机器学习神经网络分析面板数据的代码
时间: 2023-06-25 21:01:19 浏览: 66
这是一个基于Python的示例代码,使用了TensorFlow和Keras库。假设你已经有了一些用于训练和测试神经网络的数据集,并且已经建立了一个适当的神经网络模型。
首先,导入所需的库和模块:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
from tensorflow.keras.layers.experimental import preprocessing
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
```
接下来,加载和准备数据集。假设你已经有了一个CSV文件,其中包含用于训练和测试神经网络的数据。使用Pandas库将数据加载到一个DataFrame中:
```python
dataframe = pd.read_csv("data.csv")
```
然后,将DataFrame中的数据分成训练集和测试集:
```python
train_dataset = dataframe.sample(frac=0.8, random_state=0)
test_dataset = dataframe.drop(train_dataset.index)
```
接下来,将数据集转换为张量(Tensor)格式:
```python
train_features = train_dataset.copy()
test_features = test_dataset.copy()
train_labels = train_features.pop('label')
test_labels = test_features.pop('label')
train_features = np.array(train_features)
test_features = np.array(test_features)
train_labels = np.array(train_labels)
test_labels = np.array(test_labels)
```
然后,对数据进行标准化处理:
```python
normalizer = preprocessing.Normalization()
normalizer.adapt(train_features)
train_features = normalizer(train_features)
test_features = normalizer(test_features)
```
接下来,建立神经网络模型:
```python
model = keras.Sequential([
layers.Dense(64, activation='relu', input_shape=[len(train_features[0])]),
layers.Dense(64, activation='relu'),
layers.Dense(1)
])
model.compile(
optimizer=tf.keras.optimizers.Adam(0.001),
loss='mse',
metrics=['mae', 'mse']
)
```
然后,训练神经网络模型:
```python
history = model.fit(
train_features, train_labels,
validation_split=0.2,
verbose=0, epochs=100)
```
最后,使用Matplotlib库绘制训练和验证的损失和精度曲线:
```python
plt.plot(history.history['mse'], label='mse')
plt.plot(history.history['val_mse'], label='val_mse')
plt.xlabel('Epoch')
plt.ylabel('mse')
plt.legend()
plt.show()
```
以上是一个简单的示例代码,可以帮助你开始分析神经网络的性能。当然,具体实现还需要根据你的数据集和任务需求进行调整和优化。
相关推荐
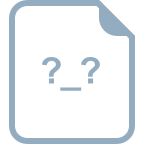














