请仔细阅读右侧代码,根据方法内的提示,在begin - end区域内进行代码补充,具体任务如下: 使用继承thread类的方式创建一个名为 threadclassone 的类,重写的run方法需要实现输出0-10之间的奇数,输出结果如下: 1 3 5 7 9; 使用实现runnable接口的方式创建一个名为threadclasstwo的类,重写run方法,编写start方法,run方法需要实现打印0-10之间的偶数,输出结果如下: 0 2 4 6 8 10
时间: 2023-05-31 11:17:55 浏览: 510
### 回答1:
这段代码需要仔细阅读,根据方法内的提示,在 begin - end 区域内进行代码补充,具体任务如下:
1. 使用继承 thread 类的方式创建一个名为 threadclassone 的类,重写其中的 run 方法实现输出 0-10 之间的奇数,输出结果如下:1 3 5 7 9;
2. 使用实现 runnable 接口的方式创建一个名为 threadclasstwo 的类,重写其中的 run 方法和 start 方法,实现输出 0-10 之间的偶数,并实现 start 方法启动线程,输出结果如下:0 2 4 6 8 10。
### 回答2:
首先需要了解两种方式创建线程的基本概念:
1. 继承 Thread 类
继承 Thread 类并重写其 run 方法,然后通过创建 Thread 类的实例并调用 start 方法来启动线程。
2. 实现 Runnable 接口
实现 Runnable 接口并重写其 run 方法,然后通过创建 Thread 类的实例并将其作为参数传递给 Thread 类的构造函数来创建线程。
现在根据题目要求,我们需要分别创建 threadclassone 和 threadclasstwo 两个类实现不同的线程输出。
1. 创建 threadclassone 类:
```
class threadclassone extends Thread{
public void run(){
for(int i=1;i<=10;i+=2){
System.out.print(i + " ");
}
}
}
```
在 run 方法中,使用 for 循环输出 1-10 之间的奇数。
2. 创建 threadclasstwo 类:
```
class threadclasstwo implements Runnable{
public void run(){
for(int i=0;i<=10;i+=2){
System.out.print(i + " ");
}
}
public void start(){
Thread t = new Thread(this);
t.start();
}
}
```
在 run 方法中,使用 for 循环输出 0-10 之间的偶数,同时还需要重写 start 方法,通过创建 Thread 类的实例并传入当前对象来启动线程。
现在可以通过创建 threadclassone 和 threadclasstwo 两个类的实例来启动线程并输出结果:
```
public class Main {
public static void main(String[] args) {
threadclassone t1 = new threadclassone();
t1.start();
threadclasstwo t2 = new threadclasstwo();
t2.start();
}
}
```
以上代码将同时输出:
```
1 3 5 7 9 0 2 4 6 8 10
```
### 回答3:
根据题目要求,需要编写两个类,分别是threadclassone和threadclasstwo,实现输出0-10之间的奇数和偶数。
首先,我们来实现threadclassone类。该类需要继承自thread类,并重写run方法,在该方法中实现输出0-10之间的奇数。具体代码如下:
```
public class threadclassone extends Thread {
public void run() {
for (int i = 1; i <= 10; i += 2) {
System.out.print(i + " ");
}
}
}
```
上面的代码中,我们使用for循环来遍历1-10之间的奇数,每次输出一个奇数。由于该类继承了thread类并重写了run方法,因此可以直接调用start方法来启动该线程,从而实现输出0-10之间的奇数。如下所示:
```
public static void main(String[] args) {
threadclassone t1 = new threadclassone();
t1.start();
}
```
接下来我们来实现threadclasstwo类。该类需要实现runnable接口,并重写run方法,在该方法中实现输出0-10之间的偶数。我们还需要编写start方法,通过start方法启动该线程。具体代码如下:
```
public class threadclasstwo implements Runnable {
public void run() {
for (int i = 0; i <= 10; i += 2) {
System.out.print(i + " ");
}
}
public void start() {
Thread t = new Thread(this);
t.start();
}
}
```
上面的代码中,我们通过实现runnable接口,重写run方法来实现输出0-10之间的偶数。在start方法中,我们创建了一个新的线程对象,并将该对象的运行目标设置为当前类的实例,然后启动该线程。这样便可以通过调用该类的start方法来启动线程。如下所示:
```
public static void main(String[] args) {
threadclasstwo t2 = new threadclasstwo();
t2.start();
}
```
以上便是题目所要求的代码实现。通过继承thread类和实现runnable接口的方式分别创建两个线程类,实现输出0-10之间的奇数和偶数。我们可以在main方法中分别创建这两个线程的对象,并通过调用start方法来启动线程,从而实现输出要求的结果。
相关推荐
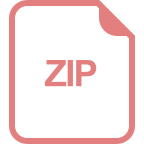
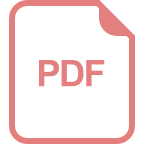
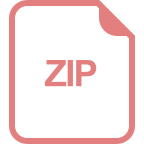














