从文件中读取之前保存的目录树结构,并根据其重新建立当前目录树相关C++代码
时间: 2024-05-03 12:16:20 浏览: 51
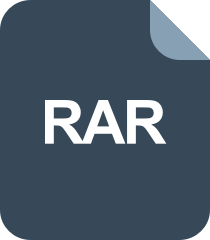
创建目录树并保存到磁盘文件,再读取该文件重建目录树

好的,我会尽力回答你的问题。
首先,从文件中读取之前保存的目录树结构,需要先确定保存目录树结构的文件格式。假设我们采用以下格式:
每一行表示一个目录或文件,以空格分隔,第一个单词表示类型(d表示目录,f表示文件),第二个单词表示名称,第三个单词表示父目录名称(根目录的父目录名称为/)。
例如,如下所示的目录树结构:
```
d root /
f file1 root
d dir1 root
f file2 dir1
```
将保存为以下文本文件:
```
d root /
f file1 root
d dir1 root
f file2 dir1
```
读取该文件的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 目录或文件结构体
struct node {
char type; // 类型,d表示目录,f表示文件
char name[256]; // 名称
char parent[256]; // 父目录名称
struct node *child; // 子节点指针
struct node *sibling; // 兄弟节点指针
};
// 根据名称查找节点
struct node *find_node(struct node *root, const char *name) {
if (root == NULL || name == NULL) {
return NULL;
}
if (strcmp(root->name, name) == 0) {
return root;
}
struct node *node = find_node(root->child, name);
if (node != NULL) {
return node;
}
node = find_node(root->sibling, name);
if (node != NULL) {
return node;
}
return NULL;
}
// 创建节点
struct node *create_node(char type, const char *name, const char *parent) {
struct node *node = (struct node *) malloc(sizeof(struct node));
node->type = type;
strcpy(node->name, name);
strcpy(node->parent, parent);
node->child = NULL;
node->sibling = NULL;
return node;
}
// 插入节点
void insert_node(struct node *parent, struct node *node) {
if (parent == NULL || node == NULL) {
return;
}
if (parent->child == NULL) {
parent->child = node;
} else {
struct node *sibling = parent->child;
while (sibling->sibling != NULL) {
sibling = sibling->sibling;
}
sibling->sibling = node;
}
}
// 从文件中读取目录树结构
struct node *read_tree(const char *filename) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
return NULL;
}
struct node *root = NULL;
char line[1024];
while (fgets(line, sizeof(line), file) != NULL) {
char type, name[256], parent[256];
sscanf(line, "%c %s %s", &type, name, parent);
struct node *node = create_node(type, name, parent);
if (root == NULL) {
root = node;
} else {
struct node *parent_node = find_node(root, parent);
if (parent_node != NULL) {
insert_node(parent_node, node);
} else {
free(node);
}
}
}
fclose(file);
return root;
}
```
然后,根据读取的目录树结构重新建立当前目录树相关的C代码,可以使用递归方式遍历目录树并生成相关的C代码。代码如下:
```c
// 生成目录或文件的C代码
void gen_code(struct node *node) {
if (node == NULL) {
return;
}
if (node->type == 'd') {
printf("mkdir(\"%s\", 0755);\n", node->name);
printf("chdir(\"%s\");\n", node->name);
} else {
printf("FILE *fp = fopen(\"%s\", \"w\");\n", node->name);
printf("fclose(fp);\n");
}
gen_code(node->child);
printf("chdir(\"..\");\n");
gen_code(node->sibling);
}
// 生成当前目录树的C代码
void gen_tree_code(struct node *root) {
if (root == NULL) {
return;
}
printf("#include <stdio.h>\n");
printf("#include <stdlib.h>\n");
printf("#include <sys/stat.h>\n");
printf("#include <unistd.h>\n");
printf("int main() {\n");
gen_code(root);
printf("return 0;\n");
printf("}\n");
}
```
调用方式如下:
```c
int main() {
struct node *root = read_tree("tree.txt");
gen_tree_code(root);
return 0;
}
```
这样就可以根据之前保存的目录树结构重新建立当前目录树相关的C代码了。
阅读全文
相关推荐
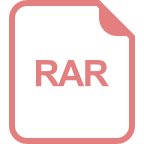
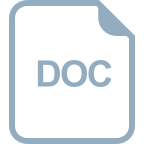
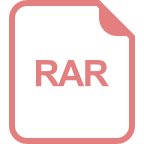
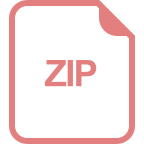
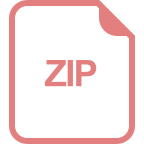
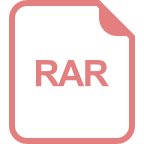
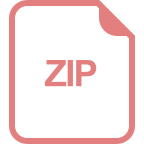
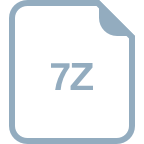
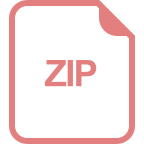
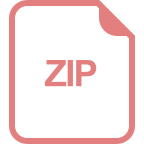
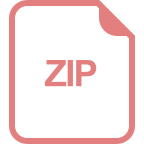
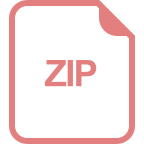
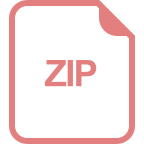
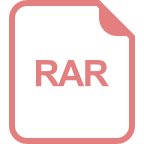
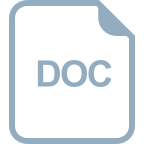
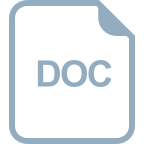