for i in range(10): imgL = cv2.imread('left{i}.jpg') imgR = cv2.imread('right{i}.jpg')
时间: 2024-05-24 21:10:40 浏览: 13
This code snippet reads 10 pairs of images named 'left{i}.jpg' and 'right{i}.jpg', where i is a variable that takes on values from 0 to 9 in the range function. The images are read into imgL and imgR variables respectively using the OpenCV library in Python.
相关问题
解释for i in range(10): imgL = cv2.imread('left{i}.jpg') imgR = cv2.imread('right{i}.jpg')
这段代码使用了Python的for循环语句以及OpenCV库中的imread函数。
for i in range(10)语句表示循环10次,每次循环i的值从0到9递增。
在循环内部,imgL = cv2.imread('left{i}.jpg') 表示读取名为"left{i}.jpg"的图像文件,并将其赋值给变量imgL。其中{i}表示i的值将会被插入到字符串中,以便读取不同的图像文件。
同样的,imgR = cv2.imread('right{i}.jpg') 表示读取名为"right{i}.jpg"的图像文件,并将其赋值给变量imgR。
因此,这段代码的作用是读取名为"left0.jpg"到"left9.jpg"和"right0.jpg"到"right9.jpg"的图像文件,分别存储在imgL和imgR变量中。
、3种三维重建方法实现视差估计 python代码
以下是三种三维重建方法的视差估计Python代码示例:
1. 基于SIFT特征匹配的视差估计:
```python
import cv2
import numpy as np
# 读取左右相机的图像
imgL = cv2.imread('left.jpg', 0)
imgR = cv2.imread('right.jpg', 0)
# 创建SIFT对象
sift = cv2.xfeatures2d.SIFT_create()
# 对左右图像提取SIFT特征和描述符
kp1, des1 = sift.detectAndCompute(imgL, None)
kp2, des2 = sift.detectAndCompute(imgR, None)
# 创建暴力匹配器对象
bf = cv2.BFMatcher()
# 对左右图像的SIFT描述符进行匹配
matches = bf.knnMatch(des1, des2, k=2)
# 根据Lowe's ratio test筛选匹配点
good = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good.append(m)
# 计算视差
disparity = np.zeros_like(imgL)
for m in good:
x, y = kp1[m.queryIdx].pt
x1, y1 = kp2[m.trainIdx].pt
disparity[int(y), int(x)] = x - x1
# 显示视差图像
cv2.imshow('disparity', disparity)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 基于BM算法的视差估计:
```python
import cv2
import numpy as np
# 读取左右相机的图像
imgL = cv2.imread('left.jpg', 0)
imgR = cv2.imread('right.jpg', 0)
# 创建立体匹配对象
stereo = cv2.StereoBM_create(numDisparities=16, blockSize=15)
# 计算视差
disparity = stereo.compute(imgL, imgR)
# 显示视差图像
cv2.imshow('disparity', disparity)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 基于SGBM算法的视差估计:
```python
import cv2
import numpy as np
# 读取左右相机的图像
imgL = cv2.imread('left.jpg', 0)
imgR = cv2.imread('right.jpg', 0)
# 创建立体匹配对象
stereo = cv2.StereoSGBM_create(numDisparities=16, blockSize=15)
# 计算视差
disparity = stereo.compute(imgL, imgR)
# 显示视差图像
cv2.imshow('disparity', disparity)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
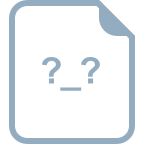
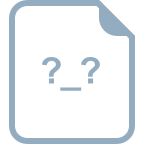
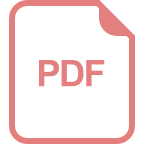












