visual studio 2022 c++如何连接mysql
时间: 2024-05-07 19:15:50 浏览: 5
要在Visual Studio 2022中连接MySQL,您需要执行以下步骤:
1. 下载和安装MySQL Connector/C++:您可以从MySQL官方网站下载和安装MySQL Connector/C++。此外,您需要将Connector/C++添加到Visual Studio 2022的“附加依赖项”中。
2. 创建一个新的C++项目:在Visual Studio 2022中,创建一个新的C++项目。
3. 添加MySQL头文件:在项目中添加MySQL的头文件,以便您可以使用MySQL连接函数。
4. 添加MySQL库文件:在项目中添加MySQL的库文件,以便您可以连接到MySQL数据库。
5. 编写连接代码:编写代码以连接到MySQL数据库。您可以使用MySQL的C++ API或ODBC API进行连接。
以下是一个使用C++ API连接到MySQL数据库的示例代码:
```cpp
#include <iostream>
#include <mysql.h>
using namespace std;
int main()
{
MYSQL* conn; // MySQL连接对象
MYSQL_RES* res; // MySQL查询结果对象
MYSQL_ROW row; // MySQL查询结果的一行
conn = mysql_init(NULL); // 初始化MySQL连接对象
if (conn == NULL)
{
cout << "Error: " << mysql_error(conn) << endl;
exit(1);
}
// 连接到MySQL服务器
if (mysql_real_connect(conn, "localhost", "username", "password", "database_name", 0, NULL, 0) == NULL)
{
cout << "Error: " << mysql_error(conn) << endl;
exit(1);
}
// 执行SQL查询语句
if (mysql_query(conn, "SELECT * FROM table_name"))
{
cout << "Error: " << mysql_error(conn) << endl;
exit(1);
}
res = mysql_use_result(conn); // 获取查询结果
// 输出查询结果
while ((row = mysql_fetch_row(res)) != NULL)
{
cout << row[0] << " " << row[1] << " " << row[2] << endl;
}
mysql_free_result(res); // 释放查询结果对象
mysql_close(conn); // 关闭MySQL连接对象
return 0;
}
```
在上面的代码中,您需要将“username”、“password”和“database_name”替换为您自己的用户名、密码和数据库名称。
相关推荐
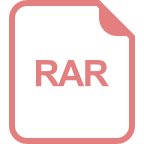
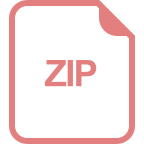
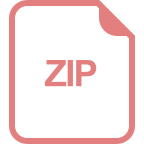
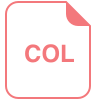













