SpringBoot后端从Local storage中获取信息
时间: 2024-09-27 22:13:29 浏览: 38
Spring Boot 后端应用通常通过文件存储(如 Local Storage)读取数据时,会利用Java的标准库或者第三方库,例如`java.nio.file`包提供的功能来操作本地文件。以下是一个基本步骤:
1. **添加依赖**:如果项目中尚未集成,需要在Spring Boot的pom.xml或build.gradle文件中添加对文件操作的支持,比如Apache Commons IO 或者 Java NIO。
```xml
<!-- Maven -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
</dependency>
<!-- Gradle -->
implementation 'commons-io:commons-io'
```
2. **访问文件**:使用`File`或`Path`类来定位文件,比如通过相对路径或绝对路径。
```java
Path filePath = Paths.get("src/main/resources/data.txt"); // 使用默认工作目录作为基础
File file = new File(filePath.toFile()); // 如果需要File对象
```
3. **读取内容**:可以使用`Files.readAllLines()`、`Files.readFile()`等方法来读取文件的内容,如果是文本文件。
```java
List<String> lines = Files.readAllLines(file.toPath());
String content = new String(Files.readAllBytes(file.toPath()));
```
4. **处理错误**:记得检查文件是否存在、是否可读等异常情况。
5. **将数据注入到服务中**:读取的数据可以根据需求转换为模型对象,并注入到业务层的服务中供其他组件使用。
```java
@Service
public class DataService {
private final ObjectMapper objectMapper;
public DataService(ObjectMapper objectMapper) {
this.objectMapper = objectMapper;
}
public YourModelClass readDataFromLocalStorage() {
try {
String jsonContent = Files.readString(file.toPath());
return objectMapper.readValue(jsonContent, YourModelClass.class);
} catch (IOException e) {
log.error("Failed to read data from local storage", e);
throw new RuntimeException(e);
}
}
}
```
阅读全文
相关推荐
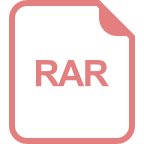
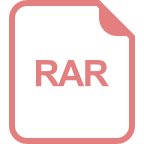
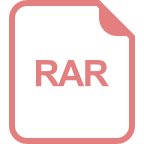
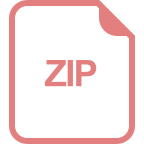
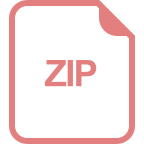
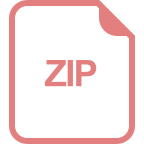
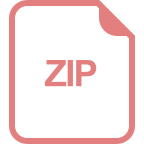
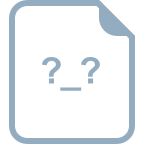
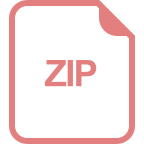
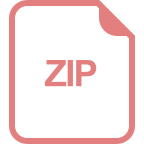
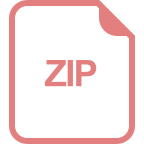
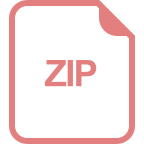
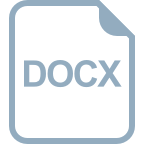
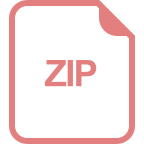
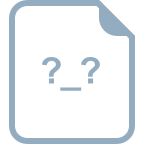
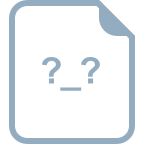
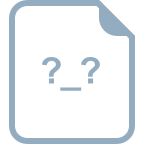
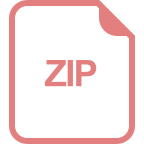
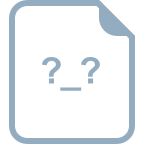