在MATLAB中随机生成障碍物、起点和终点,用A*算法得出路径
时间: 2023-07-15 15:11:35 浏览: 71
好的,下面是一个基本的实现过程:
1. 首先你需要创建一个地图,在地图中随机生成障碍物、起点和终点。可以使用MATLAB中的图形界面工具箱来创建地图。
2. 然后你需要实现A*算法。A*算法是一种启发式搜索算法,它可以找到从起点到终点的最短路径。该算法需要使用一个启发函数来评估每个节点的代价,以便确定下一步应该扩展哪个节点。在MATLAB中,你可以使用内置函数 `astarsearch` 来实现A*算法。
3. 在实现 A*算法之前,你需要先将地图转换成一个网格图。网格图是一个二维数组,其中每个元素代表一个网格,每个网格可以是一个空格或一个障碍物。在MATLAB中,你可以使用 `binaryOccupancyMap` 函数来创建网格图。
4. 接下来,你需要实现启发函数。启发函数是一个估计函数,它可以评估从当前节点到目标节点的代价。在A*算法中,启发函数通常使用欧几里得距离或曼哈顿距离来计算。在MATLAB中,你可以使用 `heuristic` 参数来指定启发函数。
5. 最后,你需要使用 `astarsearch` 函数来搜索最短路径。该函数需要指定起点和终点坐标,以及网格图和启发函数。它将返回一个包含路径点的二维数组。
这是一个简单的实现过程,你可以在此基础上进行修改和优化。
相关问题
在MATLAB中,在20×20的栅格图中随机设置起点、终点和障碍物,并用A*算法运算得出路径
好的,以下是一个示例代码,你可以根据需要进行修改:
```matlab
% 设置栅格图大小
n = 20;
m = 20;
% 随机设置起点、终点和障碍物
start = randi([1 n], 1, 2);
goal = randi([1 n], 1, 2);
obs_num = ceil(n * m / 5); % 随机生成的障碍物数量为栅格数的五分之一
obs = randi([1 n*m], 1, obs_num);
obs = unique(obs); % 确保障碍物不重复
obs = [mod(obs-1, n)+1, floor((obs-1)/n)+1]; % 将随机生成的障碍物转化为二维坐标
% 初始化栅格图
grid = zeros(n, m);
for i = 1:size(obs,1)
grid(obs(i,1), obs(i,2)) = 1; % 将障碍物标记为1
end
% 显示栅格图
figure(1)
clf
imagesc(grid);
hold on
plot(start(2), start(1), 'rx', 'MarkerSize', 15, 'LineWidth', 3)
plot(goal(2), goal(1), 'gx', 'MarkerSize', 15, 'LineWidth', 3)
axis equal
axis tight
% 计算启发函数
H = zeros(n, m);
for i = 1:n
for j = 1:m
H(i,j) = sqrt((i-goal(1))^2 + (j-goal(2))^2);
end
end
% A*算法
openSet = [start, 0, H(start(1),start(2))];
closedSet = [];
while ~isempty(openSet)
[~, idx] = min(openSet(:,3));
current = openSet(idx,:);
if isequal(current(1:2), goal)
% 找到路径
path = [current(1:2)];
while ~isequal(path(end,:), start)
for i = 1:size(closedSet,1)
if isequal(closedSet(i,1:2), path(end,:))
path = [path; closedSet(i,4:5)];
break
end
end
end
path = flip(path);
% 显示路径
plot(path(:,2), path(:,1), 'm', 'LineWidth', 3);
break
end
openSet(idx,:) = [];
closedSet = [closedSet; current];
neighbors = [current(1)-1, current(2); current(1)+1, current(2); ...
current(1), current(2)-1; current(1), current(2)+1];
for i = 1:size(neighbors,1)
if any(neighbors(i,:) < 1) || any(neighbors(i,:) > n) || ...
grid(neighbors(i,1), neighbors(i,2)) == 1
continue
end
temp_g = current(3) + 1;
temp_h = H(neighbors(i,1),neighbors(i,2));
temp_f = temp_g + temp_h;
if any(ismember(neighbors(i,:), closedSet(:,1:2), 'rows'))
continue
end
idx_open = find(ismember(openSet(:,1:2), neighbors(i,:), 'rows'));
if isempty(idx_open)
openSet = [openSet; neighbors(i,:), temp_g, temp_h, temp_f];
elseif temp_f < openSet(idx_open,6)
openSet(idx_open,3:6) = [temp_g, temp_h, temp_f];
end
end
end
```
这段代码会生成一个随机的栅格图,并用 A* 算法计算出起点到终点的最短路径,并将路径显示在图中。你可以运行这段代码,看看效果如何。
生成 Matlab 的 A* 算法进行避障路径规划的示例代码
### 回答1:
以下是一个使用A*算法进行避障路径规划的MATLAB示例代码:
```matlab
% 地图宽度和高度
width = 10;
height = 10;
% 创建障碍物地图,1表示障碍物,0表示可通过
map = zeros(width, height);
map(3:7, 4) = 1;
map(5, 3:8) = 1;
map(9, 1:5) = 1;
% 起点和终点
start = [1, 1];
goal = [10, 10];
% 生成A*算法对象
astar = robotics.AStar;
% 设置A*算法对象的地图和起点终点
astar.Map = robotics.BinaryOccupancyGrid(map, 1);
astar.Start = start;
astar.End = goal;
% 进行路径规划
path = findpath(astar);
% 显示地图和路径
figure;
show(astar.Map);
hold on;
plot(path(:,1), path(:,2), 'r', 'LineWidth', 2);
title('A*算法路径规划');
```
在上述代码中,首先定义了一个地图,包含了一些障碍物。然后定义了起点和终点,并生成了一个A*算法对象。接着将地图和起点终点传入A*算法对象中,进行路径规划。最后在地图上显示路径。
### 回答2:
下面是一个使用A*算法进行避障路径规划的示例代码:
```matlab
% A*算法避障路径规划示例代码
% 地图尺寸
mapSize = [10, 10];
% 起点和终点坐标
startPos = [1, 1];
goalPos = [10, 10];
% 障碍物坐标
obstacles = [3, 4; 4, 4; 5, 4; 6, 4; 7, 4; 8, 4; 9, 4];
% 创建地图
map = zeros(mapSize);
% 标记障碍物
for i = 1:size(obstacles, 1)
obstaclePos = obstacles(i, :);
map(obstaclePos(1), obstaclePos(2)) = 1;
end
% 定义节点类
classdef Node
properties
position
parent
gCost
hCost
fCost
end
methods
function obj = Node(position, parent, gCost, hCost)
obj.position = position;
obj.parent = parent;
obj.gCost = gCost;
obj.hCost = hCost;
obj.fCost = gCost + hCost;
end
end
end
% A*算法
function path = AStar(startPos, goalPos, map)
% 生成起点和终点节点
startNode = Node(startPos, [], 0, heuristic(startPos, goalPos));
goalNode = Node(goalPos, [], 0, 0);
% 初始化开启列表和闭合列表
openList = [startNode];
closedList = [];
% 开始遍历
while ~isempty(openList)
% 选取开启列表中最小fCost的节点
currentNode = openList(1);
currentIndex = 1;
for i = 1:length(openList)
if openList(i).fCost < currentNode.fCost
currentNode = openList(i);
currentIndex = i;
end
end
% 将当前节点从开启列表移至闭合列表
openList(currentIndex) = [];
closedList = [closedList, currentNode];
% 到达终点
if isequal(currentNode.position, goalNode.position)
path = [];
node = currentNode;
while ~isempty(node.parent)
path = [node.position; path];
node = node.parent;
end
return;
end
% 寻找当前节点相邻的可行节点
adjacentNodes = [];
for dx = -1:1
for dy = -1:1
if dx ~= 0 || dy ~= 0
neighborPos = currentNode.position + [dx, dy];
if all(neighborPos >= 1) && all(neighborPos <= mapSize) && ~map(neighborPos(1), neighborPos(2))
adjacentNodes = [adjacentNodes, Node(neighborPos, currentNode, currentNode.gCost + 1, heuristic(neighborPos, goalPos))];
end
end
end
end
% 更新相邻节点的gCost、hCost和fCost
for i = 1:length(adjacentNodes)
node = adjacentNodes(i);
% 如果节点已在闭合列表中,则跳过
if any(isequal(node.position, closedList.position))
continue;
end
% 计算新的gCost和fCost
newGCost = currentNode.gCost + 1;
if ~any(isequal(node.position, openList.position)) || newGCost < node.gCost
node.gCost = newGCost;
node.fCost = node.gCost + node.hCost;
node.parent = currentNode;
if ~any(isequal(node.position, openList.position))
openList = [openList, node];
end
end
end
end
% 无法找到路径
path = [];
end
% 启发式函数(使用曼哈顿距离)
function h = heuristic(pos, goalPos)
h = abs(pos(1) - goalPos(1)) + abs(pos(2) - goalPos(2));
end
% 运行A*算法
path = AStar(startPos, goalPos, map);
% 绘制地图和路径
figure;
image(map' + 1);
colormap([1, 1, 1; 0, 0, 0]);
hold on;
plot(startPos(2), startPos(1), 'go', 'MarkerSize', 10);
plot(goalPos(2), goalPos(1), 'ro', 'MarkerSize', 10);
if ~isempty(path)
plot(path(:, 2), path(:, 1), 'b', 'LineWidth', 2);
end
hold off;
```
此示例代码使用A*算法实现了一个简单的避障路径规划。首先定义了节点类,然后实现了A*算法函数。使用曼哈顿距离作为启发式函数来估计节点到目标节点的距离。最后,绘制了地图和路径,绿色点表示起点,红色点表示终点,蓝色线表示找到的路径。
### 回答3:
A*算法是一种常用于寻找最短路径的算法,可以应用于避障路径规划。下面是一个使用MATLAB实现A*算法进行路径规划的示例代码:
```matlab
% 定义地图和启发函数
map = [0, 0, 1, 0, 0, 0;
0, 0, 1, 0, 0, 0;
0, 0, 0, 0, 1, 0;
0, 0, 1, 1, 1, 0;
0, 0, 0, 0, 1, 0;
0, 0, 0, 0, 0, 0];
heuristic = @(pos, goal) norm(pos - goal);
% 定义起点和终点
start = [1, 1];
goal = [6, 6];
% 初始化开放列表和关闭列表
openList = [];
closeList = [];
% 设置起点的代价g和启发函数值f
start.g = 0;
start.f = start.g + heuristic(start, goal);
% 将起点加入开放列表
openList = [openList, start];
% 开始A*算法
while ~isempty(openList)
% 寻找开放列表中f值最小的节点
[~, index] = min([openList.f]);
currentNode = openList(index);
% 如果当前节点为终点,路径搜索结束
if currentNode(1) == goal(1) && currentNode(2) == goal(2)
break;
end
% 将当前节点加入关闭列表
closeList = [closeList, currentNode];
% 从开放列表中移除当前节点
openList(index) = [];
% 遍历当前节点的相邻节点
for i = -1:1
for j = -1:1
% 忽略对角线方向
if i == 0 && j == 0 || i ~= 0 && j ~= 0
continue;
end
% 计算相邻节点的坐标
neighbor = [currentNode(1) + i, currentNode(2) + j];
% 如果相邻节点在地图范围内且未被访问过
if neighbor(1) >= 1 && neighbor(1) <= size(map, 1) && ...
neighbor(2) >= 1 && neighbor(2) <= size(map, 2) && ...
~any([closeList.x] == neighbor(1) & [closeList.y] == neighbor(2)) && ...
map(neighbor(1), neighbor(2)) == 0
% 计算相邻节点的代价g和启发函数值f
neighbor.g = currentNode.g + 1;
neighbor.f = neighbor.g + heuristic(neighbor, goal);
% 如果相邻节点已经在开放列表中,更新其代价和启发函数值
index = find([openList.x] == neighbor(1) & [openList.y] == neighbor(2));
if ~isempty(index) && neighbor.g < openList(index).g
openList(index).g = neighbor.g;
openList(index).f = neighbor.f;
end
% 如果相邻节点不在开放列表中,将其添加进去
if isempty(index)
openList = [openList, neighbor];
end
end
end
end
end
% 从终点开始回溯路径
path = [];
currentNode = goal;
while ~isempty(currentNode.parent)
path = [currentNode; path];
currentNode = currentNode.parent;
end
path = [start; path];
% 可视化路径
figure;
imagesc(map);
colormap(flipud(gray));
hold on;
plot(path(:, 2), path(:, 1), 'r', 'LineWidth', 2);
axis equal;
```
相关推荐
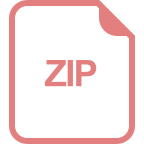












