AttributeError: 'Tensor' object has no attribute 'astype'转为torch
时间: 2023-06-22 07:22:26 浏览: 723
在PyTorch中,可以使用`torch.Tensor`的`type()`方法来变换数据类型,而不是使用`astype()`方法。因此,如果您想将`Tensor`对象的数据类型转换为另一个数据类型,可以使用以下代码:
```
import torch
# 创建一个float类型的张量
x = torch.tensor([1.0, 2.0, 3.0])
# 将其转换为int类型
y = x.type(torch.IntTensor)
# 打印输出结果
print(y)
```
输出结果如下:
```
tensor([1, 2, 3], dtype=torch.int32)
```
在上面的代码中,我们首先创建了一个float类型的张量`x`,然后使用`type()`方法将其转换为int类型的张量`y`,最后打印输出结果。通过指定`torch.IntTensor`参数,我们可以将数据类型转换为int类型。
相关问题
AttributeError: 'Tensor' object has no attribute 'astype'
这个错误通常发生在尝试将一个Tensor类型的对象转换为另一个类型时。astype是一个NumPy数组的方法,但是Tensor没有该方法。
要解决这个问题,可以使用PyTorch中的to方法来转换Tensor类型。例如,要将一个FloatTensor转换为一个LongTensor,可以这样做:
```python
import torch
float_tensor = torch.FloatTensor([1.0, 2.0, 3.0])
long_tensor = float_tensor.to(torch.long)
```
在这个例子中,我们首先创建了一个FloatTensor对象,然后使用to方法将其转换为一个LongTensor对象。这个方法可以接受一个参数,即要转换的数据类型。
AttributeError: 'Tensor' object has no attribute 'astype'如何解决
`AttributeError: 'Tensor' object has no attribute 'astype'` 这个错误通常发生在尝试对一个张量(Tensor)对象应用 `astype()` 函数时,而这个函数并未被该对象支持。
张量对象通常来自于一些科学计算库如 NumPy、PyTorch 或 TensorFlow 等。在这类库中,`astype()` 通常是用于转换数据类型的操作,例如从整数型到浮点型等。然而,某些特定类型的张量并不支持这一操作。这可能是由于几个原因:
1. **错误导入**:确保你已经正确地导入了相关的库。例如,在使用 PyTorch 的时候应该导入 `torch` 库,而在使用 TensorFlow 时则需要导入 `tf` 或 `keras` 相关模块。
2. **张量类型限制**:不是所有张量都可以应用 `astype()` 函数。例如,在 PyTorch 中,张量类型(如 `torch.LongTensor`, `torch.FloatTensor` 等)之间可以相互转换,但如果试图将某个非张量类型的数据转换成张量类型,则会引发错误。
3. **使用不当**:有时,错误可能是由代码结构造成的。例如,如果在错误的上下文中尝试使用 `astype()`, 比如在一个不应该涉及到张量运算的地方。
### 解决步骤
#### 步骤一:确认环境设置
确保你正在使用的库已经被正确安装并且版本兼容。检查当前激活的环境中包含哪些关键库,并查看它们的版本是否适合你的项目需求。
#### 步骤二:验证张量实例
确保你调用 `astype()` 的对象确实是一个张量。你可以通过打印变量名并检查其类型(如使用 Python 的 `type()` 函数)来完成这一点。
```python
print(type(tensor_variable))
```
#### 步骤三:指定正确的张量类型
如果你的目标是将张量类型从一个数据类型转换到另一个类型,确保目标数据类型是在可用张量类型列表内。例如,若你想将一个整型张量转换为浮点型张量:
```python
new_tensor = tensor_variable.astype(torch.float)
```
对于 PyTorch 张量来说,应使用 `.to(dtype)` 方法:
```python
new_tensor = tensor_variable.to(torch.float)
```
#### 步骤四:处理异常情况
添加适当的错误处理机制,以防未来可能出现未知的问题,使得程序能够优雅地处理异常情况。
```python
try:
new_tensor = tensor_variable.astype(torch.float) # 如果在NumPy环境下
except Exception as e:
print("Error occurred:", str(e))
# 或者使用PyTorch的方式
try:
new_tensor = tensor_variable.to(torch.float)
except Exception as e:
print("Error occurred:", str(e))
```
#### 步骤五:审查代码逻辑
检查是否有其他部分的代码逻辑导致这种错误的发生。例如,确保在调用 `astype()` 之前,该变量已被初始化为张量形式,并且存在于预期的上下文中。
### 相关问题:
1. 如何识别我的数据类型是否适用于转换?
2. 对于不同库(如NumPy vs TensorFlow),转换操作有何差异?
3. 能否提供一个示例代码片段说明如何正确转换张量类型?
阅读全文
相关推荐
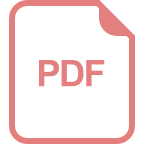
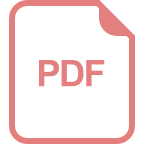






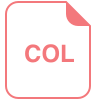






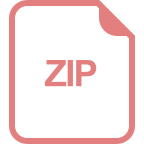