一个好的程序要有一定比例的注释。编写一个程序统计一个C源文件中注释所占的百分比。百分比计算公式为:程序注释中字符总数(/*和*/除外的所有字符)除以程序文件中总字符数(程序文件中的所有字符)。 注:只简单考虑/*...*/的注释,而且要考虑注释跨行的情况,不要考虑其它复杂情况。
时间: 2024-02-25 10:55:24 浏览: 73
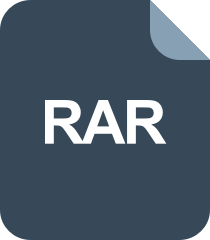
less语法小结-源代码+注释.rar
以下是Java代码实现:
```java
import java.io.*;
public class CountComments {
public static void main(String[] args) {
String fileName = "test.c";
// 读取文件内容
String content = readFile(fileName);
// 统计注释所占百分比
double commentRatio = countCommentRatio(content);
System.out.println("注释所占百分比:" + commentRatio * 100 + "%");
}
/**
* 读取文件内容
*/
private static String readFile(String fileName) {
StringBuilder sb = new StringBuilder();
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
} catch (IOException e) {
e.printStackTrace();
}
return sb.toString();
}
/**
* 统计注释所占百分比
*/
private static double countCommentRatio(String content) {
int commentLength = 0;
int totalLength = 0;
boolean inComment = false;
for (int i = 0; i < content.length(); i++) {
char c = content.charAt(i);
if (inComment) {
if (c == '*' && i < content.length() - 1 && content.charAt(i + 1) == '/') {
inComment = false;
i++;
} else {
commentLength++;
}
} else {
if (c == '/' && i < content.length() - 1 && content.charAt(i + 1) == '*') {
inComment = true;
i++;
} else if (c != '\n' && c != '\r' && c != ' ') {
totalLength++;
}
}
}
return (double) commentLength / totalLength;
}
}
```
代码思路:
1. 首先读取指定文件中的C源文件内容。
2. 遍历源文件中的每个字符,统计注释和总字符数。
3. 用注释长度除以总字符数,得到注释所占的百分比。
注意事项:
1. 注释可能跨行,需要特殊处理。
2. 在统计字符数时,需要排除换行符、回车符和空格等无关字符。
3. 在try-with-resources语句中使用BufferedReader类,可以避免手动关闭文件流,代码更简洁。
阅读全文
相关推荐
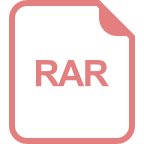
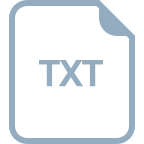
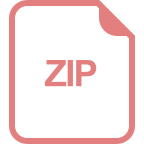
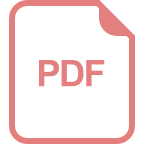
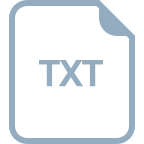
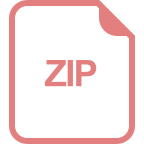
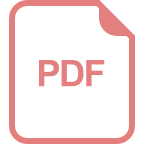
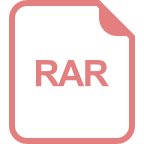
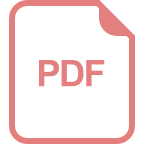
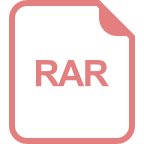
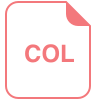
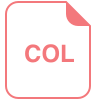
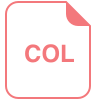
