替换文件中指定函数和使用函数时里面某个传参的python脚本
时间: 2024-09-09 10:06:14 浏览: 59
在Python中,你可以使用一些模块如`ast`(Abstract Syntax Trees,抽象语法树)来替换文件中的特定函数和修改函数参数。下面是一个简化的示例步骤:
1. 首先,你需要导入必要的模块:
```python
import ast
from pathlib import Path
```
2. 定义你要替换的函数名和新的实现,以及目标参数的新值(如果需要)。假设我们要替换函数`old_function`并改变参数`arg_name`:
```python
def new_function(old_function_body, arg_name, new_value):
# 替换函数体内容
new_function_code = ast.parse(old_function_body).body[0].body
# 修改参数位置和值
for node in new_function_code:
if isinstance(node, ast.arg) and node.arg == arg_name:
node.value = ast.Constant(new_value)
# 将修改后的代码转换回字符串
new_function_code_str = ast.unparse(new_function_code)
return new_function_code_str
```
3. 然后,读取文件并查找你要替换的函数:
```python
def replace_and_write(file_path, old_function_name, arg_name, new_value):
with open(file_path, 'r') as file:
source_code = file.read()
tree = ast.parse(source_code)
# 查找函数节点
func_node = find_node_by_name(tree, old_function_name)
if func_node is not None:
new_func_code = new_function(func_node.body, arg_name, new_value)
updated_source_code = ast.fix_missing_locations(ast.parse(new_func_code))
# 写入修改后的源代码
with open(file_path, 'w') as file:
file.write(ast.unparse(updated_source_code))
else:
print(f"Function {old_function_name} not found in the file.")
# 辅助函数用于搜索AST中的函数节点
def find_node_by_name(node, name):
if isinstance(node, ast.FunctionDef) and node.name == name:
return node
for child in node.body:
result = find_node_by_name(child, name)
if result is not None:
return result
return None
```
4. 调用这个函数,并提供你要操作的文件路径、函数名称和参数:
```python
replace_and_write('your_file.py', 'old_function', 'arg_name', new_value)
```
请注意,这只是一个基本示例,实际应用中你可能需要处理更复杂的结构,比如嵌套函数、异常处理等。
阅读全文
相关推荐
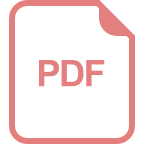
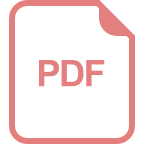
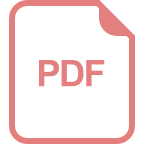
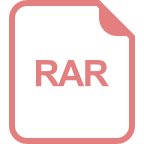
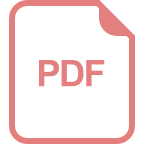
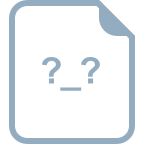
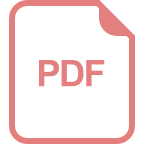
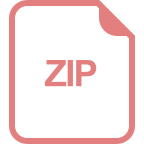
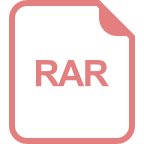
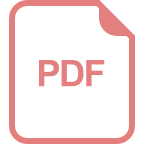
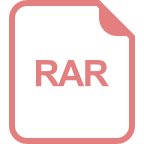
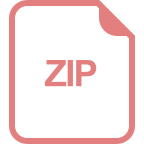