spring boot中使用aop如何配置
时间: 2023-08-06 19:03:57 浏览: 126
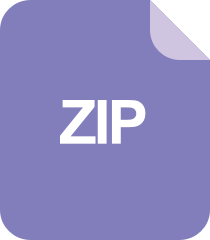
spring的aop配置
在 Spring Boot 中使用 AOP,可以通过以下步骤来配置:
1. 在 Maven 或 Gradle 构建文件中,添加 `spring-boot-starter-aop` 依赖项,以引入 Spring Boot AOP 模块。
2. 定义一个切面类,使用 `@Aspect` 注解标注该类,同时在该类中定义一个或多个通知方法,通知方法使用 `@Before`、`@After`、`@Around` 等注解来标注。
3. 在 Spring Boot 应用程序类上添加 `@EnableAspectJAutoProxy` 注解,以启用 AspectJ 自动代理。
4. (可选)如果需要使用基于注解的 AOP 配置,可以在 Spring Boot 应用程序类上添加 `@ComponentScan` 注解,以扫描切面类和被切入的类。
5. 在 Spring Boot 配置文件(如 `application.properties` 或 `application.yml`)中,可以配置 AOP 的相关属性,例如日志级别、切入点表达式等。
下面是一个简单的示例:
```
// 定义切面类
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.demo.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("method " + joinPoint.getSignature().getName() + " is called");
}
}
// 启用 AspectJ 自动代理
@EnableAspectJAutoProxy
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
在上面的示例中,定义了一个切面类 `LoggingAspect`,它包含一个 `@Before` 通知方法。在 `DemoApplication` 类上添加了 `@EnableAspectJAutoProxy` 注解,以启用 AspectJ 自动代理。在 `LoggingAspect` 类上添加了 `@Component` 注解,以使它能够被 Spring 扫描到并注册为一个切面类。在 `@Before` 注解中定义了一个切入点表达式,表示该通知方法将在 `com.example.demo.service` 包中的任何方法执行前调用。
阅读全文
相关推荐
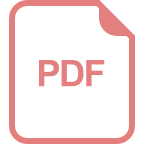
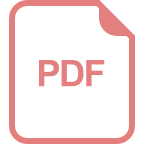
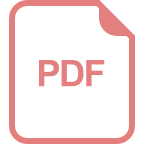
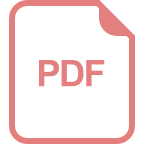
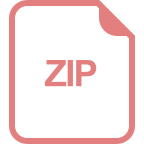
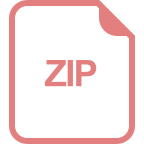
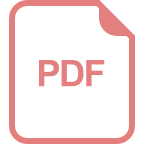
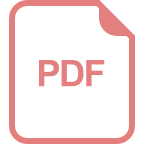
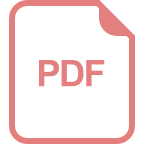
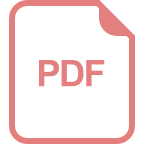
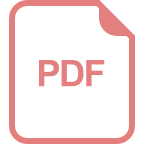
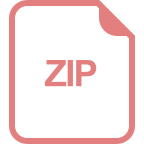
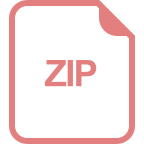
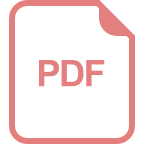
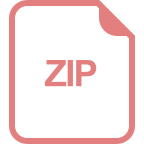