hutool 解析excel批注
时间: 2023-07-07 22:30:21 浏览: 252
使用Hutool解析Excel批注的步骤如下:
1. 导入Hutool的Excel读取依赖:
```xml
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-poi</artifactId>
<version>5.7.10</version>
</dependency>
```
2. 创建ExcelReader对象,读取Excel文件:
```java
ExcelReader reader = ExcelUtil.getReader("test.xlsx");
```
3. 获取Excel中的批注信息:
```java
// 获取第1个sheet的第1行第1列的批注
Comment comment = reader.getComment(0, 0, 0);
if (comment != null) {
String author = comment.getAuthor(); // 获取批注作者
String text = comment.getText(); // 获取批注内容
}
```
通过以上步骤,就可以使用Hutool解析Excel批注了。
相关问题
Java 解析excel批注
Java 可以使用 Apache POI 库来解析和操作 Excel 文件。要解析批注,可以使用以下代码:
```java
import org.apache.poi.ss.usermodel.Comment;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import java.io.FileInputStream;
public class ExcelParser {
public static void main(String[] args) throws Exception {
// 读取 Excel 文件
FileInputStream fis = new FileInputStream("example.xlsx");
Workbook workbook = WorkbookFactory.create(fis);
// 获取第一个 Sheet
Sheet sheet = workbook.getSheetAt(0);
// 遍历 Sheet 中的每个单元格
for (int rowIndex = 0; rowIndex <= sheet.getLastRowNum(); rowIndex++) {
for (int columnIndex = 0; columnIndex < sheet.getRow(rowIndex).getLastCellNum(); columnIndex++) {
// 获取单元格的批注
Comment comment = sheet.getCellComment(rowIndex, columnIndex);
if (comment != null) {
String author = comment.getAuthor();
String text = comment.getString().getString();
System.out.println("批注作者:" + author);
System.out.println("批注内容:" + text);
}
}
}
// 关闭文件流
fis.close();
}
}
```
其中,`getCellComment(rowIndex, columnIndex)` 方法可以获取指定单元格的批注对象。如果该单元格没有批注,则返回 `null`。使用 `getAuthor()` 方法可以获取批注的作者,使用 `getString().getString()` 方法可以获取批注的内容。
Java 解析excel及批注
可以使用 Apache POI 库来解析 Excel 文件以及批注。以下是一个简单的示例代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Comment;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelParser {
public static void main(String[] args) {
try (InputStream inp = new FileInputStream("example.xlsx")) {
Workbook wb = WorkbookFactory.create(inp);
Sheet sheet = wb.getSheetAt(0);
// 遍历每一行
for (Row row : sheet) {
// 遍历每一列
for (Cell cell : row) {
// 获取单元格的值
String value = cell.getStringCellValue();
System.out.print(value);
// 获取单元格的批注
Comment comment = cell.getCellComment();
if (comment != null) {
System.out.print(" (注: " + comment.getString().getString() + ")");
}
System.out.print("\t");
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在此示例中,我们使用 `WorkbookFactory.create` 方法来打开 Excel 文件,并使用 `Sheet` 和 `Row` 对象来遍历每个单元格。使用 `Cell` 对象来获取单元格的值,并使用 `Comment` 对象来获取单元格的批注。注意,使用前需要先检查单元格是否有批注。
阅读全文
相关推荐
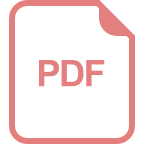
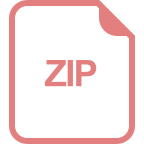
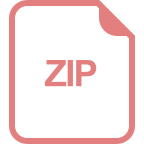
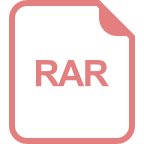
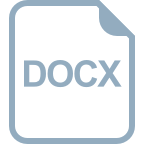
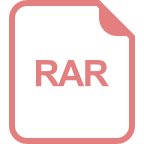
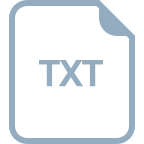
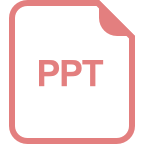
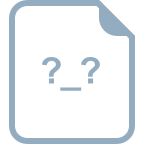
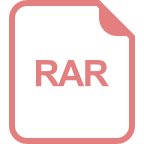
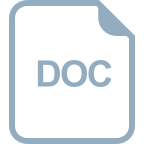
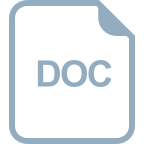
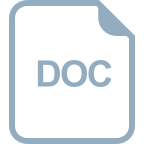
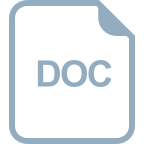
