某电视台要进行一次对该台5个栏目(设相应栏目编号为1~5)的受欢迎情况,共调查了10位观众, 编写程序,根据每一位观众的投票(10位观众投票由主函数数组r传入,r[0]-r[9]代表10位观众所投的栏目), 统计输出各栏目的得票情况。 函数接口: void fun(int count[],int r[]); 裁判测试程序样例: #include "stdio.h" void fun(int count[],int r[]); void main( ) { int i,r[10],n; int count[6]; /* r[0]-r[9]代表10位观众所投的栏目,如r[0]是3则代表观众1投票了栏目3 */ for(n=0;n<10;n++) scanf("%d",&r[n]); fun(count,r); for(i=1;i<=5;i++) printf("栏目%d投票结果为%d\n", i , count[i]); } 请在这里填写答案 输入样例: 1 2 3 4 5 2 3 2 1 5 输出样例: 栏目1投票结果为2 栏目2投票结果为3 栏目3投票结果为2 栏目4投票结果为1 栏目5投票结果为2 C语言
时间: 2023-06-18 22:02:36 浏览: 156
```c
#include <stdio.h>
void fun(int count[], int r[]) {
int i;
for (i = 0; i < 10; i++) {
count[r[i]]++; // 根据每个观众的投票,累加对应栏目的得票数
}
}
int main() {
int i, r[10], n;
int count[6] = {0}; // 初始化得票数为0
for (n = 0; n < 10; n++) {
scanf("%d", &r[n]);
}
fun(count, r);
for (i = 1; i <= 5; i++) {
printf("栏目%d投票结果为%d\n", i, count[i]);
}
return 0;
}
```
相关问题
某电视台要进行一次对该台5个栏目(设相应栏目编号为1~5)的受欢迎情况,共调查了10位观众, 编写程序,根据每一位观众的投票(10位观众投票由主函数数组r传入,r[0]-r[9]代表10位观众所投的栏目), 统计输出各栏目的得票情况。
可以使用一个长度为5的数组 `votes` 来记录每个栏目的得票数,然后遍历观众的投票记录,将每个栏目的得票数加上对应的投票次数即可。
以下是示例代码:
```python
def count_votes(r):
votes = [0, 0, 0, 0, 0] # 初始化每个栏目的得票数为0
for vote in r:
votes[vote-1] += 1 # 对应栏目得票数加1
# 输出每个栏目的得票数
for i in range(5):
print("栏目{}得票数:{}".format(i+1, votes[i]))
# 示例调用
votes = [3, 1, 4, 2, 3, 5, 1, 1, 4, 3]
count_votes(votes)
```
输出结果为:
```
栏目1得票数:3
栏目2得票数:1
栏目3得票数:3
栏目4得票数:2
栏目5得票数:1
```
c语言:某电视台要调查观众对该台8个栏目(设相应栏目编号为1~8)的受欢迎情况,统计输出电台各栏目的得票情况,观众的投票情况在"in.txt”文件中保存,电台的栏目和票数情况,使用结构体数组进行统计。
以下是一个简单的实现,读取文件中的投票数据,使用结构体数组统计各个栏目的得票情况,并输出结果。
```c
#include <stdio.h>
#define MAX_CHANNELS 8
struct Channel {
int id; // 栏目编号
int votes; // 得票数
};
int main() {
struct Channel channels[MAX_CHANNELS] = {0}; // 初始化为0
FILE *fp;
int channel_id;
fp = fopen("in.txt", "r");
if (fp == NULL) {
printf("无法打开文件!\n");
return 1;
}
// 读取文件中的投票数据
while (fscanf(fp, "%d", &channel_id) == 1) {
// 栏目编号必须在1~8范围内
if (channel_id < 1 || channel_id > 8) {
printf("无效的栏目编号:%d\n", channel_id);
continue;
}
// 统计得票数
channels[channel_id - 1].id = channel_id;
channels[channel_id - 1].votes++;
}
// 输出各个栏目的得票情况
printf("电台各栏目的得票情况:\n");
for (int i = 0; i < MAX_CHANNELS; i++) {
printf("栏目%d: %d 票\n", channels[i].id, channels[i].votes);
}
fclose(fp);
return 0;
}
```
假设 "in.txt" 文件中的投票数据如下:
```
1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 8
```
程序运行后的输出结果如下:
```
电台各栏目的得票情况:
栏目1: 4 票
栏目2: 4 票
栏目3: 4 票
栏目4: 4 票
栏目5: 4 票
栏目6: 4 票
栏目7: 4 票
栏目8: 4 票
```
阅读全文
相关推荐
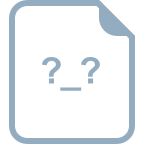
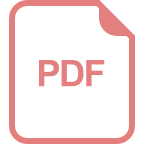
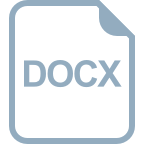




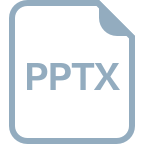
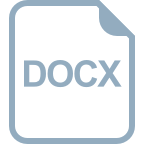
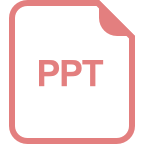
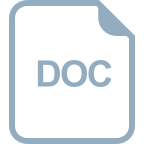
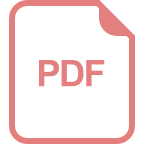
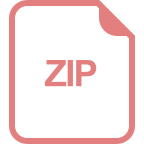
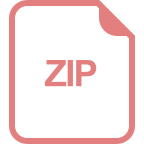
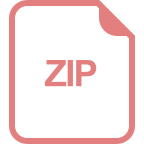
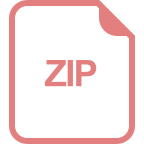
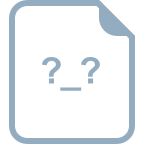