Swagger的授权配置帮我用java写出来
时间: 2024-05-28 20:09:57 浏览: 46
当您使用Swagger来为您的API文档生成器时,您需要进行授权配置。这可以通过使用Spring Security实现。以下是一个简单的示例代码:
@Configuration
@EnableSwagger2
@EnableWebMvcSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().antMatchers("/swagger-ui.html").authenticated();
http.httpBasic();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication().withUser("username").password("password").roles("USER");
}
}
这将限制访问Swagger UI并将其保护在需要身份验证的URL之后。您可以使用Spring Security提供的默认登录表单进行身份验证,或使用自定义身份验证机制以适应您的应用程序的特定需求。
相关问题
java 防止swagger未授权访问
在Java中,使用Swagger作为API文档工具时,为了防止未经授权的访问,你可以采取以下几个步骤:
1. **设置认证机制**:可以集成如OAuth2、JWT(JSON Web Tokens)等身份验证方案。Spring Security或Dropwizard等框架提供了一整套支持,确保只有经过验证的用户才能访问API。
```java
@Autowired
private AuthenticationManager authenticationManager;
@Bean
public SecurityFilterChain httpBasicSecurityFilterChain(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/**").authenticated() // 只允许已授权的路径
.and()
.httpBasic();
return http.build();
}
```
2. **配置Swagger安全拦截器**:在Swagger的配置文件中,添加对特定API的访问限制。例如,在`application.yml`或`application.properties`中:
```yaml
swagger:
securityDefinitions:
apiKey:
type: apiKey
name: Authorization
in: header
security:
- apiKey: []
apis:
- path: /api/secure
security:
- apiKey: []
```
3. **使用Swagger UI Secure**:Swagger UI Secure插件可以帮助你限制Swagger UI的访问,只允许已经通过认证的用户查看API文档。
4. **代码层面检查**:在处理请求之前,可以在控制器方法上添加检查,确保当前用户有权限访问该资源。
```java
@RestController
@PreAuthorize("hasRole('ADMIN')")
@RequestMapping("/admin")
public class AdminController { ... }
```
java 如何禁掉/v2/api-docs 防止swagger未授权访问
在Spring Boot项目中,如果你想要禁用Swagger(通常是基于`springfox`库的API文档功能)的`/v2/api-docs`端点以防止未经授权的访问,可以采取以下步骤:
1. **移除Swagger依赖**:
如果不再需要Swagger,可以直接从pom.xml或build.gradle文件中删除相关的 Swagger依赖。例如,在Maven中:
```xml
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>版本号</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>版本号</version>
</dependency>
```
将这两个依赖项注释掉或者直接删掉。
2. **禁用Swagger配置**:
如果你仍然希望保留其他功能但关闭`/v2/api-docs`,可以在Spring配置类中禁用Swagger的相关配置。比如使用`@EnableSwagger2`注解:
```java
@EnableSwagger2(basePackages = "com.example.api", // 包含API的包路径)
public class SwaggerConfig {
// 可以将接下来的@Configuration和@EnableSwagger2WebMvc注解移除
}
```
3. **配置Security**:
添加Spring Security配置,限制对特定资源(如`/v2/api-docs`)的访问。例如,使用`@PreAuthorize`注解:
```java
@GetMapping("/v2/api-docs")
@PreAuthorize("hasRole('ADMIN')")
public String apiDocumentation() {
return "Your custom documentation page";
}
```
或者,你可以设置Spring Security的访问规则来阻止未授权用户访问。
4. **部署隐藏**:
在生产环境中,你还可以通过服务器配置将`/v2/api-docs`隐藏起来,例如在Apache或Nginx的反向代理设置中。
记住,在实施这些更改之前,一定要确认这不会影响到你的应用程序其他正常的功能需求。
阅读全文
相关推荐
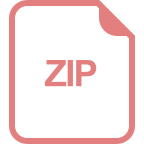
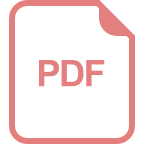
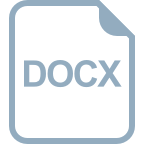













