python图像序列补帧
时间: 2023-10-30 08:05:25 浏览: 149
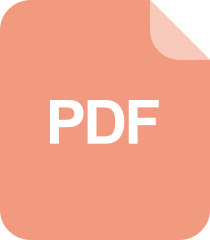
python 实现视频 图像帧提取
在Python中,可以使用OpenCV库对图像序列进行处理。如果要对图像序列进行补帧操作,可以使用以下步骤:
1. 使用OpenCV的cv2.VideoCapture()函数读取图像序列。
2. 使用cv2.VideoWriter()函数创建一个写入器,以便将补帧后的图像序列保存到文件中。
3. 使用循环遍历图像序列中的每一帧。
4. 对于每一帧,使用cv2.copyMakeBorder()函数在图像周围添加黑色边框,以便扩展图像大小。
5. 将扩展后的图像写入输出文件。
下面是一个示例代码,它将图像序列中的每一帧扩展到640x480的大小,并保存为一个新的视频文件:
```
import cv2
# 打开输入视频文件
cap = cv2.VideoCapture('input_video.mp4')
# 获取输入视频的帧率和尺寸
fps = cap.get(cv2.CAP_PROP_FPS)
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# 创建输出视频文件的写入器
out = cv2.VideoWriter('output_video.mp4',
cv2.VideoWriter_fourcc(*'mp4v'),
fps, (640, 480))
# 读取输入视频的每一帧,并对其进行补帧操作
while True:
ret, frame = cap.read()
if not ret:
break
# 在图像周围添加黑色边框,将图像扩展到640x480的大小
border_width = 640 - width
border_height = 480 - height
border_left = border_width // 2
border_right = border_width - border_left
border_top = border_height // 2
border_bottom = border_height - border_top
frame = cv2.copyMakeBorder(frame, border_top, border_bottom, border_left, border_right,
cv2.BORDER_CONSTANT, value=(0, 0, 0))
# 将补帧后的图像写入输出文件
out.write(frame)
# 释放输入和输出视频文件的资源
cap.release()
out.release()
```
阅读全文
相关推荐
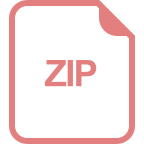
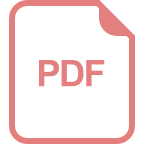







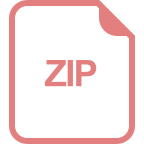
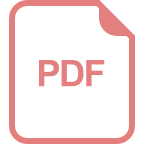
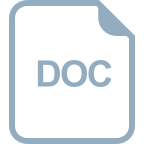
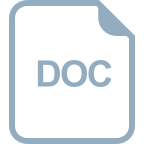
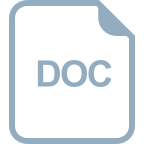
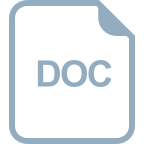
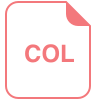

