ttkbootstrap radiobutton 选中
时间: 2023-08-05 19:07:04 浏览: 173
你可以使用 `ttk.Checkbutton` 来创建一个单选按钮,并设置它的状态。以下是一个示例代码:
```python
import tkinter as tk
from tkinter import ttk
def on_select():
print("选中了:" + var.get())
root = tk.Tk()
var = tk.StringVar()
rb1 = ttk.Checkbutton(root, text="选项1", variable=var, value="Option 1", command=on_select)
rb2 = ttk.Checkbutton(root, text="选项2", variable=var, value="Option 2", command=on_select)
rb1.pack()
rb2.pack()
root.mainloop()
```
在这个例子中,我们使用了 `ttk.Checkbutton` 创建了两个单选按钮,每个按钮都有一个不同的值。在 `on_select` 函数中,我们可以使用 `var.get()` 获取当前选中的值。当用户单击任何一个按钮时,该函数将被调用,并将选定的选项打印到控制台中。
相关问题
ttkbootstrap radiobutton 默认选中
要设置 `ttk.Checkbutton` 的默认选中状态,可以在创建 `Checkbutton` 时使用 `variable` 和 `value` 参数来设置默认值。例如,要将第一个单选按钮设置为默认选中状态,可以在 `var` 变量中设置默认值,如下所示:
```python
import tkinter as tk
from tkinter import ttk
def on_select():
print("选中了:" + var.get())
root = tk.Tk()
var = tk.StringVar(value="Option 1") # 设置默认值为 "Option 1"
rb1 = ttk.Checkbutton(root, text="选项1", variable=var, value="Option 1", command=on_select)
rb2 = ttk.Checkbutton(root, text="选项2", variable=var, value="Option 2", command=on_select)
rb1.pack()
rb2.pack()
root.mainloop()
```
在这个例子中,我们将 `var` 变量的默认值设置为 "Option 1",这将使第一个单选按钮默认选中。
属性 radiobutton 选中改变字体大小,android radiobutton选中字体颜色改变的方法,Android radiobutton 选中字体加粗
1. 改变字体大小
可以通过在 `onCheckedChanged` 方法中设置 `TextView` 的字体大小来实现。具体步骤如下:
1. 在布局文件中添加一个 `RadioButton` 和一个 `TextView`。
```xml
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:id="@+id/radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="RadioButton"/>
</RadioGroup>
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hello World!"/>
```
2. 在 Activity 中获取 `RadioButton` 和 `TextView` 的实例,并设置 `onCheckedChangeListener`。
```java
RadioButton radioButton = findViewById(R.id.radio_button);
TextView textView = findViewById(R.id.text_view);
radioButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
textView.setTextSize(24);
} else {
textView.setTextSize(16);
}
}
});
```
当 `RadioButton` 被选中时,设置 `TextView` 的字体大小为 24sp;当 `RadioButton` 没有被选中时,设置 `TextView` 的字体大小为 16sp。
2. 改变字体颜色
可以通过在 `onCheckedChanged` 方法中设置 `TextView` 的字体颜色来实现。具体步骤如下:
1. 在布局文件中添加一个 `RadioButton` 和一个 `TextView`。
```xml
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:id="@+id/radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="RadioButton"/>
</RadioGroup>
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hello World!"/>
```
2. 在 Activity 中获取 `RadioButton` 和 `TextView` 的实例,并设置 `onCheckedChangeListener`。
```java
RadioButton radioButton = findViewById(R.id.radio_button);
TextView textView = findViewById(R.id.text_view);
radioButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
textView.setTextColor(Color.RED);
} else {
textView.setTextColor(Color.BLACK);
}
}
});
```
当 `RadioButton` 被选中时,设置 `TextView` 的字体颜色为红色;当 `RadioButton` 没有被选中时,设置 `TextView` 的字体颜色为黑色。
3. 改变字体加粗
可以通过在 `onCheckedChanged` 方法中设置 `TextView` 的字体样式来实现。具体步骤如下:
1. 在布局文件中添加一个 `RadioButton` 和一个 `TextView`。
```xml
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:id="@+id/radio_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="RadioButton"/>
</RadioGroup>
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hello World!"/>
```
2. 在 Activity 中获取 `RadioButton` 和 `TextView` 的实例,并设置 `onCheckedChangeListener`。
```java
RadioButton radioButton = findViewById(R.id.radio_button);
TextView textView = findViewById(R.id.text_view);
radioButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
textView.setTypeface(Typeface.defaultFromStyle(Typeface.BOLD));
} else {
textView.setTypeface(Typeface.defaultFromStyle(Typeface.NORMAL));
}
}
});
```
当 `RadioButton` 被选中时,设置 `TextView` 的字体样式为加粗;当 `RadioButton` 没有被选中时,设置 `TextView` 的字体样式为正常。
阅读全文
相关推荐
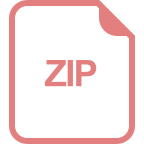
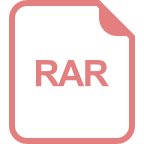
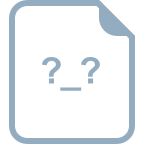
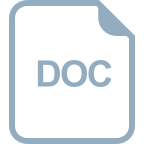











