vue3移动端蓝牙通信
时间: 2023-09-15 12:19:05 浏览: 244
对于Vue 3移动端蓝牙通信,你可以使用Web Bluetooth API来实现。下面是一个简单的示例代码:
1. 首先,在你的Vue项目中安装 `web-bluetooth` 包:
```shell
npm install web-bluetooth
```
2. 创建一个蓝牙通信的Vue组件,比如 `BluetoothDevice.vue`:
```html
<template>
<div>
<button @click="connectToDevice">连接设备</button>
<button @click="disconnectFromDevice">断开连接</button>
<button @click="sendData">发送数据</button>
</div>
</template>
<script>
import * as WebBluetooth from 'web-bluetooth';
export default {
data() {
return {
device: null,
characteristic: null,
};
},
methods: {
async connectToDevice() {
try {
// 请求蓝牙设备
const device = await WebBluetooth.requestDevice({
filters: [{ services: ['heart_rate'] }],
});
// 连接蓝牙设备
await device.gatt.connect();
// 获取蓝牙设备的服务和特征值
const service = await device.gatt.getPrimaryService('heart_rate');
const characteristic = await service.getCharacteristic('heart_rate_measurement');
// 设置数据接收的回调函数
characteristic.addEventListener('characteristicvaluechanged', this.handleData);
// 启动数据接收
await characteristic.startNotifications();
this.device = device;
this.characteristic = characteristic;
} catch(error) {
console.error('连接蓝牙设备错误:', error);
}
},
async disconnectFromDevice() {
if (this.device && this.device.gatt.connected) {
await this.device.gatt.disconnect();
this.device = null;
this.characteristic = null;
}
},
async sendData() {
if (this.device && this.characteristic) {
const encoder = new TextEncoder();
const data = encoder.encode('Hello, Bluetooth!');
await this.characteristic.writeValue(data);
}
},
handleData(event) {
const value = event.target.value;
// 处理接收到的数据
console.log('接收到的数据:', value);
},
},
};
</script>
```
3. 在你的Vue页面中使用该组件:
```html
<template>
<div>
<bluetooth-device></bluetooth-device>
</div>
</template>
<script>
import BluetoothDevice from './BluetoothDevice.vue';
export default {
components: {
BluetoothDevice,
},
};
</script>
```
注意:上述代码仅为示例,实际使用时需要根据具体的蓝牙设备和特征值进行相应的配置和处理。另外,为了能在移动端使用Web Bluetooth API,需要在安全的上下文环境中(HTTPS、localhost)运行。
阅读全文
相关推荐
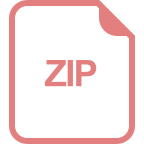
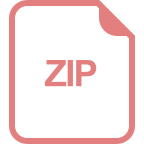
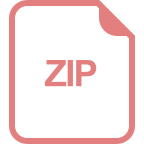
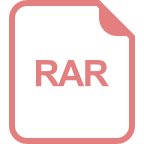
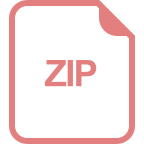
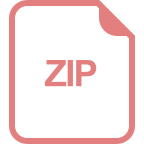
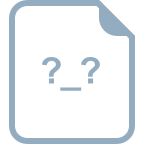
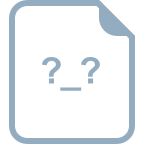
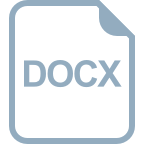
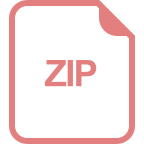
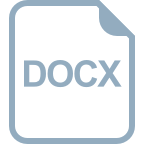
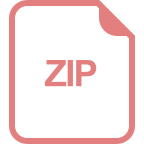