java读xlsx文件取出相同字段的行
时间: 2024-05-08 16:18:13 浏览: 7
可以使用Apache POI库来读取xlsx文件,然后使用Java代码来筛选出相同字段的行。以下是一个简单的示例:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ReadXLSXFile {
public static void main(String[] args) {
try {
File file = new File("example.xlsx");
FileInputStream fis = new FileInputStream(file);
XSSFWorkbook workbook = new XSSFWorkbook(fis);
XSSFSheet sheet = workbook.getSheetAt(0);
String searchValue = "example";
for (Row row : sheet) {
Cell cell = row.getCell(0); // assuming the field is in the first cell
if (cell != null && cell.getStringCellValue().equals(searchValue)) {
// do something with the matching row
System.out.println("Found a row with the value: " + searchValue);
break; // assuming there is only one matching row
}
}
workbook.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码会打开名为"example.xlsx"的文件,并在第一个工作表中查找第一列中值为"example"的行。如果找到了匹配的行,则输出一条消息,否则什么也不做。你可以根据自己的需求修改这个示例代码。
相关推荐
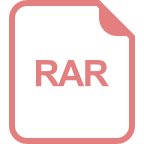














