
1 number of functions
1 number of names
ordinal hint RVA name
1 0 00001010 Add
Summary
1000 .data
1000 .pdata
1000 .rdata
1000 .reloc
1000 .rsrc
1000 .text
```
我们可以看到,动态链接库中导出了一个名为"Add"的函数。
现在,我们需要创建一个新程序,以便使用刚才创建的动态链接库中的函数。在Visual Studio中,我们可以选择创建一个"Console Application"类型的项目。创建完成后,我们会看到一个名为"ConsoleApplication1"的解决方案和一个名为"ConsoleApplication1.exe"的可执行文件。
接下来,我们需要在新程序中调用动态链接库中的函数。有两种方式可以实现这个目标:隐式链接和显式链接。
隐式链接是指在编译时自动将动态链接库中的函数链接到可执行文件中。我们只需要在新程序中包含动态链接库的头文件,并在链接器选项中添加动态链接库的库文件即可。代码如下:
```c++
// ConsoleApplication1.cpp
#include "pch.h"
#include <iostream>
extern "C" __declspec(dllimport) int Add(int a, int b);
int main()
{
int sum = Add(1, 2);
std::cout << "The sum of 1 and 2 is: " << sum << std::endl;
return 0;
}
```
这里的__declspec(dllimport)关键字表示从动态链接库中导入函数。
显式链接是指在运行时手动加载动态链接库,并使用GetProcAddress函数获取动态链接库中的函数指针。代码如下:
```c++
// ConsoleApplication1.cpp
#include "pch.h"
#include <iostream>
#include <Windows.h>
int main()
{
HMODULE hDll = LoadLibraryA("Dll1.dll");
if (hDll == NULL)
{
std::cerr << "Failed to load DLL: " << GetLastError() << std::endl;
return 1;
}
typedef int(*AddFunc)(int, int);
AddFunc pAdd = (AddFunc)GetProcAddress(hDll, "Add");
if (pAdd == NULL)
{
std::cerr << "Failed to get function pointer: " << GetLastError() << std::endl;
FreeLibrary(hDll);
return 1;
}
int sum = pAdd(1, 2);
std::cout << "The sum of 1 and 2 is: " << sum << std::endl;
FreeLibrary(hDll);
return 0;
}
```
这里的LoadLibraryA函数用于加载动态链接库,GetProcAddress函数用于获取动态链接库中的函数指针。
最后,编译并运行新程序。如果一切正常,控制台会输出"the sum of 1 and 2 is: 3"的信息,表示成功调用了动态链接库中的函数。
阅读全文
相关推荐
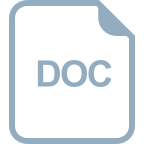
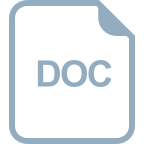
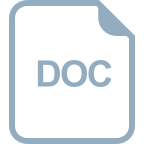

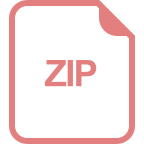
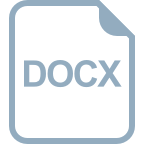
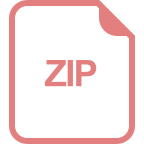
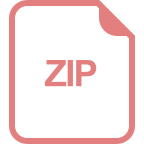
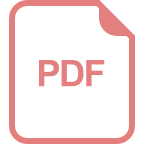
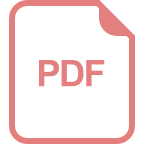
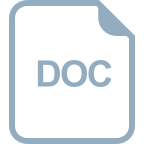
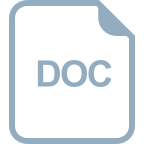
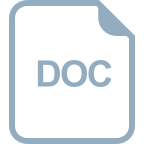
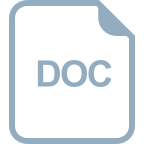
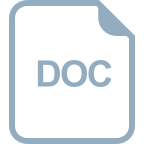



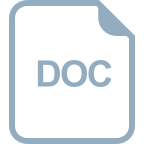