android蓝牙和线同时播放,Android蓝牙音频播放和录制
时间: 2023-11-27 15:53:39 浏览: 185
Android蓝牙和线同时播放音频是可以实现的,需要使用AudioManager的多媒体音量控制和AudioTrack进行音频播放。
具体实现步骤如下:
1. 获取系统AudioManager实例。
```
AudioManager audioManager = (AudioManager) getSystemService(Context.AUDIO_SERVICE);
```
2. 将多媒体音量设置为最大值。
```
audioManager.setStreamVolume(AudioManager.STREAM_MUSIC, audioManager.getStreamMaxVolume(AudioManager.STREAM_MUSIC), 0);
```
3. 初始化AudioTrack,指定音频采样率、声道数和音频格式。
```
int sampleRate = 44100;
int channelConfig = AudioFormat.CHANNEL_OUT_STEREO;
int audioFormat = AudioFormat.ENCODING_PCM_16BIT;
int bufferSize = AudioTrack.getMinBufferSize(sampleRate, channelConfig, audioFormat);
AudioTrack audioTrack = new AudioTrack(AudioManager.STREAM_MUSIC, sampleRate, channelConfig, audioFormat, bufferSize, AudioTrack.MODE_STREAM);
```
4. 将音频数据写入AudioTrack。
```
audioTrack.write(audioData, 0, audioData.length);
```
5. 启动AudioTrack播放音频。
```
audioTrack.play();
```
同时,如果要实现Android蓝牙音频播放和录制,需要使用BluetoothA2dp和BluetoothHeadsetProfile代表蓝牙A2DP和耳机协议,并使用MediaRecorder进行录制。
具体实现步骤如下:
1. 获取系统BluetoothAdapter实例。
```
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
```
2. 获取蓝牙A2DP和耳机协议的代表实例。
```
BluetoothProfile a2dpProfile = bluetoothAdapter.getProfileProxy(context, new BluetoothProfile.ServiceListener() {
@Override
public void onServiceConnected(int profile, BluetoothProfile proxy) {
if (profile == BluetoothProfile.A2DP) {
a2dpProfile = (BluetoothA2dp) proxy;
}
}
@Override
public void onServiceDisconnected(int profile) {
if (profile == BluetoothProfile.A2DP) {
a2dpProfile = null;
}
}
}, BluetoothProfile.A2DP);
BluetoothProfile headsetProfile = bluetoothAdapter.getProfileProxy(context, new BluetoothProfile.ServiceListener() {
@Override
public void onServiceConnected(int profile, BluetoothProfile proxy) {
if (profile == BluetoothProfile.HEADSET) {
headsetProfile = (BluetoothHeadset) proxy;
}
}
@Override
public void onServiceDisconnected(int profile) {
if (profile == BluetoothProfile.HEADSET) {
headsetProfile = null;
}
}
}, BluetoothProfile.HEADSET);
```
3. 连接蓝牙设备。
```
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
a2dpProfile.connect(device);
headsetProfile.connect(device);
```
4. 使用MediaRecorder进行录制。
```
MediaRecorder mediaRecorder = new MediaRecorder();
mediaRecorder.setAudioSource(MediaRecorder.AudioSource.MIC);
mediaRecorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);
mediaRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB);
mediaRecorder.setOutputFile(outputFile);
mediaRecorder.prepare();
mediaRecorder.start();
```
5. 使用AudioTrack进行播放。
```
int sampleRate = 44100;
int channelConfig = AudioFormat.CHANNEL_OUT_STEREO;
int audioFormat = AudioFormat.ENCODING_PCM_16BIT;
int bufferSize = AudioTrack.getMinBufferSize(sampleRate, channelConfig, audioFormat);
AudioTrack audioTrack = new AudioTrack(AudioManager.STREAM_MUSIC, sampleRate, channelConfig, audioFormat, bufferSize, AudioTrack.MODE_STREAM);
audioTrack.play();
```
阅读全文
相关推荐

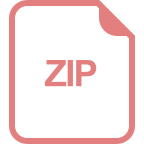
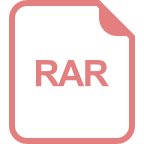
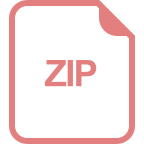
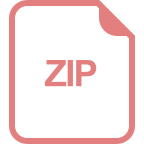
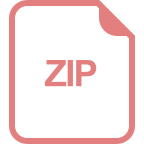
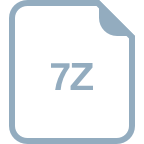
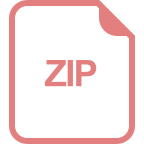
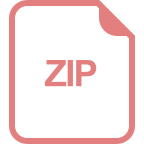
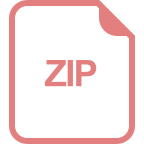
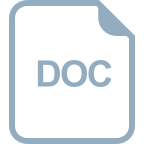
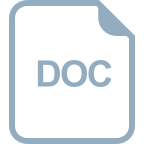
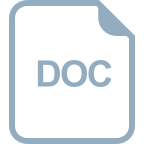


